


Add, delete, modify and query operations of XML data in Python
Add, delete, modify and query operations of XML data in Python
XML (Extensible Markup Language) is a text format used to store and transmit data. In Python, we can use a variety of libraries to process XML data, the most commonly used of which is the xml.etree.ElementTree
library. This article will introduce how to use Python to add, delete, modify and query XML data, and illustrate it through code examples.
1. Introduction of modules
First, we need to introduce the xml.etree.ElementTree library to process XML data. You can use the following code to introduce it:
import xml.etree.ElementTree as ET
2. Parse XML files
Before operating on XML data, we first need to parse the XML file into an Element object. You can use the following code to parse XML files:
tree = ET.parse('data.xml') root = tree.getroot()
The above code will parse the data.xml file into an ElementTree object tree, and obtain the root element object root from the tree.
3. Search for elements
In XML, there can be hierarchical relationships between elements. We can find a specific element by its tag name and attributes. You can use the following code to find elements:
-
Find elements by tag name:
element = root.find('element_name')
-
Find elements by attributes:
element = root.find(".//element_name[@attribute='value']")
4. Traverse elements
In XML data, there may be multiple elements with the same tag name. We can get all these elements by traversing. You can use the following code to traverse elements:
for element in root.iter('element_name'): # 执行相关操作
The above code will traverse all child elements named element_name under the root element root and perform related operations on each element.
5. New elements
We can use the Element
method of the Element object to create a new element, and use the append
method to add it to the specified under the parent element. You can use the following code to add new elements:
new_element = ET.Element('new_element') parent_element.append(new_element)
The above code will create an element named new_element and add it to the parent element parent_element.
6. Modify the element
You can use the set
method of the Element object to modify the attribute value of the element. You can use the following code to modify the attribute value of an element:
element.set('attribute', 'new_value')
The above code will change the attribute value of the element to new_value.
7. Delete elements
You can use the remove
method of the Element object to delete the specified element. You can use the following code to delete elements:
parent_element.remove(element)
The above code will delete the specified element element from the parent_element parent element.
To sum up, we introduced how to use Python to add, delete, modify and query XML data and explained it through code examples. By mastering these basic operations, we can process XML data more conveniently and implement our own business logic.
The above is the detailed content of Add, delete, modify and query operations of XML data in Python. For more information, please follow other related articles on the PHP Chinese website!
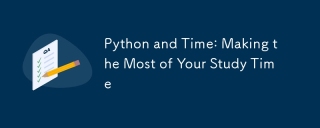
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
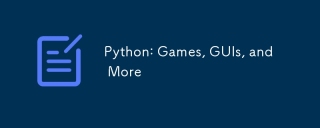
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
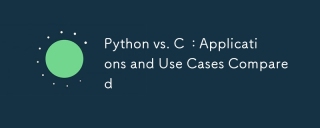
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
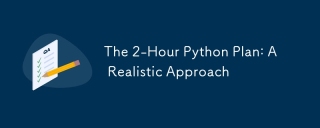
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
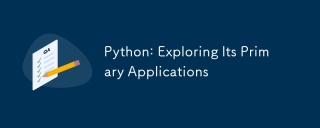
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
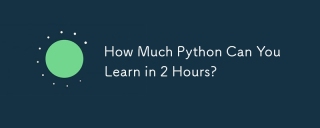
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
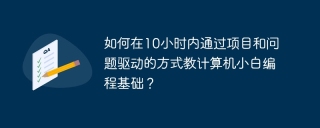
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
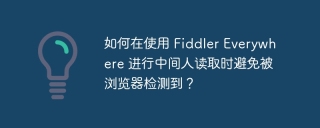
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
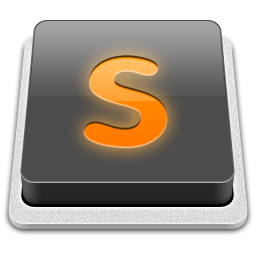
SublimeText3 Mac version
God-level code editing software (SublimeText3)
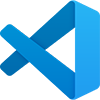
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft