


How to use Python to implement the user survey function of CMS system
How to use Python to implement the user survey function of the CMS system
Introduction:
Content management system (CMS) is a tool widely used in website construction. It can help website administrators conveniently Create, modify and manage website content. In many websites, user survey feedback is an important part, which can help website administrators understand user needs and improve website experience. This article will introduce how to use Python language to implement the user survey function in CMS system, and attach code examples.
- System Design
The user survey function mainly includes four main steps: creating questionnaires, displaying questionnaires, collecting user feedback and generating statistical reports. We will use Python's web framework Flask to build and develop the website. - Create a questionnaire
First, we need to create a questionnaire form to collect user feedback. Questionnaires can be created using HTML form elements, such as radio buttons, check boxes, text input boxes, etc. Use Flask's form extension package wtforms to process form data and validate input more conveniently. The following is a code example for a simple questionnaire form:
from flask_wtf import FlaskForm from wtforms import StringField, SubmitField, RadioField class SurveyForm(FlaskForm): name = StringField('姓名') gender = RadioField('性别', choices=[('男', '男'), ('女', '女')]) feedback = StringField('反馈') submit = SubmitField('提交')
- Display questionnaire
Displaying the questionnaire on the website can be achieved by using Flask's route decorator. We can create a routing function to pass the questionnaire form as a parameter to the template and display it in the template. The following is a simple code example showing the routing function of the questionnaire:
from flask import render_template @app.route('/survey', methods=['GET', 'POST']) def survey(): form = SurveyForm() if form.validate_on_submit(): # 处理用户提交的问卷数据 return '谢谢参与!' return render_template('survey.html', form=form)
- Collecting user feedback
For the questionnaire data submitted by the user, we can save it to the database for convenience Follow-up analysis and reporting. You can use Python's database operation modules such as SQLAlchemy or MongoDB to complete data storage. The following is a code example that uses SQLAlchemy to save user feedback data:
from flask_sqlalchemy import SQLAlchemy app = Flask(__name__) app.config['SQLALCHEMY_DATABASE_URI'] = 'mysql://username:password@localhost/mydatabase' db = SQLAlchemy(app) class Feedback(db.Model): id = db.Column(db.Integer, primary_key=True) name = db.Column(db.String(50)) gender = db.Column(db.String(10)) feedback = db.Column(db.String(100)) def save(self): db.session.add(self) db.session.commit()
In the routing function of questionnaire submission, we can save the user's feedback data to the database:
@app.route('/survey', methods=['GET', 'POST']) def survey(): # ... if form.validate_on_submit(): feedback = Feedback(name=form.name.data, gender=form.gender.data, feedback=form.feedback.data) feedback.save() return '谢谢参与!' # ...
- Generate statistical report
In order to better understand user needs and evaluations, we can perform statistics and analysis on the collected user feedback data and generate statistical reports. Python comes with many libraries for processing data and generating charts, such as pandas, matplotlib and seaborn. The following is a simple code example for generating statistical reports:
import pandas as pd import matplotlib.pyplot as plt import seaborn as sns # 从数据库中读取反馈数据 feedbacks = Feedback.query.all() # 将反馈数据转换为DataFrame df = pd.DataFrame([(f.name, f.gender, f.feedback) for f in feedbacks], columns=['姓名', '性别', '反馈']) # 统计性别比例 gender_counts = df['性别'].value_counts() plt.pie(gender_counts, labels=gender_counts.index, autopct='%1.1f%%') plt.title('参与用户性别比例') plt.show() # 分析反馈内容 word_counts = df['反馈'].str.lower().str.split().explode().value_counts().head(10) sns.barplot(x=word_counts.index, y=word_counts.values) plt.title('反馈内容常用词统计') plt.show()
Conclusion:
By using the Python language and corresponding libraries, we can easily implement the user survey function in the CMS system, and Better understand user needs and reviews through statistical analysis. This article shows an implementation method based on the Flask framework and attaches corresponding code examples. I hope it will be helpful to readers.
The above is the detailed content of How to use Python to implement the user survey function of CMS system. For more information, please follow other related articles on the PHP Chinese website!
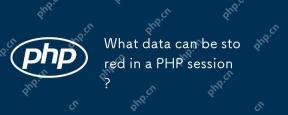
PHPsessionscanstorestrings,numbers,arrays,andobjects.1.Strings:textdatalikeusernames.2.Numbers:integersorfloatsforcounters.3.Arrays:listslikeshoppingcarts.4.Objects:complexstructuresthatareserialized.
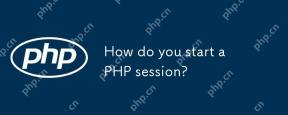
TostartaPHPsession,usesession_start()atthescript'sbeginning.1)Placeitbeforeanyoutputtosetthesessioncookie.2)Usesessionsforuserdatalikeloginstatusorshoppingcarts.3)RegeneratesessionIDstopreventfixationattacks.4)Considerusingadatabaseforsessionstoragei
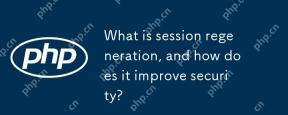
Session regeneration refers to generating a new session ID and invalidating the old ID when the user performs sensitive operations in case of session fixed attacks. The implementation steps include: 1. Detect sensitive operations, 2. Generate new session ID, 3. Destroy old session ID, 4. Update user-side session information.
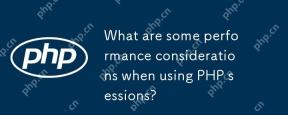
PHP sessions have a significant impact on application performance. Optimization methods include: 1. Use a database to store session data to improve response speed; 2. Reduce the use of session data and only store necessary information; 3. Use a non-blocking session processor to improve concurrency capabilities; 4. Adjust the session expiration time to balance user experience and server burden; 5. Use persistent sessions to reduce the number of data read and write times.
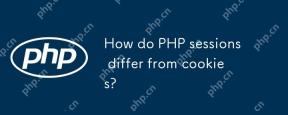
PHPsessionsareserver-side,whilecookiesareclient-side.1)Sessionsstoredataontheserver,aremoresecure,andhandlelargerdata.2)Cookiesstoredataontheclient,arelesssecure,andlimitedinsize.Usesessionsforsensitivedataandcookiesfornon-sensitive,client-sidedata.
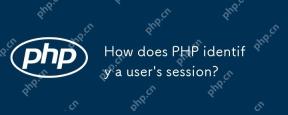
PHPidentifiesauser'ssessionusingsessioncookiesandsessionIDs.1)Whensession_start()iscalled,PHPgeneratesauniquesessionIDstoredinacookienamedPHPSESSIDontheuser'sbrowser.2)ThisIDallowsPHPtoretrievesessiondatafromtheserver.
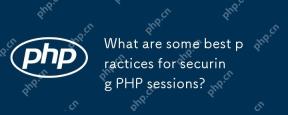
The security of PHP sessions can be achieved through the following measures: 1. Use session_regenerate_id() to regenerate the session ID when the user logs in or is an important operation. 2. Encrypt the transmission session ID through the HTTPS protocol. 3. Use session_save_path() to specify the secure directory to store session data and set permissions correctly.
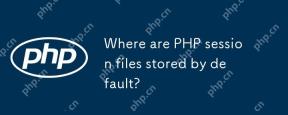
PHPsessionfilesarestoredinthedirectoryspecifiedbysession.save_path,typically/tmponUnix-likesystemsorC:\Windows\TemponWindows.Tocustomizethis:1)Usesession_save_path()tosetacustomdirectory,ensuringit'swritable;2)Verifythecustomdirectoryexistsandiswrita


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use
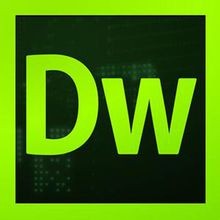
Dreamweaver CS6
Visual web development tools
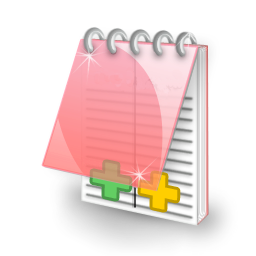
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
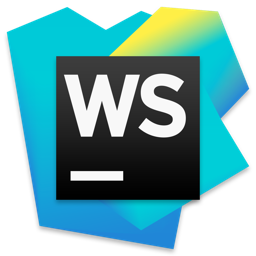
WebStorm Mac version
Useful JavaScript development tools
