


The new Stream API was introduced in Java 8, which provides a more efficient and concise way to process collection data. The Stream API provides various methods to process and transform data, among which the collect()
method is one of the most important and commonly used methods. This article describes how to use the collect()
method to collect a collection into a Map object, and provides corresponding code examples.
Before Java 8, if we wanted to convert a collection into a Map object, we needed to use cumbersome traversal and addition operations. In Java 8, this goal can be achieved more conveniently using the collect()
method of the Stream API. The
collect()
method is one of the termination operations of the Stream API. It receives a Collector
parameter to specify the collection method. When collecting as a Map object, we can use the Collectors.toMap()
method to collect.
The following is a sample code that uses the collect()
method to collect a collection into a Map object:
import java.util.*; import java.util.stream.Collectors; public class StreamCollectExample { public static void main(String[] args) { List<String> fruits = Arrays.asList("apple", "banana", "orange"); Map<String, Integer> fruitLengthMap = fruits.stream() .collect(Collectors.toMap( fruit -> fruit, // Key 映射函数 fruit -> fruit.length() // Value 映射函数 )); System.out.println(fruitLengthMap); } }
In the above code, we first create a collection containing three fruits Collect fruits
and convert it to a stream via the stream()
method. Then use the collect()
method and pass in the Collectors.toMap()
method as a parameter. This method receives two lambda expression parameters for specifying the mapping function of Key and Value.
In our example, the Key mapping function is fruit -> fruit
, which takes fruit as the Key; the Value mapping function is fruit -> fruit.length()
, that is, the length of the fruit is used as Value. Finally, the collect()
method processes the elements in the stream according to the specified mapping function and returns a Map object.
The output results are as follows:
{orange=6, banana=6, apple=5}
As you can see, we finally obtained a Map object containing the fruit and its length.
In addition to the basic collection function, the Collectors.toMap()
method also provides some other parameters. For example, we can specify what should be done when there are duplicate Keys, by passing in a merge function to resolve conflicts.
The following is a sample code with Key conflict handling:
import java.util.*; import java.util.stream.Collectors; public class StreamCollectExample { public static void main(String[] args) { List<String> fruits = Arrays.asList("apple", "banana", "orange", "apple"); Map<String, Integer> fruitLengthMap = fruits.stream() .collect(Collectors.toMap( fruit -> fruit, // Key 映射函数 fruit -> fruit.length(), // Value 映射函数 (length1, length2) -> length1 // Key 冲突处理函数 )); System.out.println(fruitLengthMap); } }
In the above code, we enter the third parameter position of the toMap()
method A merge function (length1, length2) -> length1
is created. This function will choose to keep the first Key when encountering duplicate Keys, and ignore subsequent Keys.
The output results are as follows:
{orange=6, banana=6, apple=5}
It can be seen that when a Key conflict occurs, only the first Key that appears is retained, and other Keys are ignored.
By using the collect()
method of the Stream API, we can very conveniently collect the collection as a Map object, and we can also customize the mapping function of Key and Value and the way to handle conflicts. . In this way, we can process collection data more flexibly and improve the readability and efficiency of the code.
The above is an introduction and sample code for using the collect()
method to collect collections into Map objects in Java 8. I hope this article can help you understand the use of Stream API.
The above is the detailed content of Stream API in Java 8: How to collect collection as Map object using collect() method. For more information, please follow other related articles on the PHP Chinese website!
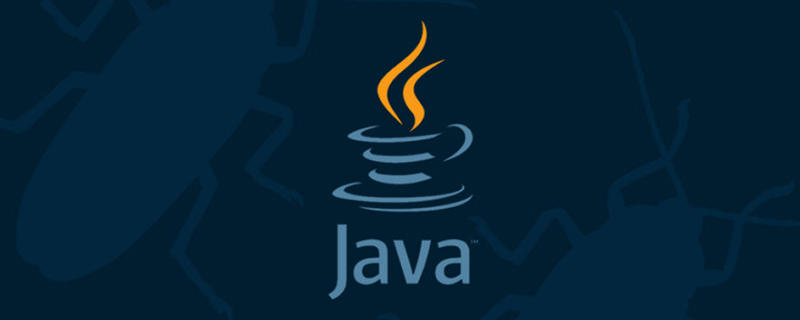
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
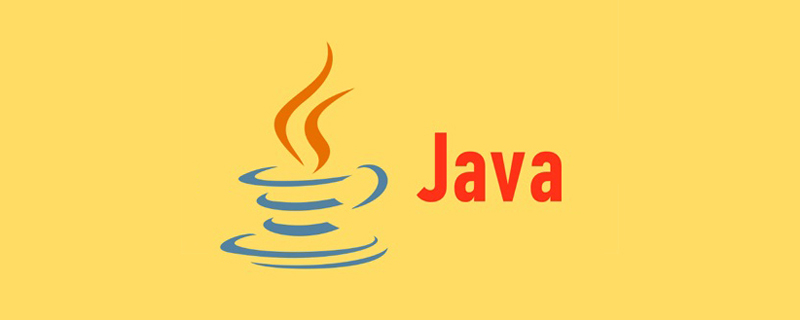
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
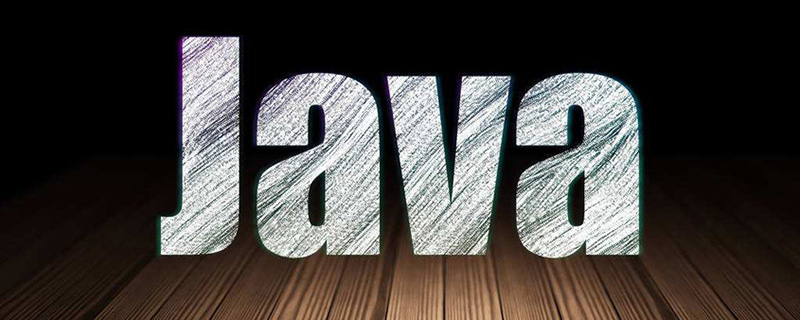
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
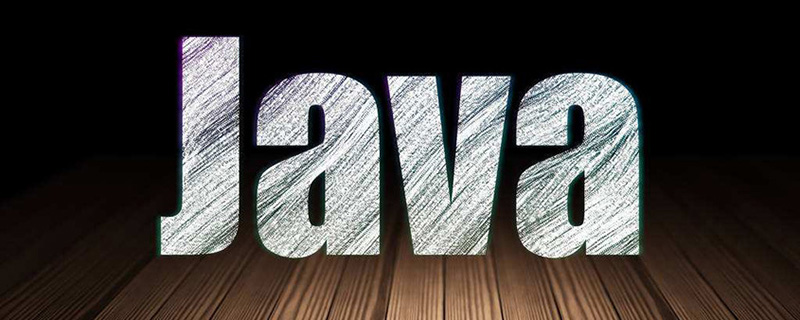
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
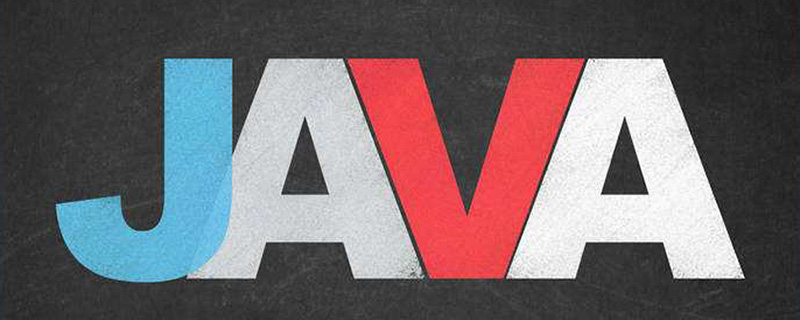
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
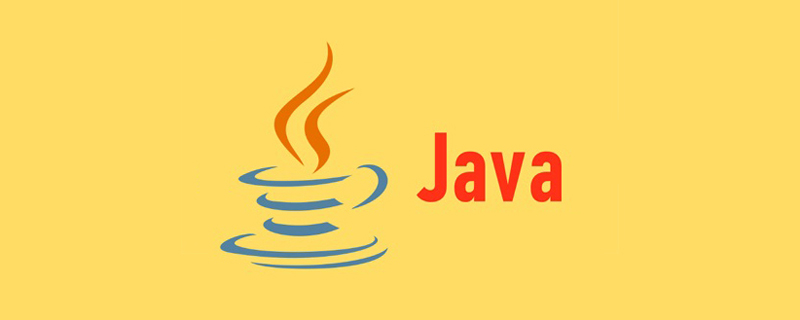
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
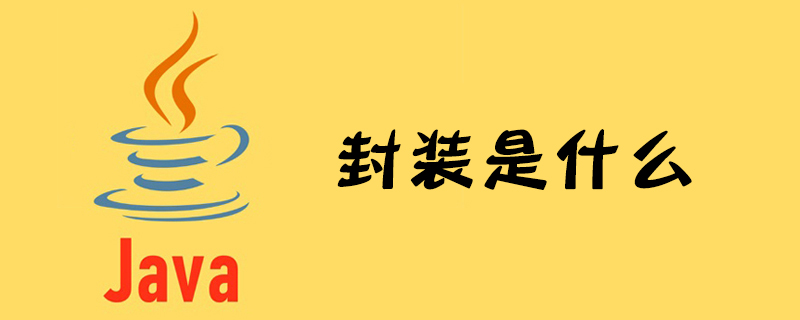
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
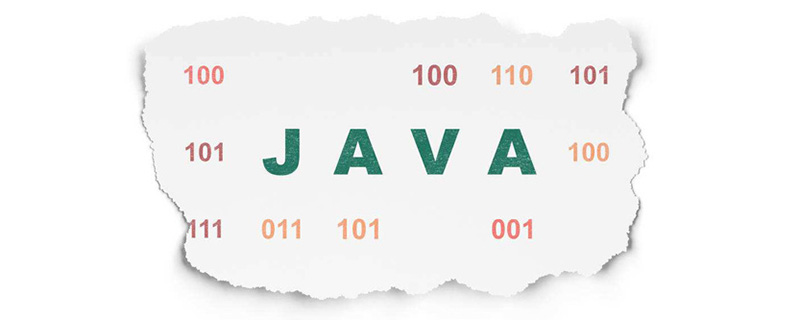
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于设计模式的相关问题,主要将装饰器模式的相关内容,指在不改变现有对象结构的情况下,动态地给该对象增加一些职责的模式,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
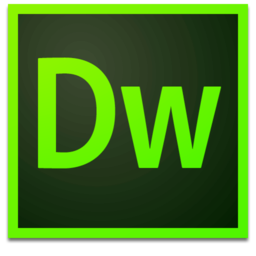
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!
