How to implement distributed task queue using Redis and Node.js
How to use Redis and Node.js to implement distributed task queues
Distributed systems are an important concept in modern software development. In distributed systems, task queues are a commonly used component used to coordinate and manage concurrent tasks on multiple nodes. Redis is an open source, high-performance in-memory database, and Node.js is a lightweight event-driven JavaScript runtime. This article will introduce how to use Redis and Node.js to implement a distributed task queue, and provide corresponding code examples.
- Installing and configuring Redis
First, you need to install and configure Redis on a local or remote server. You can download the installation package from the Redis official website and install and configure it according to the official documentation. After completing the installation and configuration, you can perform Redis interactive operations through the redis-cli command line interface.
- Create task queue
To create a task queue using Node.js, you can use the following code:
const redis = require('redis'); class TaskQueue { constructor(queueName) { this.queueName = queueName; this.client = redis.createClient(); } enqueue(task) { this.client.rpush(this.queueName, JSON.stringify(task)); } dequeue(callback) { this.client.lpop(this.queueName, (err, task) => { if (task) { callback(JSON.parse(task)); } }); } } module.exports = TaskQueue;
In the above code, first import the redis module , and then create a TaskQueue class. The constructor receives a queue name as a parameter and creates a Redis client object. The enqueue method adds the task to the queue, and uses the rpush command to store the task in the form of a JSON string in the Redis list. The dequeue method removes the tasks from the queue, pops the first task in the task list through the lpop command and returns it to the callback function.
- Create task processor
To create a task processor, you can use the following code:
class Worker { constructor(queueName, processTask) { this.queue = new TaskQueue(queueName); this.processTask = processTask; } start() { setInterval(() => { this.queue.dequeue(task => { this.processTask(task); }); }, 1000); } } module.exports = Worker;
In the above code, a Worker class is created, The constructor receives a queue name and a function to handle the task as parameters. The start method uses the setInterval function to regularly remove tasks from the queue and pass the tasks to the processing function.
- Using task queue
Using task queue, you can write a simple sample program:
const TaskQueue = require('./taskQueue'); const Worker = require('./worker'); const taskQueue = new TaskQueue('myQueue'); const worker = new Worker('myQueue', task => { console.log(`Processing task: ${task.name}`); }); worker.start(); taskQueue.enqueue({ name: 'Task1' }); taskQueue.enqueue({ name: 'Task2' }); taskQueue.enqueue({ name: 'Task3' });
In the above code, first import the TaskQueue and Worker modules , and then create a task queue and a task processor. Add three tasks to the task queue and start the task processor. The task processor periodically removes tasks from the task queue and processes them.
- Run the sample program
Before running the sample program, you need to ensure that the Redis server has been started. Execute the following command on the command line:
node example.js
The sample program will output the following content:
Processing task: Task1 Processing task: Task2 Processing task: Task3
Description The sample program successfully retrieved three tasks from the task queue and processed them in order.
This article introduces how to use Redis and Node.js to implement distributed task queues. By using the list data structure of Redis and the event-driven mechanism of Node.js, an efficient distributed task queue can be easily implemented. The code sample provides a simple example that can serve as a starting point for beginners. I believe that by reading this article, you have a deeper understanding of the implementation of distributed task queues and can use it in actual development projects.
The above is the detailed content of How to implement distributed task queue using Redis and Node.js. For more information, please follow other related articles on the PHP Chinese website!
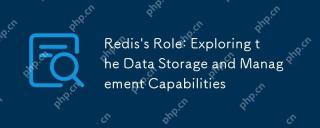
Redis plays a key role in data storage and management, and has become the core of modern applications through its multiple data structures and persistence mechanisms. 1) Redis supports data structures such as strings, lists, collections, ordered collections and hash tables, and is suitable for cache and complex business logic. 2) Through two persistence methods, RDB and AOF, Redis ensures reliable storage and rapid recovery of data.
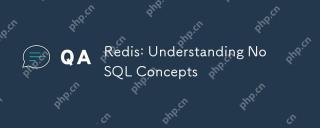
Redis is a NoSQL database suitable for efficient storage and access of large-scale data. 1.Redis is an open source memory data structure storage system that supports multiple data structures. 2. It provides extremely fast read and write speeds, suitable for caching, session management, etc. 3.Redis supports persistence and ensures data security through RDB and AOF. 4. Usage examples include basic key-value pair operations and advanced collection deduplication functions. 5. Common errors include connection problems, data type mismatch and memory overflow, so you need to pay attention to debugging. 6. Performance optimization suggestions include selecting the appropriate data structure and setting up memory elimination strategies.
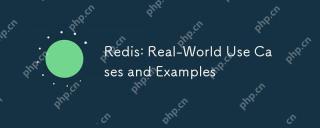
The applications of Redis in the real world include: 1. As a cache system, accelerate database query, 2. To store the session data of web applications, 3. To implement real-time rankings, 4. To simplify message delivery as a message queue. Redis's versatility and high performance make it shine in these scenarios.
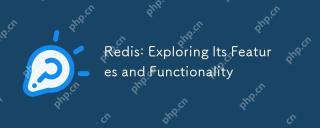
Redis stands out because of its high speed, versatility and rich data structure. 1) Redis supports data structures such as strings, lists, collections, hashs and ordered collections. 2) It stores data through memory and supports RDB and AOF persistence. 3) Starting from Redis 6.0, multi-threaded I/O operations have been introduced, which has improved performance in high concurrency scenarios.
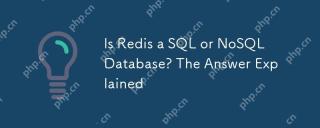
RedisisclassifiedasaNoSQLdatabasebecauseitusesakey-valuedatamodelinsteadofthetraditionalrelationaldatabasemodel.Itoffersspeedandflexibility,makingitidealforreal-timeapplicationsandcaching,butitmaynotbesuitableforscenariosrequiringstrictdataintegrityo
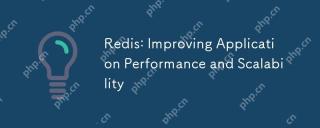
Redis improves application performance and scalability by caching data, implementing distributed locking and data persistence. 1) Cache data: Use Redis to cache frequently accessed data to improve data access speed. 2) Distributed lock: Use Redis to implement distributed locks to ensure the security of operation in a distributed environment. 3) Data persistence: Ensure data security through RDB and AOF mechanisms to prevent data loss.
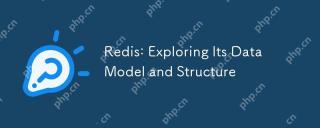
Redis's data model and structure include five main types: 1. String: used to store text or binary data, and supports atomic operations. 2. List: Ordered elements collection, suitable for queues and stacks. 3. Set: Unordered unique elements set, supporting set operation. 4. Ordered Set (SortedSet): A unique set of elements with scores, suitable for rankings. 5. Hash table (Hash): a collection of key-value pairs, suitable for storing objects.
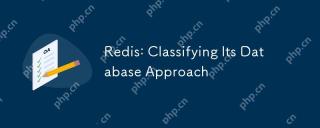
Redis's database methods include in-memory databases and key-value storage. 1) Redis stores data in memory, and reads and writes fast. 2) It uses key-value pairs to store data, supports complex data structures such as lists, collections, hash tables and ordered collections, suitable for caches and NoSQL databases.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
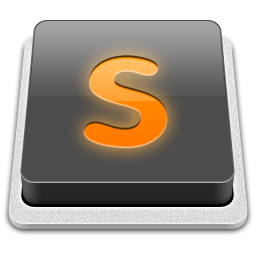
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor