


How to use the concurrent function in Go language to crawl multiple web pages in parallel?
How to use the concurrent function in Go language to achieve parallel crawling of multiple web pages?
In modern web development, it is often necessary to crawl data from multiple web pages. The general approach is to initiate network requests one by one and wait for responses, which is less efficient. The Go language provides powerful concurrency functions that can improve efficiency by crawling multiple web pages in parallel. This article will introduce how to use the concurrent function of Go language to achieve parallel crawling of multiple web pages, as well as some precautions.
First, we need to create concurrent tasks using the go
keyword built into the Go language. By adding the go
keyword before the function call, the Go language will wrap the function call into a concurrent task, and then immediately return control to the main program to continue executing subsequent code. This can achieve the effect of crawling multiple web pages in parallel.
The following is a simple sample code:
package main import ( "fmt" "io/ioutil" "net/http" ) // 并发抓取网页的函数 func fetch(url string, ch chan<- string) { resp, err := http.Get(url) if err != nil { ch <- fmt.Sprintf("fetch %s failed: %v", url, err) return } defer resp.Body.Close() body, err := ioutil.ReadAll(resp.Body) if err != nil { ch <- fmt.Sprintf("read %s failed: %v", url, err) return } ch <- fmt.Sprintf("fetch %s success: %d bytes", url, len(body)) } func main() { urls := []string{"http://www.example.com", "http://www.google.com", "http://www.microsoft.com"} ch := make(chan string) for _, url := range urls { go fetch(url, ch) } for range urls { fmt.Println(<-ch) } }
In the above code, we define a fetch
function to crawl a single web page. The fetch
function initiates a network request through http.Get
and sends the request result to a chan
type channel ch
. In the main program, we create a channel ch
and a slice urls
containing multiple web page URLs. Then, loop through the urls
slices by for
and call the fetch
function on each URL. Each time the fetch
function is called, a concurrent task will be created using the go
keyword so that multiple tasks can be executed at the same time.
Finally, we traverse the urls
slice once through the for
loop, receive the crawl results from the channel ch
and print the output. Because the read operation of the channel will block, the program will wait for all concurrent tasks to complete before outputting.
It should be noted that the execution order of concurrent tasks is uncertain, so the order of the final output results is also uncertain. If you need to maintain the order of results, you can use sync.WaitGroup
to wait for the completion of concurrent tasks and then process the results in order.
In addition, it should be noted that concurrently crawling web pages may cause greater pressure on the target website. In order to avoid being blocked by the target website or affecting service quality, you can reasonably adjust the number of concurrent tasks, increase the crawl interval and other strategies.
In short, by using the concurrency function of Go language, we can easily achieve parallel crawling of multiple web pages. This can not only improve the crawling efficiency, but also better cope with large-scale data collection needs. At the same time, using concurrent tasks can also improve the scalability and parallel computing capabilities of the program.
The above is the detailed content of How to use the concurrent function in Go language to crawl multiple web pages in parallel?. For more information, please follow other related articles on the PHP Chinese website!
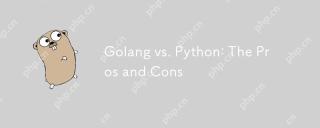
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
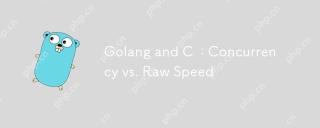
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
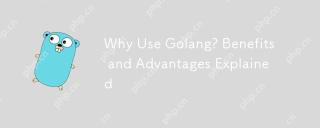
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
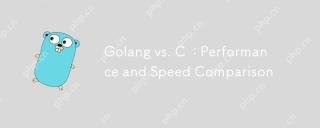
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
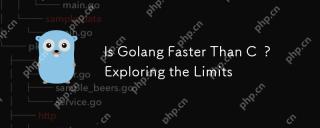
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
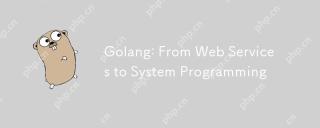
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
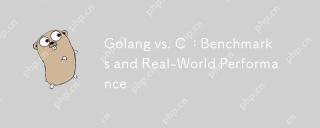
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
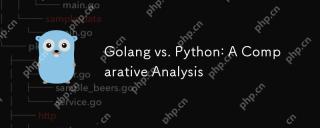
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
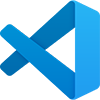
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
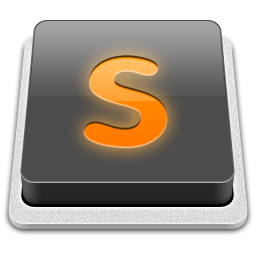
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
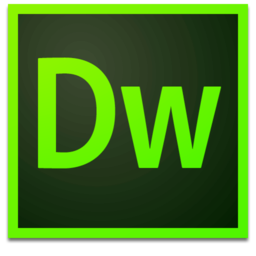
Dreamweaver Mac version
Visual web development tools