


PHP mall development skills: Design user registration and login process
When building a PHP mall website, the user registration and login process is a key part of it. A good user registration and login process can improve user experience and enhance the security and usability of the website. This article will introduce some techniques for designing user registration and login processes in PHP mall development, and provide code examples.
- Database design
First, we need to design the user table of the database. The user table should contain at least the following fields: user ID (user_id), username (username), password (password), email address (email), mobile phone number (phone), registration time (register_time), etc. When designing the database table, we can use the self-increasing user ID as the primary key and automatically assign the user ID when the user registers.
The following is a simplified user table design example:
CREATE TABLE users ( user_id INT(10) UNSIGNED AUTO_INCREMENT PRIMARY KEY, username VARCHAR(255) NOT NULL, password VARCHAR(255) NOT NULL, email VARCHAR(255) NOT NULL, phone VARCHAR(20) NOT NULL, register_time TIMESTAMP DEFAULT CURRENT_TIMESTAMP );
- User registration process
User registration is a common operation in a mall website. The following is A basic user registration process:
- The user fills out the registration form and enters necessary information such as user name, password, email address, and mobile phone number.
- The backend performs data verification to ensure that the entered information is in the correct format and checks whether the user name and email address have been registered.
- Insert the information submitted by the user into the user table in the database to complete user registration.
The following is a simple PHP code example for user registration:
<?php if ($_SERVER["REQUEST_METHOD"] == "POST") { $username = $_POST["username"]; $password = $_POST["password"]; $email = $_POST["email"]; $phone = $_POST["phone"]; // 数据验证 // 略... // 检查用户名和邮箱是否已经存在 // 略... // 插入用户数据到数据库中 $query = "INSERT INTO users (username, password, email, phone) VALUES ('$username', '$password', '$email', '$phone')"; $result = mysqli_query($conn, $query); if ($result) { // 注册成功 echo "注册成功!"; } else { // 注册失败 echo "注册失败!"; } } ?>
- User login process
User login is another step in the mall website An important operation, the following is a basic user login process:
- The user enters the username and password.
- The backend verifies whether the username and password entered by the user match the records in the database.
- If the match is successful, the user identification (such as user ID) is saved in the Session, indicating that the user has logged in successfully.
The following is a simple user login PHP code example:
<?php if ($_SERVER["REQUEST_METHOD"] == "POST") { $username = $_POST["username"]; $password = $_POST["password"]; // 后端验证用户名和密码是否匹配 $query = "SELECT user_id FROM users WHERE username='$username' AND password='$password'"; $result = mysqli_query($conn, $query); if (mysqli_num_rows($result) == 1) { // 登录成功 session_start(); $_SESSION["user_id"] = mysqli_fetch_assoc($result)["user_id"]; echo "登录成功!"; } else { // 登录失败 echo "用户名或密码错误!"; } } ?>
The above is a simple user registration and login process design and code example. In actual mall development, more security and user experience issues need to be considered, such as password encryption, verification code verification, etc. I hope this article will be helpful to PHP mall development.
The above is the detailed content of PHP mall development skills: Design user registration and login process. For more information, please follow other related articles on the PHP Chinese website!
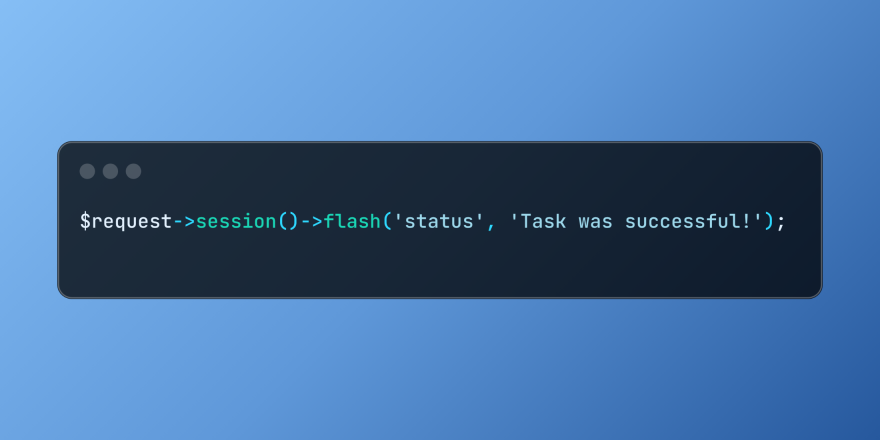
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
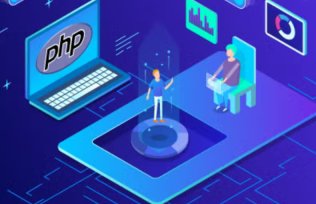
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
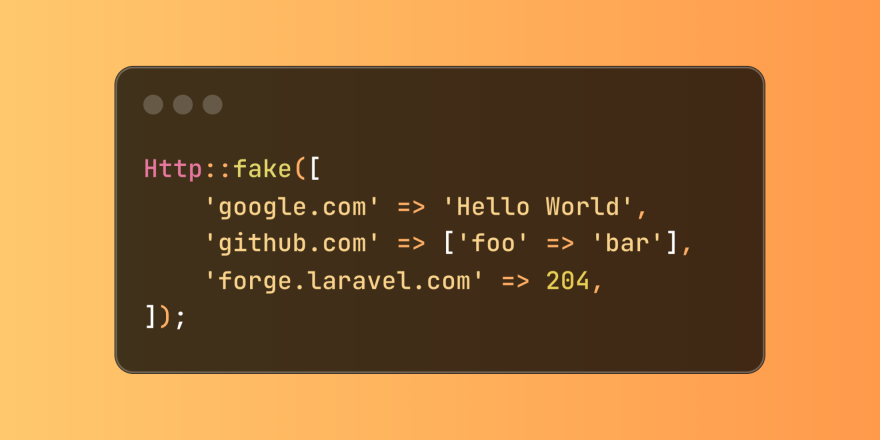
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
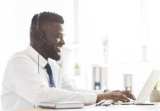
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
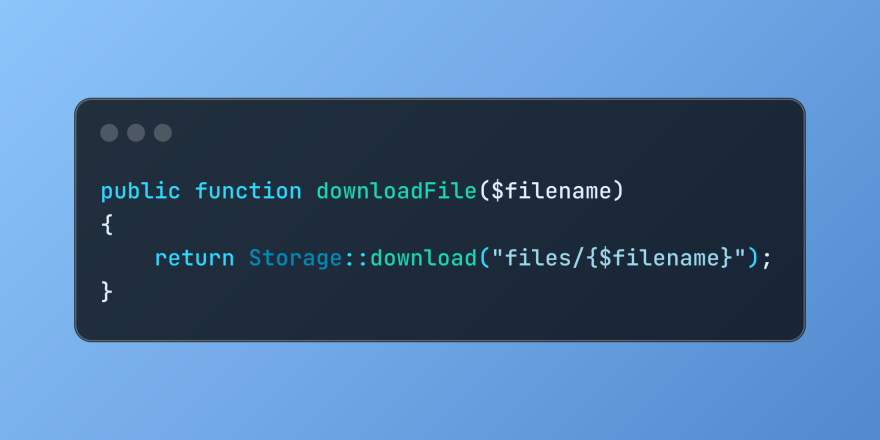
The Storage::download method of the Laravel framework provides a concise API for safely handling file downloads while managing abstractions of file storage. Here is an example of using Storage::download() in the example controller:
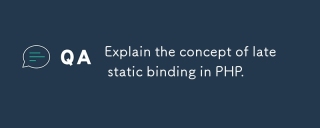
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
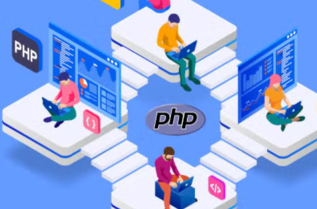
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
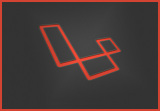
Laravel's service container and service providers are fundamental to its architecture. This article explores service containers, details service provider creation, registration, and demonstrates practical usage with examples. We'll begin with an ove


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
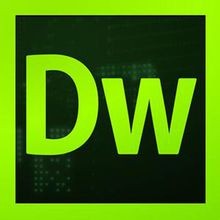
Dreamweaver CS6
Visual web development tools
