How to use the flask module for web development in Python 3.x
How to use the Flask module for Web development in Python 3.x
Introduction:
With the rapid development of the Internet, the demand for Web development is also increasing. To meet the needs of developers, many web development frameworks have emerged. Among them, Flask is a simple and practical web development framework. It is lightweight, flexible, and easy to expand. It is the first choice for many beginners and small and medium-sized projects.
This article will introduce you to how to use the Flask module in Python 3.x for web development, and provide some practical code examples.
Part One: Install Flask
Before we begin, first we need to install the Flask module. Flask can be installed from the command line using the following command:
pip install flask
Part 2: Create a simple Flask application
Next, we will create a simple Flask application. In this example, we will create a basic "Hello World" web page.
First, create a file named app.py
in the code editor and enter the following code:
# 导入 Flask 模块 from flask import Flask # 创建一个 Flask 应用实例 app = Flask(__name__) # 创建一个路由,处理根目录访问 @app.route('/') def hello_world(): return 'Hello, world!' # 运行应用 if __name__ == '__main__': app.run()
This code is very simple, it first imports The Flask
module was installed, and then a Flask
instance app
was created. Next, use the decorator @app.route('/')
to create a route that handles requests to access the root directory and returns a string "Hello, world!". Finally, the application is run via app.run()
.
After saving the code, execute the following command on the command line to run the application:
python app.py
If everything goes well, you will see output similar to the following:
* Running on http://127.0.0.1:5000/ (Press CTRL+C to quit)
At this time You can enter http://127.0.0.1:5000/
in your browser and you will see the "Hello, world!" string.
Part 3: Routing and View Functions
In the above example, we created a simple route, processed the access request to the root directory, and returned a string. Now we'll cover more about routing and view functions.
Flask supports using different URL rules to define routes. Routes can be defined using the decorator @app.route
. Here is an example:
# 创建一个路由,处理 /hello 路径的 GET 请求 @app.route('/hello') def hello(): return 'Hello, Flask!'
In this example, @app.route('/hello')
defines a route that will handle GET requests accessing the /hello path, and Returns the string "Hello, Flask!".
View functions are functions that process requests and return responses. In the above example, hello()
is a view function.
Part 4: Requests and Responses
In web development, requests and responses are very important concepts. Flask provides multiple ways to handle requests and responses.
Through the request
object, you can access request-related information, such as path, parameters, form data, etc. Here is an example:
from flask import request # 创建一个路由,处理 /search 路径的 GET 请求 @app.route('/search') def search(): keyword = request.args.get('keyword', '') # 获取查询参数 keyword return 'You are searching for: ' + keyword
In this example, we use the request.args.get()
method to get the value of the query parameter keyword
and return a string.
To return a response, you can use the return
statement or the make_response()
function. Here is an example:
from flask import make_response @app.route('/cookie') def cookie(): response = make_response('This is a cookie page.') response.set_cookie('username', 'john') # 设置一个名为 username 的 cookie return response
In this example, we create a response object using the make_response()
function and set it using the response.set_cookie()
method A cookie named username
is created.
Part 5: Template Engine
In actual Web development, we usually need to dynamically generate HTML pages. In order to easily implement this function, Flask provides a template engine.
When using a template engine, we can separate HTML code and dynamic content, making the code easier to maintain and develop. Here is an example using a template engine:
from flask import render_template @app.route('/user/<username>') def profile(username): return render_template('profile.html', name=username)
In this example, we use the render_template()
function to render the template profile.html
and pass the parameters ## The value of #username is passed to the template. In templates, you can use the
{{ name }} syntax to output dynamic content.
This article introduces how to use the Flask module for web development in Python 3.x and provides some practical code examples. I hope readers can understand the basic usage of Flask through this article, and can further learn and explore more functions of the Flask framework. I wish you all success in web development!
The above is the detailed content of How to use the flask module for web development in Python 3.x. For more information, please follow other related articles on the PHP Chinese website!
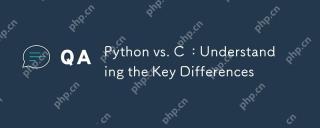
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
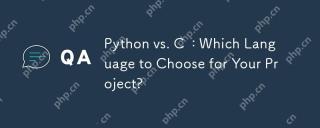
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
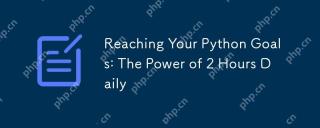
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.
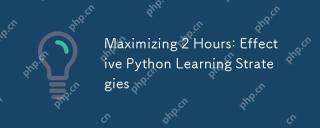
Methods to learn Python efficiently within two hours include: 1. Review the basic knowledge and ensure that you are familiar with Python installation and basic syntax; 2. Understand the core concepts of Python, such as variables, lists, functions, etc.; 3. Master basic and advanced usage by using examples; 4. Learn common errors and debugging techniques; 5. Apply performance optimization and best practices, such as using list comprehensions and following the PEP8 style guide.

Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
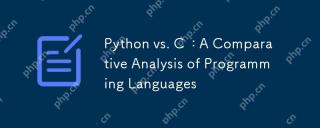
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
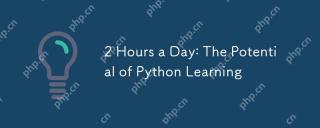
It is feasible to invest two hours a day to learn Python. 1. Learn new knowledge: Learn new concepts in one hour, such as lists and dictionaries. 2. Practice and exercises: Use one hour to perform programming exercises, such as writing small programs. Through reasonable planning and perseverance, you can master the core concepts of Python in a short time.
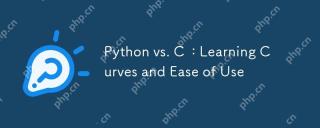
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
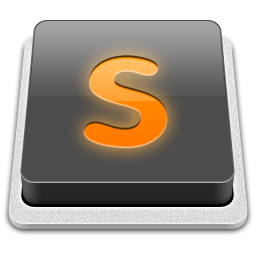
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Atom editor mac version download
The most popular open source editor
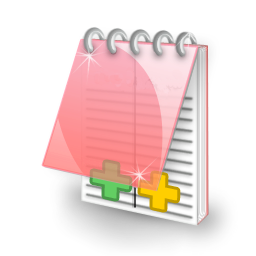
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.