PHP and SQLite: How to do data compression and encryption
PHP and SQLite: How to perform data compression and encryption
In many web applications, data security and storage space utilization are very important considerations. PHP and SQLite are two very widely used tools, and this article will introduce how to use them for data compression and encryption.
SQLite is a lightweight embedded database engine that does not have an independent server process but interacts directly with applications. PHP is a popular server-side scripting language that is widely used to build dynamic web applications.
First, we will discuss how to use PHP for data compression. Although SQLite itself does not support data compression, we can use PHP's compression library to compress SQLite database files.
The following is a sample code that demonstrates how to use PHP's compression library to compress a SQLite database:
<?php // 打开一个SQLite数据库连接 $db = new PDO('sqlite:mydatabase.sqlite'); // 执行数据压缩 $result = $db->exec('VACUUM'); if ($result !== false) { echo '数据库压缩成功!'; } else { echo '数据库压缩失败!'; } // 关闭数据库连接 $db = null; ?>
In the above code, we first open a SQLite database connection through the PDO class. Then, we use the exec() method to execute the VACUUM command, which will reorganize the database to reduce space usage. Finally, we close the database connection.
Next, let’s discuss how to encrypt a SQLite database. SQLite itself does not support data encryption, but we can use PHP's encryption library to encrypt SQLite database files.
The following is a sample code that demonstrates how to use PHP's encryption library to encrypt a SQLite database:
<?php // 打开一个SQLite数据库连接 $db = new PDO('sqlite:mydatabase.sqlite'); // 加密数据库 $result = $db->exec('ATTACH DATABASE 'encrypted.sqlite' AS encrypted KEY 'mypassword''); if ($result !== false) { echo '数据库加密成功!'; } else { echo '数据库加密失败!'; } // 关闭数据库连接 $db = null; ?>
In the above code, we first open a SQLite database connection through the PDO class. Then, we use the exec() method to execute the ATTACH DATABASE command and specify an encrypted database file name and password. This will create a new encrypted database file. Finally, we close the database connection.
To decrypt the encrypted database, we can open the encrypted database file in the same way and detach it from the original database using the DETACH DATABASE command.
To sum up, we can use PHP's compression library and encryption library to compress and encrypt data in the SQLite database. This improves data security and saves storage space. Of course, based on actual application requirements, we should use data compression and encryption functions with caution to avoid affecting performance and availability.
Reference:
- PHP Manual: PDO::__construct - www.php.net/manual/en/pdo.construct.php
- SQLite Documentation: Vacuum - www.sqlite.org/lang_vacuum.html
- SQLite Documentation: Attach Database - www.sqlite.org/lang_attach.html
The above is the detailed content of PHP and SQLite: How to do data compression and encryption. For more information, please follow other related articles on the PHP Chinese website!
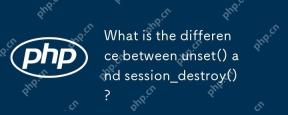
Thedifferencebetweenunset()andsession_destroy()isthatunset()clearsspecificsessionvariableswhilekeepingthesessionactive,whereassession_destroy()terminatestheentiresession.1)Useunset()toremovespecificsessionvariableswithoutaffectingthesession'soveralls
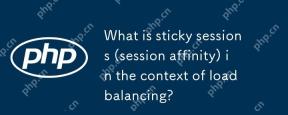
Stickysessionsensureuserrequestsareroutedtothesameserverforsessiondataconsistency.1)SessionIdentificationassignsuserstoserversusingcookiesorURLmodifications.2)ConsistentRoutingdirectssubsequentrequeststothesameserver.3)LoadBalancingdistributesnewuser
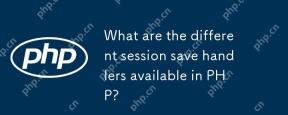
PHPoffersvarioussessionsavehandlers:1)Files:Default,simplebutmaybottleneckonhigh-trafficsites.2)Memcached:High-performance,idealforspeed-criticalapplications.3)Redis:SimilartoMemcached,withaddedpersistence.4)Databases:Offerscontrol,usefulforintegrati
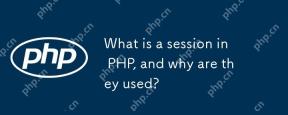
Session in PHP is a mechanism for saving user data on the server side to maintain state between multiple requests. Specifically, 1) the session is started by the session_start() function, and data is stored and read through the $_SESSION super global array; 2) the session data is stored in the server's temporary files by default, but can be optimized through database or memory storage; 3) the session can be used to realize user login status tracking and shopping cart management functions; 4) Pay attention to the secure transmission and performance optimization of the session to ensure the security and efficiency of the application.
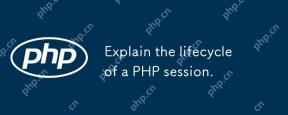
PHPsessionsstartwithsession_start(),whichgeneratesauniqueIDandcreatesaserverfile;theypersistacrossrequestsandcanbemanuallyendedwithsession_destroy().1)Sessionsbeginwhensession_start()iscalled,creatingauniqueIDandserverfile.2)Theycontinueasdataisloade
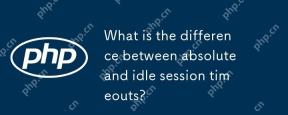
Absolute session timeout starts at the time of session creation, while an idle session timeout starts at the time of user's no operation. Absolute session timeout is suitable for scenarios where strict control of the session life cycle is required, such as financial applications; idle session timeout is suitable for applications that want users to keep their session active for a long time, such as social media.
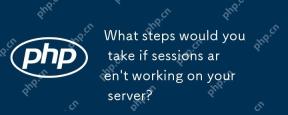
The server session failure can be solved through the following steps: 1. Check the server configuration to ensure that the session is set correctly. 2. Verify client cookies, confirm that the browser supports it and send it correctly. 3. Check session storage services, such as Redis, to ensure that they are running normally. 4. Review the application code to ensure the correct session logic. Through these steps, conversation problems can be effectively diagnosed and repaired and user experience can be improved.
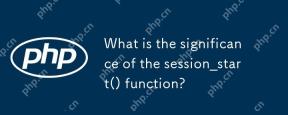
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
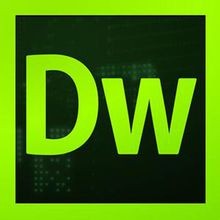
Dreamweaver CS6
Visual web development tools
