How to use PDO preprocessing to prevent SQL injection attacks
How to use PDO preprocessing to prevent SQL injection attacks
Introduction:
In the process of web development, we often need to interact with the database. However, incorrect database query operations may lead to serious security risks, and one of the most widely exploited attack methods is SQL injection attacks. In order to prevent SQL injection attacks, PDO provides a preprocessing mechanism. This article will introduce how to use PDO preprocessing correctly.
What is a SQL injection attack:
SQL injection is an attack technique against the database. The attacker inserts malicious code into the user input to cause the database to execute unverified query statements, thereby obtaining or Modify database data. For example, if a common login function does not handle user input correctly, an attacker can bypass login verification and directly access the database by entering a string of malicious SQL statements.
Principle of using PDO preprocessing to prevent SQL injection attacks:
PDO (PHP Data Object) is a database access abstraction layer provided by PHP. It uses a preprocessing mechanism to effectively prevent SQL injection attacks. Preprocessing refers to executing a query in two steps: first, setting parameters for the query's placeholders (for example: ?), and then executing the query. This mechanism ensures that user input is not directly executed as part of the SQL statement, thereby avoiding SQL injection attacks.
How to use PDO preprocessing to prevent SQL injection attacks:
The following will demonstrate how to use PDO preprocessing to prevent SQL injection attacks through a practical example.
First, we need to establish a connection to the database and set the relevant configuration of the database. The following is an example using a MySQL database:
$servername = "localhost"; $username = "root"; $password = "password"; $dbname = "myDB"; try { $conn = new PDO("mysql:host=$servername;dbname=$dbname", $username, $password); $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); echo "Connected successfully"; } catch(PDOException $e) { echo "Connection failed: " . $e->getMessage(); }
Next, we take the login function as an example to introduce how to use PDO preprocessing to prevent SQL injection attacks.
// 获取用户输入的用户名和密码 $username = $_POST['username']; $password = $_POST['password']; try { // 使用预处理查询用户信息 $stmt = $conn->prepare("SELECT * FROM users WHERE username = :username AND password = :password"); $stmt->bindParam(':username', $username); $stmt->bindParam(':password', $password); // 执行查询 $stmt->execute(); // 获取查询结果 $result = $stmt->fetch(PDO::FETCH_ASSOC); // 验证用户名和密码是否匹配 if ($result) { echo "登录成功"; } else { echo "用户名或密码错误"; } } catch(PDOException $e) { echo "查询失败: " . $e->getMessage(); }
In the above code, we use PDO's prepare
method to create a prepared statement and bind the parameters using the bindParam
method. This way, the values entered by the user are treated as parameters rather than directly as part of the SQL query. Finally, use the execute
method to execute the query, and use the fetch
method to obtain the query results.
Summary:
Using the PDO preprocessing mechanism is an effective way to prevent SQL injection attacks. By using user-entered values as parameters instead of splicing them directly into SQL queries, you can avoid the injection of malicious code. When writing database query code, be sure to use PDO preprocessing to ensure the security of the application.
The above is the detailed content of How to use PDO preprocessing to prevent SQL injection attacks. For more information, please follow other related articles on the PHP Chinese website!
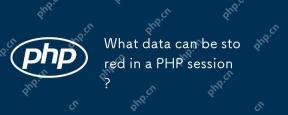
PHPsessionscanstorestrings,numbers,arrays,andobjects.1.Strings:textdatalikeusernames.2.Numbers:integersorfloatsforcounters.3.Arrays:listslikeshoppingcarts.4.Objects:complexstructuresthatareserialized.
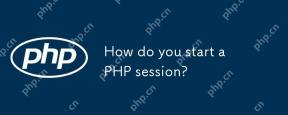
TostartaPHPsession,usesession_start()atthescript'sbeginning.1)Placeitbeforeanyoutputtosetthesessioncookie.2)Usesessionsforuserdatalikeloginstatusorshoppingcarts.3)RegeneratesessionIDstopreventfixationattacks.4)Considerusingadatabaseforsessionstoragei
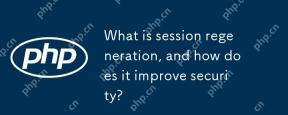
Session regeneration refers to generating a new session ID and invalidating the old ID when the user performs sensitive operations in case of session fixed attacks. The implementation steps include: 1. Detect sensitive operations, 2. Generate new session ID, 3. Destroy old session ID, 4. Update user-side session information.
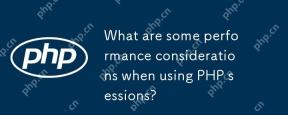
PHP sessions have a significant impact on application performance. Optimization methods include: 1. Use a database to store session data to improve response speed; 2. Reduce the use of session data and only store necessary information; 3. Use a non-blocking session processor to improve concurrency capabilities; 4. Adjust the session expiration time to balance user experience and server burden; 5. Use persistent sessions to reduce the number of data read and write times.
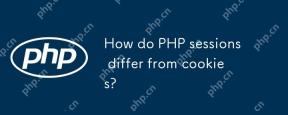
PHPsessionsareserver-side,whilecookiesareclient-side.1)Sessionsstoredataontheserver,aremoresecure,andhandlelargerdata.2)Cookiesstoredataontheclient,arelesssecure,andlimitedinsize.Usesessionsforsensitivedataandcookiesfornon-sensitive,client-sidedata.
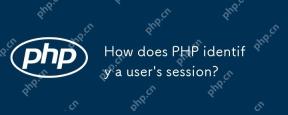
PHPidentifiesauser'ssessionusingsessioncookiesandsessionIDs.1)Whensession_start()iscalled,PHPgeneratesauniquesessionIDstoredinacookienamedPHPSESSIDontheuser'sbrowser.2)ThisIDallowsPHPtoretrievesessiondatafromtheserver.
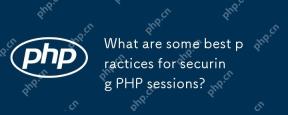
The security of PHP sessions can be achieved through the following measures: 1. Use session_regenerate_id() to regenerate the session ID when the user logs in or is an important operation. 2. Encrypt the transmission session ID through the HTTPS protocol. 3. Use session_save_path() to specify the secure directory to store session data and set permissions correctly.
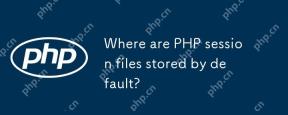
PHPsessionfilesarestoredinthedirectoryspecifiedbysession.save_path,typically/tmponUnix-likesystemsorC:\Windows\TemponWindows.Tocustomizethis:1)Usesession_save_path()tosetacustomdirectory,ensuringit'swritable;2)Verifythecustomdirectoryexistsandiswrita


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
