


Zend Framework middleware: Adds OAuth and OpenID login support to applications
Zend Framework Middleware: Add OAuth and OpenID login support to applications
User authentication is a critical feature in today's Internet applications. In order to provide better user experience and security, many applications choose to integrate third-party login services, such as OAuth and OpenID. In Zend Framework, we can easily add OAuth and OpenID login support to applications through middleware.
First, we need to install the OAuth and OpenID modules of Zend Framework. They can be installed through Composer:
composer require zendframework/zend-oauth composer require zendframework/zend-openid
After completing the installation, we can start writing middleware to handle user authentication.
First, we create a middleware class named AuthMiddleware:
use PsrHttpMessageRequestInterface; use PsrHttpMessageResponseInterface; use ZendDiactorosResponseRedirectResponse; use ZendStratigilityMiddlewareInterface; use ZendAuthenticationAuthenticationService; class AuthMiddleware implements MiddlewareInterface { private $authService; public function __construct(AuthenticationService $authService) { $this->authService = $authService; } public function __invoke(RequestInterface $request, ResponseInterface $response, callable $next = null) : ResponseInterface { // 检查用户是否已认证 if ($this->authService->hasIdentity()) { // 用户已认证,继续请求处理 return $next($request, $response); } // 用户未认证,重定向到登录页面 return new RedirectResponse('/login'); } }
In this middleware class, we use the AuthenticationService component of Zend Framework to check whether the user has been authenticated. If the user has been authenticated, we continue request processing; otherwise, jump to the login page.
Next step, we create a middleware class called LoginMiddleware to handle user login logic:
use PsrHttpMessageRequestInterface; use PsrHttpMessageResponseInterface; use ZendDiactorosResponseHtmlResponse; use ZendStratigilityMiddlewareInterface; use ZendAuthenticationAuthenticationService; use ZendAuthenticationAdapterOpenId as OpenIdAdapter; class LoginMiddleware implements MiddlewareInterface { private $authService; public function __construct(AuthenticationService $authService) { $this->authService = $authService; } public function __invoke(RequestInterface $request, ResponseInterface $response, callable $next = null) : ResponseInterface { if ($request->getMethod() === 'POST') { // 处理登录表单提交 $identity = $request->getParsedBody()['identity']; $credential = $request->getParsedBody()['credential']; // 使用OpenID适配器进行认证 $adapter = new OpenIdAdapter(); $adapter->setIdentity($identity); $adapter->setCredential($credential); // 进行认证 $result = $this->authService->authenticate($adapter); if ($result->isValid()) { // 认证成功,存储用户身份信息 $this->authService->getStorage()->write($result->getIdentity()); // 记录用户登录成功的日志 // ... // 重定向到首页 return new RedirectResponse('/'); } // 认证失败,返回登录页面并显示错误信息 return new HtmlResponse($this->renderLoginForm(['error' => '用户名或密码错误'])); } // 显示登录页面 return new HtmlResponse($this->renderLoginForm()); } private function renderLoginForm(array $params = []) : string { // 渲染登录表单模板,可使用Twig等模板引擎 // ... } }
In this middleware class, we use Zend Framework’s OpenIdAdapter. User Authentication. After successful authentication, we store the user identity information and can perform some additional operations, such as recording a log of successful user login.
Finally, we add these middlewares to the Zend Framework application:
use ZendStratigilityMiddlewarePipe; use ZendAuthenticationAuthenticationService; use ZendDiactorosServerRequestFactory; // 创建Zend Framework应用程序实例 $app = new MiddlewarePipe(); // 创建AuthenticationService实例 $authService = new AuthenticationService(); // 添加OAuth和OpenID登录中间件 $app->pipe(new AuthMiddleware($authService)); $app->pipe(new LoginMiddleware($authService)); // 处理请求 $response = $app(ServerRequestFactory::fromGlobals(), new Response()); // 发送响应 $responseEmitter = new ResponseSapiEmitter(); $responseEmitter->emit($response);
In the above code, we create a MiddlewarePipe instance and add the AuthMiddleware and LoginMiddleware middleware. We then use Zend Framework's ServerRequestFactory to create a request instance and run the application by processing the request and sending a response.
Through the above steps, we successfully added OAuth and OpenID login support to the application. Users can now use third-party login services to authenticate and gain better user experience and security.
The above example is just a simple demonstration, and there may be more customization and integration operations in actual use. However, with the flexibility and ease of use of Zend Framework middleware, we can easily accomplish these operations and add various features to the application.
Middleware is one of the powerful features in Zend Framework, which provides a concise and extensible way to handle HTTP requests and responses. Whether it is authentication, authorization, logging or other functions, middleware can help us handle it quickly and flexibly. If your application requires user authentication, try using middleware to add OAuth and OpenID login support!
The above is the detailed content of Zend Framework middleware: Adds OAuth and OpenID login support to applications. For more information, please follow other related articles on the PHP Chinese website!
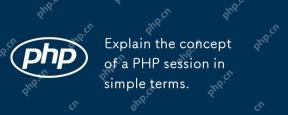
PHPsessionstrackuserdataacrossmultiplepagerequestsusingauniqueIDstoredinacookie.Here'showtomanagethemeffectively:1)Startasessionwithsession_start()andstoredatain$_SESSION.2)RegeneratethesessionIDafterloginwithsession_regenerate_id(true)topreventsessi
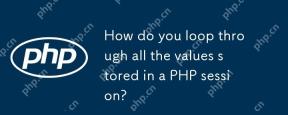
In PHP, iterating through session data can be achieved through the following steps: 1. Start the session using session_start(). 2. Iterate through foreach loop through all key-value pairs in the $_SESSION array. 3. When processing complex data structures, use is_array() or is_object() functions and use print_r() to output detailed information. 4. When optimizing traversal, paging can be used to avoid processing large amounts of data at one time. This will help you manage and use PHP session data more efficiently in your actual project.
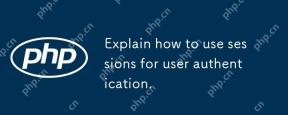
The session realizes user authentication through the server-side state management mechanism. 1) Session creation and generation of unique IDs, 2) IDs are passed through cookies, 3) Server stores and accesses session data through IDs, 4) User authentication and status management are realized, improving application security and user experience.
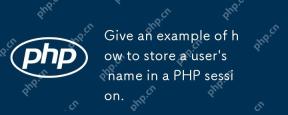
Tostoreauser'snameinaPHPsession,startthesessionwithsession_start(),thenassignthenameto$_SESSION['username'].1)Usesession_start()toinitializethesession.2)Assigntheuser'snameto$_SESSION['username'].Thisallowsyoutoaccessthenameacrossmultiplepages,enhanc
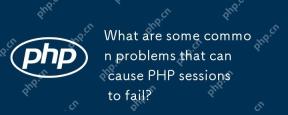
Reasons for PHPSession failure include configuration errors, cookie issues, and session expiration. 1. Configuration error: Check and set the correct session.save_path. 2.Cookie problem: Make sure the cookie is set correctly. 3.Session expires: Adjust session.gc_maxlifetime value to extend session time.
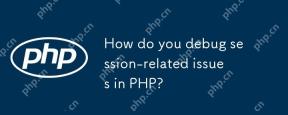
Methods to debug session problems in PHP include: 1. Check whether the session is started correctly; 2. Verify the delivery of the session ID; 3. Check the storage and reading of session data; 4. Check the server configuration. By outputting session ID and data, viewing session file content, etc., you can effectively diagnose and solve session-related problems.
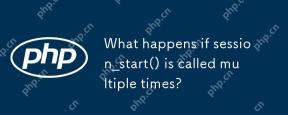
Multiple calls to session_start() will result in warning messages and possible data overwrites. 1) PHP will issue a warning, prompting that the session has been started. 2) It may cause unexpected overwriting of session data. 3) Use session_status() to check the session status to avoid repeated calls.
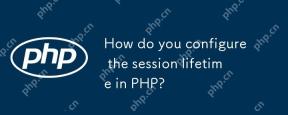
Configuring the session lifecycle in PHP can be achieved by setting session.gc_maxlifetime and session.cookie_lifetime. 1) session.gc_maxlifetime controls the survival time of server-side session data, 2) session.cookie_lifetime controls the life cycle of client cookies. When set to 0, the cookie expires when the browser is closed.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
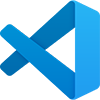
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
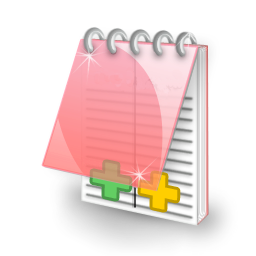
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
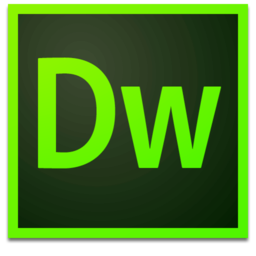
Dreamweaver Mac version
Visual web development tools
