


How does Java use the format() function of the String class to convert data into a string in a specified format?
How does Java use the format() function of the String class to convert data into a string in a specified format?
In Java development, we often encounter situations where we need to convert data into a string in a specified format. Java provides the format() function of the String class, which can convert data into a string according to the specified format. This article will introduce how to use the format() function and demonstrate its usage through code examples.
The format() function of the String class is a static method that can be called directly through the class name. Its syntax is as follows:
public static String format(String format, Object... args)
Among them, the format parameter is a string used to specify the conversion format. The args parameter is a variable parameter used to pass data that needs to be converted.
The format string can contain placeholders, through which data can be inserted into the string according to the specified format. Common placeholders include the following:
-
%c
: Convert characters to strings. -
%d
: Convert integer to string. -
%f
: Convert floating point number to string. -
%s
: Convert any type of data to a string.
The following is a simple example that demonstrates how to use the format() function to convert data into a string in a specified format:
public class FormatDemo { public static void main(String[] args) { String name = "Tom"; int age = 20; double score = 95.5; String result = String.format("姓名:%s,年龄:%d,成绩:%.2f", name, age, score); System.out.println(result); } }
In the above code, we define name, age and score three variables, and use the format() function to convert them into strings according to the specified format. The output result is as follows:
姓名:Tom,年龄:20,成绩:95.50
In the format string, %s
means inserting the string into this position, %d
means inserting the integer into this position, %.2f
means insert the floating point number into this position and keep two decimal places.
In addition to basic types of data, we can also use the format() function to convert other types of data. For example, we can convert a date into a string:
import java.util.Date; import java.text.SimpleDateFormat; public class FormatDemo { public static void main(String[] args) { Date now = new Date(); SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); String result = String.format("当前时间:%s", format.format(now)); System.out.println(result); } }
In the above code, we use the SimpleDateFormat class to format the current date into a string in a specified format, and use the format() function to insert it into the format character String in. The output result is as follows:
当前时间:2022-01-01 12:34:56
Through the format() function, we can flexibly convert various types of data into strings according to the specified format, and easily output, save or transmit them.
It should be noted that the format() function returns a new string, and the original data will not be affected. In addition, when using placeholders, the number and type of placeholders must correspond one-to-one with the incoming data, otherwise an exception will be thrown.
Summary:
The format() function of the String class in Java can convert data into a string according to the specified format. By using placeholders in the format string, we can flexibly insert various types of data. Using the format() function can conveniently format string output and improve the readability of the code.
I hope this article will help you understand how to use the format() function of the String class to convert data into a string, and I hope you can better apply this function in actual development.
The above is the detailed content of How does Java use the format() function of the String class to convert data into a string in a specified format?. For more information, please follow other related articles on the PHP Chinese website!
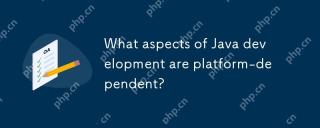
Javadevelopmentisnotentirelyplatform-independentduetoseveralfactors.1)JVMvariationsaffectperformanceandbehavioracrossdifferentOS.2)NativelibrariesviaJNIintroduceplatform-specificissues.3)Filepathsandsystempropertiesdifferbetweenplatforms.4)GUIapplica
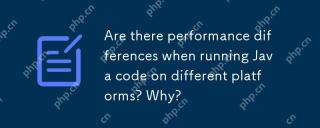
Java code will have performance differences when running on different platforms. 1) The implementation and optimization strategies of JVM are different, such as OracleJDK and OpenJDK. 2) The characteristics of the operating system, such as memory management and thread scheduling, will also affect performance. 3) Performance can be improved by selecting the appropriate JVM, adjusting JVM parameters and code optimization.
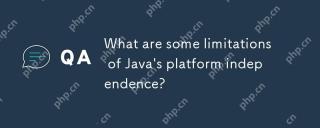
Java'splatformindependencehaslimitationsincludingperformanceoverhead,versioncompatibilityissues,challengeswithnativelibraryintegration,platform-specificfeatures,andJVMinstallation/maintenance.Thesefactorscomplicatethe"writeonce,runanywhere"
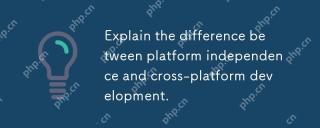
Platformindependenceallowsprogramstorunonanyplatformwithoutmodification,whilecross-platformdevelopmentrequiressomeplatform-specificadjustments.Platformindependence,exemplifiedbyJava,enablesuniversalexecutionbutmaycompromiseperformance.Cross-platformd
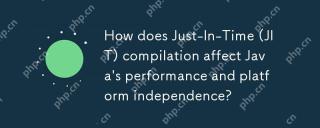
JITcompilationinJavaenhancesperformancewhilemaintainingplatformindependence.1)Itdynamicallytranslatesbytecodeintonativemachinecodeatruntime,optimizingfrequentlyusedcode.2)TheJVMremainsplatform-independent,allowingthesameJavaapplicationtorunondifferen
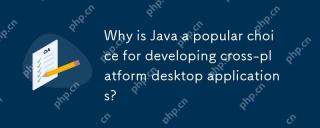
Javaispopularforcross-platformdesktopapplicationsduetoits"WriteOnce,RunAnywhere"philosophy.1)ItusesbytecodethatrunsonanyJVM-equippedplatform.2)LibrarieslikeSwingandJavaFXhelpcreatenative-lookingUIs.3)Itsextensivestandardlibrarysupportscompr
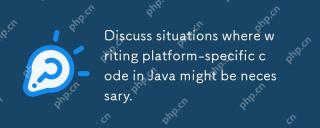
Reasons for writing platform-specific code in Java include access to specific operating system features, interacting with specific hardware, and optimizing performance. 1) Use JNA or JNI to access the Windows registry; 2) Interact with Linux-specific hardware drivers through JNI; 3) Use Metal to optimize gaming performance on macOS through JNI. Nevertheless, writing platform-specific code can affect the portability of the code, increase complexity, and potentially pose performance overhead and security risks.
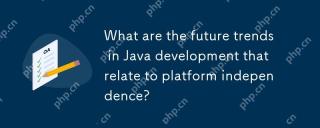
Java will further enhance platform independence through cloud-native applications, multi-platform deployment and cross-language interoperability. 1) Cloud native applications will use GraalVM and Quarkus to increase startup speed. 2) Java will be extended to embedded devices, mobile devices and quantum computers. 3) Through GraalVM, Java will seamlessly integrate with languages such as Python and JavaScript to enhance cross-language interoperability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
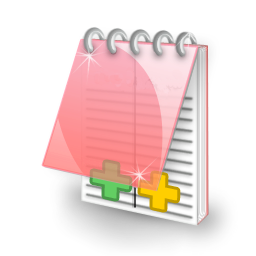
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
