How to elegantly use Go and context for error handling
How to use Go and context elegantly for error handling
In Go programming, error handling is a very important task. Handling errors gracefully improves code readability, maintainability, and stability. The context package of Go language provides us with a very convenient way to handle error-related operations. This article will introduce how to use Go and context elegantly for error handling, and provide relevant code examples.
- Introduction
The error handling mechanism of Go language is implemented by returning an error value. Generally, we will return an error type value in the return value of the function to indicate whether the function was executed successfully. But in actual development, there is often more than one error, but multiple levels of errors. At this point, simply passing all error values to the upper call chain will make the code complex and difficult to read. The context package of Go language can help us better manage and propagate these errors.
- Using the context package
The context package provides a Context type for managing all data related to the request. It can be used to track the lifecycle of a request and pass errors if needed. The following is a sample code that uses the context package for error handling:
package main import ( "context" "errors" "fmt" ) func main() { ctx := context.Background() // 创建一个根Context // 创建一个新的Context,并添加一些与请求相关的数据 ctx = context.WithValue(ctx, "userID", 1001) ctx = context.WithValue(ctx, "isAdmin", true) // 调用一个模拟的业务函数,并传入Context err := businessFunc(ctx) if err != nil { fmt.Println("Error:", err) } else { fmt.Println("Success") } } func businessFunc(ctx context.Context) error { userID := ctx.Value("userID").(int) isAdmin := ctx.Value("isAdmin").(bool) if isAdmin { return nil } else { return errors.New("Permission denied") } }
In the above code, we create a root Context and then use the WithValue method to add some request-related data to the Context, such as userID and isAdmin. Next, we call a business function called businessFunc and pass it the Context as a parameter.
In the implementation of the business function, we obtain the request data through the ctx.Value method, and use this data to determine whether we have permission to perform certain operations. If there is no permission, an error is returned.
The advantage of using the context package is that we can pass the request context information to all related functions without adding some additional parameters to each function. When errors occur, we can easily get and handle them from any function.
- Error propagation and timeout handling
In addition to the WithValue method in the above example, the context package also provides some other methods for propagating and handling errors. The most commonly used methods are WithCancel and WithTimeout.
WithCancel is used to create a cancelable Context. We can use it when we want to cancel some operations when a certain condition is met, or cancel operations when no response is received within a period of time. The following is a sample code that uses WithCancel to timeout a business function:
package main import ( "context" "errors" "fmt" "time" ) func main() { ctx := context.Background() ctx, cancel := context.WithCancel(ctx) go func() { time.Sleep(2 * time.Second) cancel() // 2秒后取消操作 }() err := businessFunc(ctx) if err != nil { fmt.Println("Error:", err) } else { fmt.Println("Success") } } func businessFunc(ctx context.Context) error { select { case <-ctx.Done(): return errors.New("Timeout") default: // 执行一些操作 time.Sleep(3 * time.Second) return nil } }
In the above example, we use WithCancel to create a cancelable Context and wait 2 seconds in a goroutine before calling cancel. function to cancel the operation. In the business function, we use the select statement to listen for messages from the ctx.Done() channel. If a message is received, it means that the Context is canceled and an error is returned.
WithTimeout is similar to WithCancel, but it automatically cancels the operation within a certain period of time. The following is a sample code that uses WithTimeout for timeout processing:
package main import ( "context" "errors" "fmt" "time" ) func main() { ctx := context.Background() // 设置超时时间为2秒 ctx, cancel := context.WithTimeout(ctx, 2*time.Second) defer cancel() // 到达超时时间后自动取消操作 err := businessFunc(ctx) if err != nil { fmt.Println("Error:", err) } else { fmt.Println("Success") } } func businessFunc(ctx context.Context) error { select { case <-ctx.Done(): return errors.New("Timeout") default: // 执行一些操作 time.Sleep(3 * time.Second) return nil } }
In the above example, we use WithTimeout to create a Context with a timeout of 2 seconds, and call cancel before the function exits through the defer keyword function to automatically cancel the operation. In the business function, we use the select statement to listen for messages from the ctx.Done() channel. If a message is received, it means that the timeout has reached and an error will be returned.
- Summary
By using the context package of Go language, we can handle errors more elegantly and propagate error information to related functions. The context package provides a series of methods, such as WithValue, WithCancel and WithTimeout, to easily handle error-related operations. In actual development, we can choose appropriate methods for error handling based on specific needs and scenarios.
I hope this article can help readers use Go and context for error handling gracefully, and improve the readability, maintainability and stability of the code.
The above is the detailed content of How to elegantly use Go and context for error handling. For more information, please follow other related articles on the PHP Chinese website!
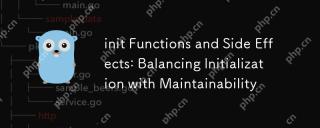
Toensureinitfunctionsareeffectiveandmaintainable:1)Minimizesideeffectsbyreturningvaluesinsteadofmodifyingglobalstate,2)Ensureidempotencytohandlemultiplecallssafely,and3)Breakdowncomplexinitializationintosmaller,focusedfunctionstoenhancemodularityandm
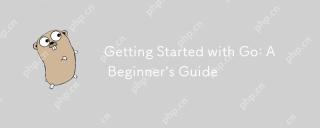
Goisidealforbeginnersandsuitableforcloudandnetworkservicesduetoitssimplicity,efficiency,andconcurrencyfeatures.1)InstallGofromtheofficialwebsiteandverifywith'goversion'.2)Createandrunyourfirstprogramwith'gorunhello.go'.3)Exploreconcurrencyusinggorout
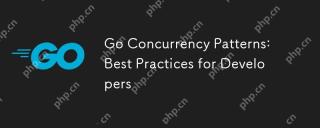
Developers should follow the following best practices: 1. Carefully manage goroutines to prevent resource leakage; 2. Use channels for synchronization, but avoid overuse; 3. Explicitly handle errors in concurrent programs; 4. Understand GOMAXPROCS to optimize performance. These practices are crucial for efficient and robust software development because they ensure effective management of resources, proper synchronization implementation, proper error handling, and performance optimization, thereby improving software efficiency and maintainability.
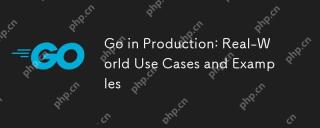
Goexcelsinproductionduetoitsperformanceandsimplicity,butrequirescarefulmanagementofscalability,errorhandling,andresources.1)DockerusesGoforefficientcontainermanagementthroughgoroutines.2)UberscalesmicroserviceswithGo,facingchallengesinservicemanageme
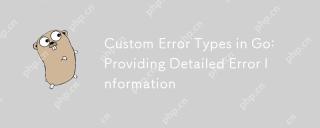
We need to customize the error type because the standard error interface provides limited information, and custom types can add more context and structured information. 1) Custom error types can contain error codes, locations, context data, etc., 2) Improve debugging efficiency and user experience, 3) But attention should be paid to its complexity and maintenance costs.
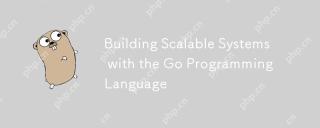
Goisidealforbuildingscalablesystemsduetoitssimplicity,efficiency,andbuilt-inconcurrencysupport.1)Go'scleansyntaxandminimalisticdesignenhanceproductivityandreduceerrors.2)Itsgoroutinesandchannelsenableefficientconcurrentprogramming,distributingworkloa
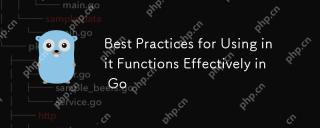
InitfunctionsinGorunautomaticallybeforemain()andareusefulforsettingupenvironmentsandinitializingvariables.Usethemforsimpletasks,avoidsideeffects,andbecautiouswithtestingandloggingtomaintaincodeclarityandtestability.
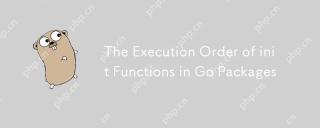
Goinitializespackagesintheordertheyareimported,thenexecutesinitfunctionswithinapackageintheirdefinitionorder,andfilenamesdeterminetheorderacrossmultiplefiles.Thisprocesscanbeinfluencedbydependenciesbetweenpackages,whichmayleadtocomplexinitializations


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
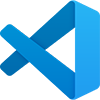
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
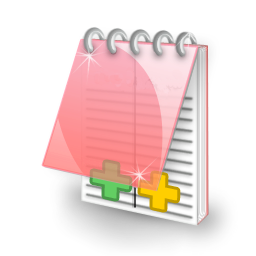
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
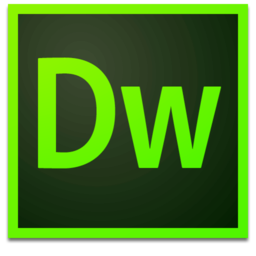
Dreamweaver Mac version
Visual web development tools
