When your VPS is running multiple service applications, but one of them sometimes takes up all the resources, making it impossible to access the server through ssh. You move to using a Kubernetes cluster and set limits for all applications. We then saw some applications being restarted as the OOM-killer fixed the memory "leak" issue.
Of course, OOM is not always a leak problem, it can also be a resource overrun. Leakage problems are most likely caused by program errors. The topic we are talking about today is how to avoid this situation as much as possible.
Excessive resource consumption can hurt the wallet, which means we need to take immediate action.
Don’t optimize prematurely
Now let’s talk about optimization. Hopefully you can understand why we shouldn’t optimize prematurely!
First, optimization may be useless work. Because we should study the entire application first, and your code will most likely not be the bottleneck. What we need is quick results, MVP (Minimum Viable Product, minimum viable product), and then we will consider its problems. #Second, optimization must have a basis. That is to say, every optimization should be based on a benchmark, and we must prove how much profit it brings us. #Third, optimization may bring complexity. What you need to know is that most optimizations make your code less readable. You need to strike this balance.
Optimization suggestions
Now we give some practical suggestions according to the standard entity classification in Go.
1. Arrays and slices
Allocate memory for slices in advance
Try to use the third parameter: <span style="font-size: 15px;"> make([]T, 0, len)</span>
If you don’t know the exact number of elements and the slice is short-lived, you can allocate a larger size to ensure that the slice does not will grow.
Don’t forget to use copy
Try not to use append when copying, such as when merging two or more slices.
Iterate correctly
For a slice containing many elements or large elements, use for to get a single element. This way unnecessary duplication will be avoided.
Multiple Slices
If some operation is performed on the incoming slice and returns a modified result, we can return it. This avoids new memory allocation.
Don't leave unused portions of the slice
If you need to cut off a small piece from the slice and use it only, the main part of the slice will also be retained. The correct approach is to use a new copy of this small slice and throw the old slice to the GC.
2. Strings
Correct splicing
If splicing strings can be completed in one statement, use <span style="font-size: 15px;"> </span>
Operator. If you need to do this in a loop, use <span style="font-size: 15px;">string.Builder</span>
, and use its <span style="font-size: 15px;">Grow</span>
Method pre-specifies the size of <span style="font-size: 15px;">Builder</span>
to reduce the number of memory allocations.
Conversion optimization
string and []byte are very similar in underlying structure, and sometimes strong conversion can be used between these two types to avoid memory allocation.
String resident
Strings can be pooled, thus helping the compiler store the same string only once.
Avoid allocation
We can use map (cascade) instead of composite keys, we can use byte slices. Try not to use the <span style="font-size: 15px;">fmt</span>
package because all its methods use reflection.
3. Structure
Avoid copying large structures
The small structure we understand is no more than 4 fields and no more than one machine word size.
Some typical copy scenes
Project to interface -
Channel reception and transmission Replace elements in the map Add elements to the slice Iterate (range)
Avoid accessing structure fields through pointers
Dereferencing is expensive and we should do it as little as possible, especially in loops. It also loses the ability to use fast registers.
Handling small structures
This work is optimized by the editor, which means it is cheap.
Use alignment to reduce structure size
We can reduce the size of a structure by aligning it (arranging them in the correct order according to the size of the fields) size itself.
4. Functions
Use inline functions or inline them yourself
Try writing small functions that can be inlined by the compiler and it will Fast, even faster than embedding the code in the function yourself. This is especially true for hot paths.
Which ones will not be inline
recovery select block Type declaration defer -
##goroutine for-range
Try to use small parameters because of their duplication will be optimized. Try to keep replication and stack growth balanced on the GC load. Avoid large numbers of parameters and let your program use fast registers (their number is limited).
Named return valuesThis seems more efficient than declaring these variables in the function body.
Save intermediate resultsHelp the compiler optimize your code, save the intermediate results, and then there will be more options to optimize your code.
Use defer carefullyTry not to use defer, or at least don't use it in a loop.
Help hot pathAvoid allocating memory in the hot path, especially for short-lived objects. Make the most common branches (if, switch)
5. MapAllocate memory in advanceSame as slice, when initializing map, specify its size .
Use empty structs as valuesstruct{} is nothing (takes up no memory), so it is very beneficial to use it when passing signals for example.
Clear map
map can only grow, not shrink. When we need to reset the map, deleting all its elements won't help.
Try not to use pointers in keys and values
If the map does not contain pointers, then the GC will not waste precious time on it. Strings also use pointers, so you should use byte arrays instead of strings as keys.
Reduce the number of modifications
Similarly, we don’t want to use pointers, but we can use a combination of map and slice, storing the keys in the map and the values in the slice. This way we can change the value without restrictions.
6. Interface
Calculate memory allocation
Remember that when you want to assign a value to an interface, you first need to copy it somewhere, Then paste the pointer to it. The key is to copy. It turns out that the cost of boxing and unboxing the interface will be approximately the same as an allocation of the struct size.
Selecting the optimal type
In some cases, there is no allocation during boxing and unboxing of an interface. For example, small or Boolean values of variables and constants, structures with one simple field, pointers (including map, channel, func)
Avoid memory allocation
As elsewhere, try to avoid unnecessary allocations. For example assigning one interface to another instead of boxing twice.
Use only when needed
Avoid using interfaces in frequently called function parameters and return results. We don't need additional unpacking operations. Reduce the frequency of using interface method calls as it prevents inlining.
7. Pointers, Channels, Bounds Checks
Avoid unnecessary dereferences
Especially in loops as it turns out to be too expensive . Dereferencing is something we don't want to do at our own expense.
Using channels is inefficient
Channel synchronization is slower than other synchronization primitive methods. In addition, the more cases in select, the slower our program will be. However, select, case plus default have been optimized.
Avoid unnecessary bounds checks
This is also expensive and we should avoid it. For example, check (get) the maximum slice index only once, not multiple times. It’s best to try getting the extreme option now.
Summary
Throughout this article, we saw some of the same optimization rules.
Help the compiler make the right decision and it will thank you. Allocate memory at compile time, use intermediate results, and try to keep your code readable.
I reiterate that for implicit optimization, benchmarks are mandatory. If something that worked yesterday won't work tomorrow because the compiler changes too quickly between versions, and vice versa.
Don’t forget to use Go’s built-in profiling and tracing tools.
The above is the detailed content of Go: Simple optimization notes. For more information, please follow other related articles on the PHP Chinese website!
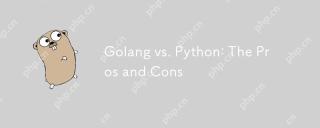
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
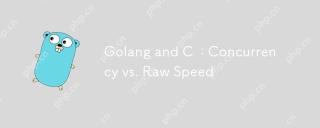
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
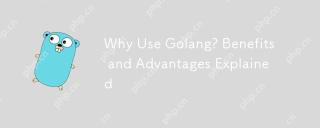
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
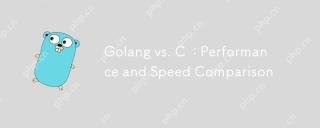
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
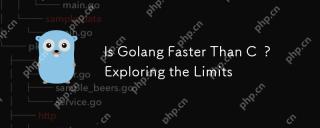
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
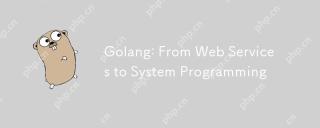
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
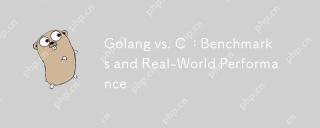
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
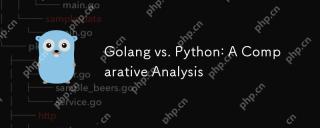
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.