Golang and Vault: Building a highly reliable access control system
Golang and Vault: Building a highly reliable access control system
Introduction:
In today's information age, the importance of access control systems cannot be ignored. As systems continue to grow in size and the sensitivity of data continues to increase, protecting data from the risk of unauthorized access becomes even more critical. This article will introduce how to use Golang and Vault to build a highly reliable access control system, and provide corresponding code examples to help readers better understand.
1. Introduction to Golang
Golang, also known as Go language, is an open source programming language developed by Google. Its advantage is that it has C language-like efficiency and strong type syntax, while providing garbage collection and concurrent programming features. Golang is widely used to build high-performance distributed systems and network applications.
2. Introduction to Vault
Vault is a tool for protecting sensitive data, developed by HashiCorp and open source. It provides a reliable way to store and access various secrets, credentials and sensitive information such as API keys, database credentials, etc. Vault helps users protect data from the risk of unauthorized access by providing access control and secret management capabilities.
3. Steps to build an access control system
- Installing and configuring Vault
First, we need to install Vault locally or on the server and configure it accordingly. Installation and configuration details are available through Vault's official website. - Writing Golang program
Next, we use Golang to write a program to connect to Vault and authorize access. Here is a simple code example to illustrate how to obtain credentials from Vault and perform access control:
package main import ( "fmt" "github.com/hashicorp/vault/api" ) func main() { // 连接到Vault服务器 client, err := api.NewClient(&api.Config{ Address: "http://localhost:8200", }) if err != nil { panic(err) } // 身份验证 client.SetToken("your_vault_token") // 从Vault中获取凭证 secret, err := client.Logical().Read("secret/data/myapp") if err != nil { panic(err) } // 检查用户权限 if secret != nil && secret.Data["role"] == "admin" { fmt.Println("You have admin access!") } else { fmt.Println("Access denied!") } }
In this example, we first connect to the Vault server, then authenticate and set the access token Card. Next, we read the credentials under a specific path (secret/data/myapp) from Vault and perform access control based on the user's permissions.
- Configure access control policy
In order to use Vault's access control function, we need to configure the corresponding access control policy. This can be configured via Vault's CLI or API. The following is a simple example policy configuration:
path "secret/data/myapp" { capabilities = ["read"] } path "secret/data/admin" { capabilities = ["read"] }
This example policy specifies the access control rules for the paths "secret/data/myapp" and "secret/data/admin", restricting the user to Read the credentials under these paths. More complex policies and rules can be configured according to actual needs.
4. Summary
In this article, we introduced how to use Golang and Vault to build a highly reliable access control system. With the efficient performance of Golang and the power of Vault, we can ensure that only authorized users can access sensitive data. At the same time, this article provides corresponding code examples to help readers better understand how to implement an access control system. I hope this article will be helpful to readers when building a secure access control system!
The above is the detailed content of Golang and Vault: Building a highly reliable access control system. For more information, please follow other related articles on the PHP Chinese website!
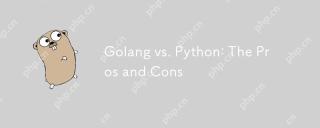
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
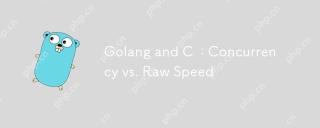
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
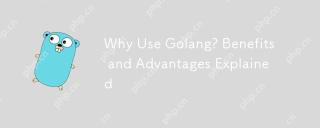
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
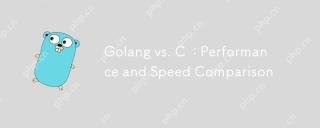
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
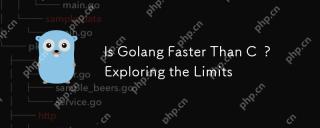
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
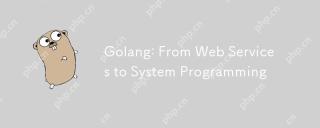
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
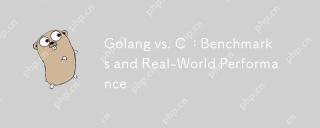
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
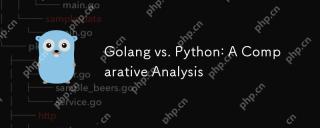
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
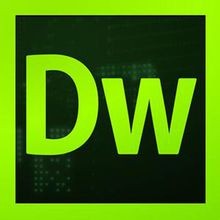
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
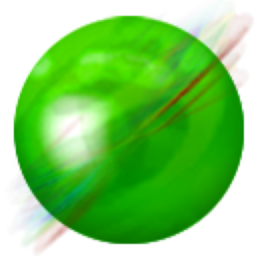
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment