


How to match consecutive occurrences of the same letters using regular expressions in Go language
How to use regular expressions to match consecutive identical letters in Go language
Regular expression is a powerful text pattern matching tool, and there is also rich regular expression support in Go language. We can use regular expressions to match consecutive occurrences of the same letters to find and process some specific character patterns.
Below we will use a specific example to introduce how to use regular expressions to match consecutive occurrences of the same letters in the Go language.
package main import ( "fmt" "regexp" ) func main() { str := "aaabbccdd" pattern := `(.)+` re := regexp.MustCompile(pattern) matches := re.FindAllString(str, -1) if len(matches) > 0 { fmt.Println("连续出现的相同字母:") for _, match := range matches { fmt.Println(match) } } else { fmt.Println("没有连续出现的相同字母") } }
In the above code, we first define a string str, which contains a series of consecutive identical letters. Next, we defined a regular expression pattern, where (.)
means matching any character, followed by at least one consecutive repetition of the same character.
Then, we use regexp.MustCompile(pattern)
to compile the regular expression pattern, and find all the substrings in the string str that match the pattern through the FindAllString
method string. Finally, we loop through and print out all consecutive substrings of the same letters that meet the criteria.
Run the above code, we will get the following output:
连续出现的相同字母: aaa bb dd
The above results show that in the string str, we successfully matched three groups of the same letters that appear continuously. In this way, we can further process these consecutive identical letters as needed.
To summarize, using regular expressions to match consecutive occurrences of the same letters is a concise and efficient method. The Go language provides powerful regular expression support, and we can make full use of this feature to achieve various text pattern matching needs. I hope this article can help you use regular expressions to match consecutive identical letters in the Go language.
The above is the detailed content of How to match consecutive occurrences of the same letters using regular expressions in Go language. For more information, please follow other related articles on the PHP Chinese website!
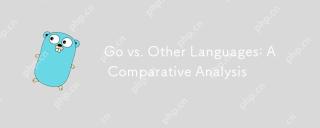
Goisastrongchoiceforprojectsneedingsimplicity,performance,andconcurrency,butitmaylackinadvancedfeaturesandecosystemmaturity.1)Go'ssyntaxissimpleandeasytolearn,leadingtofewerbugsandmoremaintainablecode,thoughitlacksfeatureslikemethodoverloading.2)Itpe
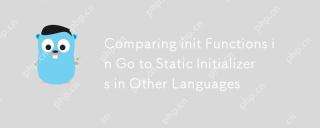
Go'sinitfunctionandJava'sstaticinitializersbothservetosetupenvironmentsbeforethemainfunction,buttheydifferinexecutionandcontrol.Go'sinitissimpleandautomatic,suitableforbasicsetupsbutcanleadtocomplexityifoverused.Java'sstaticinitializersoffermorecontr
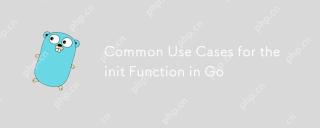
ThecommonusecasesfortheinitfunctioninGoare:1)loadingconfigurationfilesbeforethemainprogramstarts,2)initializingglobalvariables,and3)runningpre-checksorvalidationsbeforetheprogramproceeds.Theinitfunctionisautomaticallycalledbeforethemainfunction,makin
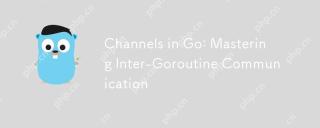
ChannelsarecrucialinGoforenablingsafeandefficientcommunicationbetweengoroutines.Theyfacilitatesynchronizationandmanagegoroutinelifecycle,essentialforconcurrentprogramming.Channelsallowsendingandreceivingvalues,actassignalsforsynchronization,andsuppor
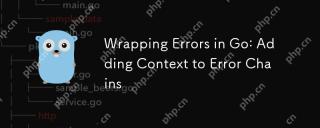
In Go, errors can be wrapped and context can be added via errors.Wrap and errors.Unwrap methods. 1) Using the new feature of the errors package, you can add context information during error propagation. 2) Help locate the problem by wrapping errors through fmt.Errorf and %w. 3) Custom error types can create more semantic errors and enhance the expressive ability of error handling.
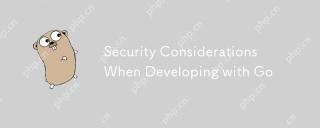
Gooffersrobustfeaturesforsecurecoding,butdevelopersmustimplementsecuritybestpracticeseffectively.1)UseGo'scryptopackageforsecuredatahandling.2)Manageconcurrencywithsynchronizationprimitivestopreventraceconditions.3)SanitizeexternalinputstoavoidSQLinj
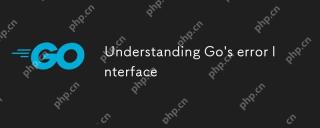
Go's error interface is defined as typeerrorinterface{Error()string}, allowing any type that implements the Error() method to be considered an error. The steps for use are as follows: 1. Basically check and log errors, such as iferr!=nil{log.Printf("Anerroroccurred:%v",err)return}. 2. Create a custom error type to provide more information, such as typeMyErrorstruct{MsgstringDetailstring}. 3. Use error wrappers (since Go1.13) to add context without losing the original error message,
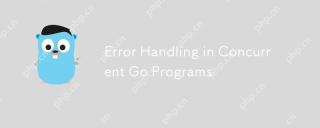
ToeffectivelyhandleerrorsinconcurrentGoprograms,usechannelstocommunicateerrors,implementerrorwatchers,considertimeouts,usebufferedchannels,andprovideclearerrormessages.1)Usechannelstopasserrorsfromgoroutinestothemainfunction.2)Implementanerrorwatcher


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
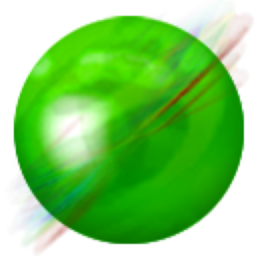
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Chinese version
Chinese version, very easy to use
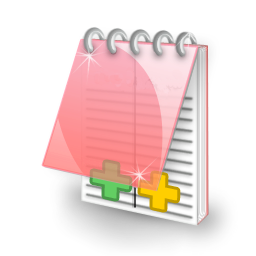
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
