Go vs. Other Languages: A Comparative Analysis
Go is a strong choice for projects needing simplicity, performance, and concurrency, but it may lack in advanced features and ecosystem maturity. 1) Go's syntax is simple and easy to learn, leading to fewer bugs and more maintainable code, though it lacks features like method overloading. 2) It performs well for systems programming and microservices, but for heavy numerical computations, languages like Python with NumPy might be better. 3) Go's goroutines and channels excel in concurrent programming, but can be overused, leading to potential performance issues. 4) While Go has a robust standard library, its ecosystem lags behind languages like Python or JavaScript. 5) Go excels in deployment and scalability with static linking and cross-compilation, ideal for DevOps and cloud-native applications. 6) Its explicit error handling can result in verbose code, which might be less appealing compared to exception-based languages like Python.
When diving into the world of programming, choosing the right language for your project can feel like navigating a labyrinth. Today, we're focusing on Go, a language that's been gaining traction for its simplicity and efficiency. How does Go stack up against other languages? Let's explore this question by comparing Go with some of its contemporaries, sharing personal insights, and diving deep into what makes Go a compelling choice or where it might fall short.
Go, or Golang as it's affectionately known, was designed by Google to be efficient, readable, and scalable. It's particularly praised for its concurrency model, which makes it a go-to for building high-performance applications. But how does it compare to languages like Python, Java, and C ? Let's delve into the details.
Go's simplicity is one of its strongest suits. When I first started using Go, I was amazed at how quickly I could pick it up. Unlike C or Java, which can feel overwhelming with their complex syntax and extensive libraries, Go's syntax is clean and straightforward. Here's a quick example to show you what I mean:
package main import "fmt" func main() { fmt.Println("Hello, Go!") }
This simplicity isn't just about ease of learning; it also translates into fewer bugs and more maintainable code. However, this simplicity comes at a cost. Go lacks some of the advanced features found in other languages. For instance, Go doesn't support method overloading, which can be a limitation when coming from languages like Java or C .
When it comes to performance, Go shines. Its compiled nature and garbage collection make it a strong contender against languages like C for systems programming. I've used Go to build microservices that needed to handle thousands of requests per second, and it performed admirably. Here's a snippet of a simple HTTP server in Go to illustrate its efficiency:
package main import ( "fmt" "net/http" ) func handler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hi there, I love %s!", r.URL.Path[1:]) } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) }
However, Go's performance isn't always the best choice. For tasks that require heavy numerical computations, languages like Python with libraries like NumPy might be more suitable due to their optimized performance for such tasks.
Concurrency is where Go truly stands out. Its goroutines and channels make concurrent programming a breeze. I remember working on a project where we needed to process large datasets in parallel. Go's concurrency model allowed us to write clean, efficient code that scaled beautifully. Here's a simple example of using goroutines and channels:
package main import ( "fmt" "time" ) func say(s string, c chan bool) { for i := 0; i < 5; i { time.Sleep(100 * time.Millisecond) fmt.Println(s) } c <- true } func main() { c := make(chan bool) go say("world", c) go say("Hello", c) <-c <-c }
While Go's concurrency model is powerful, it can be a double-edged sword. The simplicity of goroutines can lead to overuse, potentially causing performance issues if not managed properly. It's crucial to understand the underlying mechanics to avoid common pitfalls like goroutine leaks.
In terms of ecosystem, Go has a robust standard library and a growing community. However, it still lags behind languages like Python or JavaScript in terms of third-party libraries. When I needed a specific library for a project, I often found that Python had a more mature ecosystem. This can be a significant factor when choosing a language for a project with specific requirements.
One of the areas where Go excels is in deployment and scalability. Its static linking and cross-compilation capabilities make it a favorite for DevOps and cloud-native applications. I've deployed Go applications on various platforms without worrying about dependencies, which is a huge advantage over languages like Python or Java.
However, Go's error handling can be a point of contention. Its explicit error handling can lead to verbose code, which some developers find cumbersome compared to languages like Python, which use exceptions. Here's an example of error handling in Go:
package main import ( "fmt" "os" ) func main() { f, err := os.Open("filename.ext") if err != nil { fmt.Println("Error:", err) return } defer f.Close() // Use f here }
While this approach ensures that errors are not silently ignored, it can lead to a lot of boilerplate code. It's a trade-off between explicitness and conciseness, and it's something to consider when choosing Go.
In conclusion, Go is a powerful language with its strengths in simplicity, performance, and concurrency. It's an excellent choice for building scalable, high-performance applications, especially in the cloud-native space. However, it's not without its drawbacks, particularly in terms of advanced features and ecosystem maturity. When deciding whether to use Go, consider your project's specific needs and weigh the pros and cons carefully. From my experience, Go has been a game-changer for many of my projects, but it's not a one-size-fits-all solution. Choose wisely, and happy coding!
The above is the detailed content of Go vs. Other Languages: A Comparative Analysis. For more information, please follow other related articles on the PHP Chinese website!
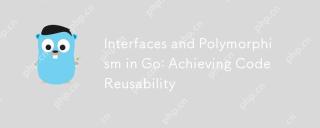
InterfacesandpolymorphisminGoenhancecodereusabilityandmaintainability.1)Defineinterfacesattherightabstractionlevel.2)Useinterfacesfordependencyinjection.3)Profilecodetomanageperformanceimpacts.
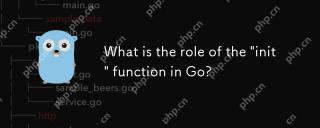
TheinitfunctioninGorunsautomaticallybeforethemainfunctiontoinitializepackagesandsetuptheenvironment.It'susefulforsettingupglobalvariables,resources,andperformingone-timesetuptasksacrossanypackage.Here'showitworks:1)Itcanbeusedinanypackage,notjusttheo
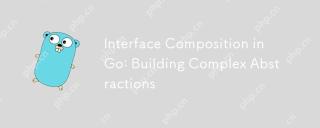
Interface combinations build complex abstractions in Go programming by breaking down functions into small, focused interfaces. 1) Define Reader, Writer and Closer interfaces. 2) Create complex types such as File and NetworkStream by combining these interfaces. 3) Use ProcessData function to show how to handle these combined interfaces. This approach enhances code flexibility, testability, and reusability, but care should be taken to avoid excessive fragmentation and combinatorial complexity.
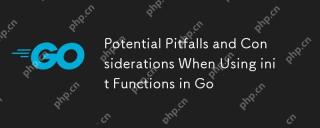
InitfunctionsinGoareautomaticallycalledbeforethemainfunctionandareusefulforsetupbutcomewithchallenges.1)Executionorder:Multipleinitfunctionsrunindefinitionorder,whichcancauseissuesiftheydependoneachother.2)Testing:Initfunctionsmayinterferewithtests,b
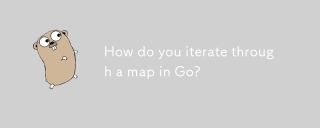
Article discusses iterating through maps in Go, focusing on safe practices, modifying entries, and performance considerations for large maps.Main issue: Ensuring safe and efficient map iteration in Go, especially in concurrent environments and with l
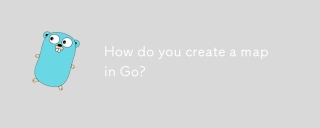
The article discusses creating and manipulating maps in Go, including initialization methods and adding/updating elements.
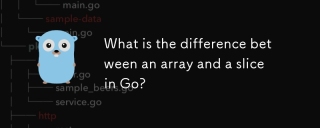
The article discusses differences between arrays and slices in Go, focusing on size, memory allocation, function passing, and usage scenarios. Arrays are fixed-size, stack-allocated, while slices are dynamic, often heap-allocated, and more flexible.
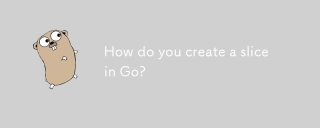
The article discusses creating and initializing slices in Go, including using literals, the make function, and slicing existing arrays or slices. It also covers slice syntax and determining slice length and capacity.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
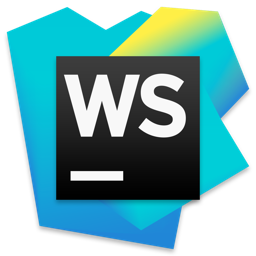
WebStorm Mac version
Useful JavaScript development tools
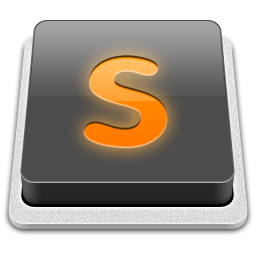
SublimeText3 Mac version
God-level code editing software (SublimeText3)
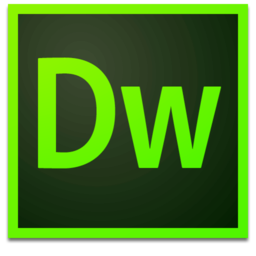
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor
