Go offers robust features for secure coding, but developers must implement security best practices effectively. 1) Use Go's crypto package for secure data handling. 2) Manage concurrency with synchronization primitives to prevent race conditions. 3) Sanitize external inputs to avoid SQL injection and XSS attacks. 4) Regularly update Go's standard library and dependencies to address vulnerabilities.
In the realm of software development, security is not just a feature—it's a fundamental necessity. When it comes to developing applications with Go, or Golang, the language offers several built-in features that make it a robust choice for secure coding. But, like any tool, the effectiveness of Go in building secure applications hinges on the developer's understanding and implementation of security best practices. So, let's dive into the security considerations you should keep in mind when developing with Go, sharing some personal experiences and insights along the way.
Go's design philosophy emphasizes simplicity and efficiency, which inherently supports secure coding practices. From my experience, one of the standout features of Go is its static typing and memory safety, which helps prevent common errors like buffer overflows that can be exploited by attackers. However, it's not just about what Go gives you; it's also about how you use it. Let's explore some key areas where security considerations are crucial.
When working with Go, one of the first things you'll notice is its straightforward approach to handling data. Go's standard library includes packages like crypto
for cryptographic operations, which are essential for tasks like encryption and secure communication. Here's a snippet of how you might use the crypto/sha256
package to hash data:
package main import ( "crypto/sha256" "fmt" ) func main() { data := []byte("Hello, Go!") hash := sha256.Sum256(data) fmt.Printf("Hash: %x\n", hash) }
This code demonstrates a simple use of SHA-256 hashing, but remember, when dealing with security-sensitive data, you need to ensure that your hashing algorithms are up to date and secure.
Another aspect to consider is Go's built-in support for concurrency through goroutines and channels. While this is a powerful feature for building efficient applications, it also introduces potential security risks if not managed properly. For instance, race conditions can lead to unpredictable behavior, which attackers might exploit. To mitigate this, always use synchronization primitives like sync.Mutex
or sync.RWMutex
to protect shared resources:
package main import ( "fmt" "sync" ) var counter int var mutex sync.Mutex func increment() { mutex.Lock() counter mutex.Unlock() } func main() { var wg sync.WaitGroup for i := 0; i < 1000; i { wg.Add(1) go func() { defer wg.Done() increment() }() } wg.Wait() fmt.Println("Final counter:", counter) }
This example shows how to safely increment a shared counter using a mutex, avoiding race conditions that could lead to security vulnerabilities.
When it comes to handling external input, such as user data or network requests, Go's standard library offers robust tools like net/http
for building web services. However, it's crucial to validate and sanitize all inputs to prevent attacks like SQL injection or cross-site scripting (XSS). Here's a simple example of how you might sanitize input using the html/template
package:
package main import ( "html/template" "net/http" ) func handler(w http.ResponseWriter, r *http.Request) { input := r.FormValue("user_input") tmpl := template.Must(template.New("index").Parse(`<p>{{.}}</p>`)) tmpl.Execute(w, template.HTMLEscapeString(input)) } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) }
This code snippet demonstrates how to escape user input to prevent XSS attacks, a common security pitfall in web applications.
In my journey with Go, I've learned that while the language provides many tools for secure coding, it's the developer's responsibility to use them effectively. For instance, Go's lack of exceptions might seem like a limitation, but it actually encourages developers to handle errors explicitly, reducing the chance of silent failures that could be exploited.
One of the challenges I've faced is keeping up with the latest security patches and updates for Go's standard library. It's essential to regularly check for updates and apply them promptly to ensure your applications remain secure. Additionally, using tools like go vet
and golint
can help catch potential security issues in your code before they become problems.
Another area where I've seen developers stumble is in the management of dependencies. Go's module system has improved significantly, but it's still important to ensure that all your dependencies are up to date and free from known vulnerabilities. Tools like go mod tidy
can help manage your dependencies effectively.
In conclusion, developing with Go offers a solid foundation for building secure applications, thanks to its design and built-in features. However, security is an ongoing journey, not a destination. By staying vigilant, keeping up with best practices, and leveraging Go's tools effectively, you can create robust and secure applications. Remember, the key to security in Go, or any language, is a combination of understanding the tools at your disposal and applying them with care and attention to detail.
The above is the detailed content of Security Considerations When Developing with Go. For more information, please follow other related articles on the PHP Chinese website!
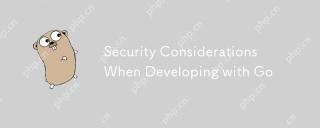
Gooffersrobustfeaturesforsecurecoding,butdevelopersmustimplementsecuritybestpracticeseffectively.1)UseGo'scryptopackageforsecuredatahandling.2)Manageconcurrencywithsynchronizationprimitivestopreventraceconditions.3)SanitizeexternalinputstoavoidSQLinj
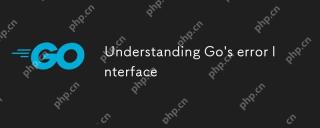
Go's error interface is defined as typeerrorinterface{Error()string}, allowing any type that implements the Error() method to be considered an error. The steps for use are as follows: 1. Basically check and log errors, such as iferr!=nil{log.Printf("Anerroroccurred:%v",err)return}. 2. Create a custom error type to provide more information, such as typeMyErrorstruct{MsgstringDetailstring}. 3. Use error wrappers (since Go1.13) to add context without losing the original error message,
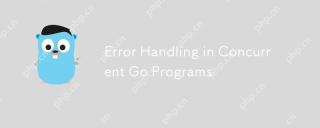
ToeffectivelyhandleerrorsinconcurrentGoprograms,usechannelstocommunicateerrors,implementerrorwatchers,considertimeouts,usebufferedchannels,andprovideclearerrormessages.1)Usechannelstopasserrorsfromgoroutinestothemainfunction.2)Implementanerrorwatcher
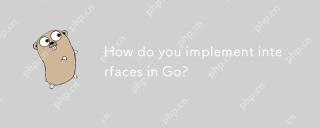
In Go language, the implementation of the interface is performed implicitly. 1) Implicit implementation: As long as the type contains all methods defined by the interface, the interface will be automatically satisfied. 2) Empty interface: All types of interface{} types are implemented, and moderate use can avoid type safety problems. 3) Interface isolation: Design a small but focused interface to improve the maintainability and reusability of the code. 4) Test: The interface helps to unit test by mocking dependencies. 5) Error handling: The error can be handled uniformly through the interface.
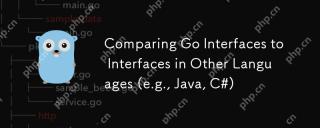
Go'sinterfacesareimplicitlyimplemented,unlikeJavaandC#whichrequireexplicitimplementation.1)InGo,anytypewiththerequiredmethodsautomaticallyimplementsaninterface,promotingsimplicityandflexibility.2)JavaandC#demandexplicitinterfacedeclarations,offeringc
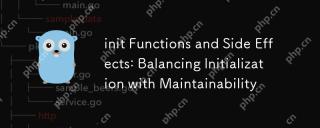
Toensureinitfunctionsareeffectiveandmaintainable:1)Minimizesideeffectsbyreturningvaluesinsteadofmodifyingglobalstate,2)Ensureidempotencytohandlemultiplecallssafely,and3)Breakdowncomplexinitializationintosmaller,focusedfunctionstoenhancemodularityandm
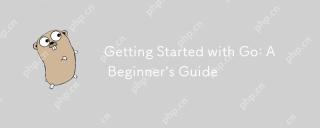
Goisidealforbeginnersandsuitableforcloudandnetworkservicesduetoitssimplicity,efficiency,andconcurrencyfeatures.1)InstallGofromtheofficialwebsiteandverifywith'goversion'.2)Createandrunyourfirstprogramwith'gorunhello.go'.3)Exploreconcurrencyusinggorout
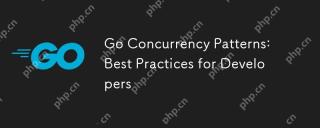
Developers should follow the following best practices: 1. Carefully manage goroutines to prevent resource leakage; 2. Use channels for synchronization, but avoid overuse; 3. Explicitly handle errors in concurrent programs; 4. Understand GOMAXPROCS to optimize performance. These practices are crucial for efficient and robust software development because they ensure effective management of resources, proper synchronization implementation, proper error handling, and performance optimization, thereby improving software efficiency and maintainability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
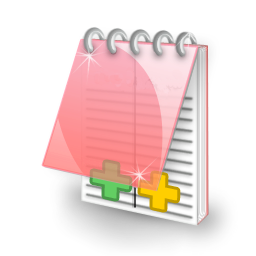
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
