


PHP programming practices on how to prevent session hijacking attacks
How to prevent session hijacking attacks in PHP programming practices
With the development of the Internet, more and more websites and applications rely on sessions to manage user identities and permissions. However, session hijacking attacks have become a major threat to network security. In this article, we will introduce some PHP programming practices to prevent session hijacking attacks and provide some code examples.
- Connect using HTTPS
Session hijacking attacks are usually carried out by stealing the user's session ID. To prevent this attack, we first ensure that the user's session ID is encrypted during transmission. Using HTTPS connections can effectively protect the user's session ID, making it impossible for attackers to obtain the user's sensitive information.
Modify the PHP configuration file php.ini and enable HTTPS support:
session.cookie_secure = true
- Set the HttpOnly flag of the session cookie
The HttpOnly flag can prevent JavaScript from accessing the session cookies. In this way, even if an attacker injects malicious code through an XSS attack, the session cookie cannot be obtained, thereby reducing the risk of session hijacking.
When setting the session cookie, you need to add the HttpOnly flag:
session_set_cookie_params(0, '/', '', true, true);
- Use a randomly generated session ID
Session hijacking attackers often guess the session ID to obtain the user's session permissions. Therefore, using randomly generated session IDs can make it harder for an attacker to guess.
In PHP, we can randomly generate session IDs by modifying the session ID generation method:
function generate_session_id() { $characters = '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'; $session_id = ''; for ($i = 0; $i < 32; $i++) { $session_id .= $characters[rand(0, strlen($characters) - 1)]; } return $session_id; } session_id(generate_session_id());
- Limit the life cycle of the session
Sessions that are valid for a long time are easily exploited by attackers. To prevent session hijacking attacks, we should limit the lifetime of the session. You can use the following code to set the expiration time of the session:
session_start(); if (isset($_SESSION['LAST_ACTIVITY']) && (time() - $_SESSION['LAST_ACTIVITY'] > 3600)) { session_unset(); session_destroy(); } $_SESSION['LAST_ACTIVITY'] = time();
The above code will check the last activity time of the session, and destroy the session if there is no activity for more than one hour.
- Monitor the IP address and user agent of the session
Attackers often carry out hijacking attacks by forging sessions. To prevent this, we should store the user's IP address and user agent information in the session and verify it on every request.
session_start(); if (isset($_SESSION['REMOTE_ADDR']) && $_SESSION['REMOTE_ADDR'] != $_SERVER['REMOTE_ADDR']) { session_unset(); session_destroy(); } if (isset($_SESSION['HTTP_USER_AGENT']) && $_SESSION['HTTP_USER_AGENT'] != $_SERVER['HTTP_USER_AGENT']) { session_unset(); session_destroy(); } $_SESSION['REMOTE_ADDR'] = $_SERVER['REMOTE_ADDR']; $_SESSION['HTTP_USER_AGENT'] = $_SERVER['HTTP_USER_AGENT'];
With the above code, if the user's IP address or user agent information changes, the session will be destroyed.
Summary:
The above are some PHP programming practices to prevent session hijacking attacks. When it comes to session security, be cautious and take as many steps as possible to improve security. At the same time, we should also pay close attention to the latest security vulnerabilities and attack techniques, and promptly update and optimize the code to deal with changing threats.
(Note: This article is only an example. Specific security practices may vary depending on application scenarios. Readers need to design corresponding security policies and protective measures based on specific issues in actual applications)
The above is the detailed content of PHP programming practices on how to prevent session hijacking attacks. For more information, please follow other related articles on the PHP Chinese website!
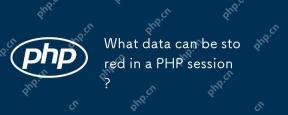
PHPsessionscanstorestrings,numbers,arrays,andobjects.1.Strings:textdatalikeusernames.2.Numbers:integersorfloatsforcounters.3.Arrays:listslikeshoppingcarts.4.Objects:complexstructuresthatareserialized.
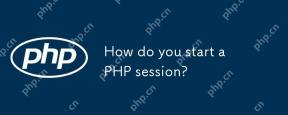
TostartaPHPsession,usesession_start()atthescript'sbeginning.1)Placeitbeforeanyoutputtosetthesessioncookie.2)Usesessionsforuserdatalikeloginstatusorshoppingcarts.3)RegeneratesessionIDstopreventfixationattacks.4)Considerusingadatabaseforsessionstoragei
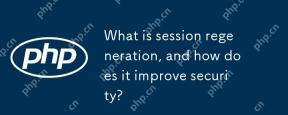
Session regeneration refers to generating a new session ID and invalidating the old ID when the user performs sensitive operations in case of session fixed attacks. The implementation steps include: 1. Detect sensitive operations, 2. Generate new session ID, 3. Destroy old session ID, 4. Update user-side session information.
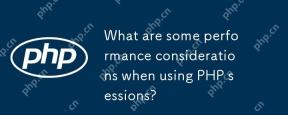
PHP sessions have a significant impact on application performance. Optimization methods include: 1. Use a database to store session data to improve response speed; 2. Reduce the use of session data and only store necessary information; 3. Use a non-blocking session processor to improve concurrency capabilities; 4. Adjust the session expiration time to balance user experience and server burden; 5. Use persistent sessions to reduce the number of data read and write times.
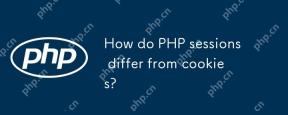
PHPsessionsareserver-side,whilecookiesareclient-side.1)Sessionsstoredataontheserver,aremoresecure,andhandlelargerdata.2)Cookiesstoredataontheclient,arelesssecure,andlimitedinsize.Usesessionsforsensitivedataandcookiesfornon-sensitive,client-sidedata.
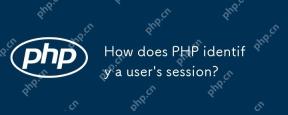
PHPidentifiesauser'ssessionusingsessioncookiesandsessionIDs.1)Whensession_start()iscalled,PHPgeneratesauniquesessionIDstoredinacookienamedPHPSESSIDontheuser'sbrowser.2)ThisIDallowsPHPtoretrievesessiondatafromtheserver.
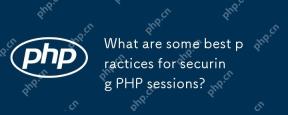
The security of PHP sessions can be achieved through the following measures: 1. Use session_regenerate_id() to regenerate the session ID when the user logs in or is an important operation. 2. Encrypt the transmission session ID through the HTTPS protocol. 3. Use session_save_path() to specify the secure directory to store session data and set permissions correctly.
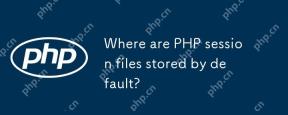
PHPsessionfilesarestoredinthedirectoryspecifiedbysession.save_path,typically/tmponUnix-likesystemsorC:\Windows\TemponWindows.Tocustomizethis:1)Usesession_save_path()tosetacustomdirectory,ensuringit'swritable;2)Verifythecustomdirectoryexistsandiswrita


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
