How Go language solves concurrency competition problems
Methods to solve the problem of concurrent data competition in Go language development
With the rapid development of the Internet, large-scale concurrent processing has become a common need in modern software development. As a powerful tool for developing high-concurrency applications, Go language greatly simplifies the complexity of concurrent programming through its unique concurrency model and rich concurrency primitives. However, one of the most commonly encountered problems in concurrent programming is data races, which can lead to undefined behavior and erroneous results of the program. This article will introduce some methods to solve the problem of concurrent data competition in Go language development.
- Using Mutex (Mutex)
Mutex is a basic synchronization primitive that ensures that only one goroutine can access protected shared resources at the same time. In Go language, you can use the Mutex type in the sync package to implement a mutex lock. By using the Lock() method to acquire the lock before the key code segment, and using the Unlock() method to release the lock after the code segment is executed, data competition problems caused by concurrent reading and writing can be effectively avoided. - Using read-write locks (RWMutex)
Similar to mutex locks, read-write locks are a synchronization primitive used to protect shared resources. The RWMutex type provides separate locks, allowing multiple goroutines to read shared resources at the same time, but only allows one goroutine to perform write operations. By using the RLock() method before the critical code segment to acquire the read lock, and using the RUnlock() method to release the read lock after the code segment is executed, data competition between multiple read operations can be avoided. The write lock uses the Lock() and Unlock() methods to ensure that only one goroutine can access the shared resource during a write operation. - Using Channel(Channel)
Channel is an important mechanism to achieve concurrent communication in Go language. The use of channels can avoid data race problems because channels ensure synchronization between concurrent read and write operations. By encapsulating shared resources in a channel with read and write permissions, you can ensure that only one goroutine can access the shared resources and avoid data competition issues. Read and write operations on shared resources are implemented by sending or receiving data to the channel. At the same time, by using buffered channels, the performance of concurrent processing can be improved and blocking between concurrent read and write operations can be alleviated. - Using atomic operations (Atomic)
The sync/atomic package of Go language provides atomic operation functions, which can ensure that update operations on shared resources are atomic and avoid data competition problems. Atomic operations do not require locking and therefore perform more efficiently. By using the functions provided in the atomic package such as AddInt32, LoadInt32, StoreInt32, etc., atomic reading and updating of shared resources can be achieved. - Using synchronization primitives (Once, WaitGroup, etc.)
Go language provides some synchronization primitives to help solve data competition problems in concurrent programming. For example, the Once type in the sync package ensures that a function is executed only once, avoiding data race problems caused by concurrent calls. In addition, the WaitGroup type can be used to wait for the end of a group of goroutines to ensure that all goroutines have been executed before continuing to execute subsequent code, thereby avoiding conflicts in concurrent reading and writing of shared resources.
To sum up, there are many ways to solve the problem of concurrent data competition in Go language development. In actual development, you can choose a suitable method according to specific scenarios and needs. Whether you use locks, channels, atomic operations, or other synchronization primitives, you should try to reduce the complexity of concurrent programming while ensuring program correctness. By properly applying these methods, we can more easily develop efficient and stable concurrent applications.
The above is the detailed content of How Go language solves concurrency competition problems. For more information, please follow other related articles on the PHP Chinese website!
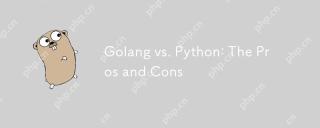
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
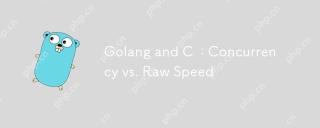
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
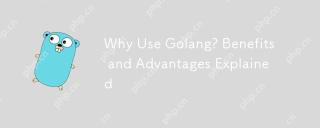
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
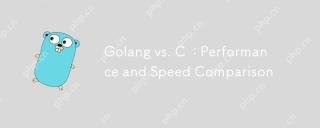
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
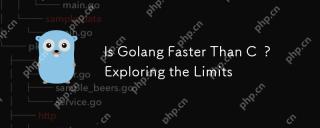
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
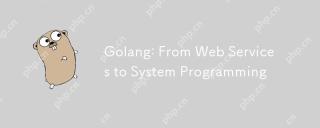
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
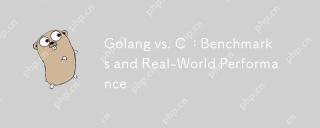
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
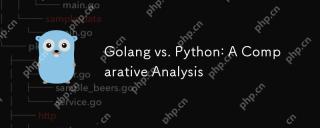
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
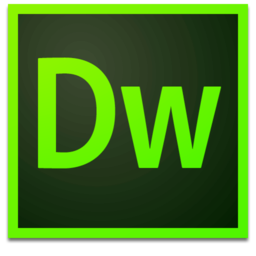
Dreamweaver Mac version
Visual web development tools
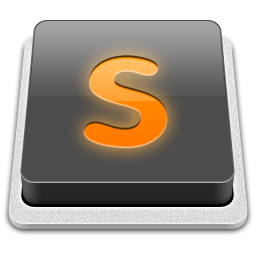
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
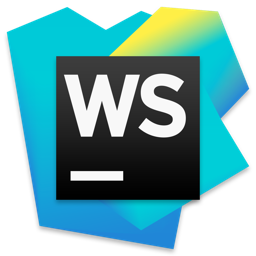
WebStorm Mac version
Useful JavaScript development tools