How to do ordered mapping using LinkedHashMap function in Java
In Java programming, sometimes it is necessary to use a sortable mapping data structure to meet the need for data to be arranged in a certain order. LinkedHashMap in Java is an ordered mapping structure, which inherits all the characteristics of HashMap and provides the additional function of remembering the insertion order. In this article, we will explain how to do ordered mapping using LinkedHashMap function in Java.
How does LinkedHashMap work?
LinkedHashMap is a Map implementation in the Java collection framework, which provides an ordered key-value pair mapping structure. Like HashMap, LinkedHashMap also inherits the Map interface and provides a Hash table to quickly retrieve and look up values in the mapping table. Unlike HashMap, LinkedHashMap not only uses a Hash table to store key-value pairs, but also uses a linked list to maintain the order of insertion. This allows the key-value pairs in the mapping table to be traversed in the order of insertion.
Implementation details of LinkedHashMap
LinkedHashMap maintains two pointers, one pointing to the head of the table and the other pointing to the tail of the table. When an element is inserted into a LinkedHashMap, it is added to the end of the linked list. When all elements of the mapping table need to be traversed, LinkedHashMap can traverse along the linked list to ensure the order of the elements.
Usage of LinkedHashMap
The usage of LinkedHashMap is similar to HashMap. It also has common methods such as put, get, remove, clear, size, etc., but it is slightly different from HashMap when traversing. The elements returned by the iterator method of LinkedHashMap are ordered, so it can be guaranteed that when traversing a LinkedHashMap, iteration is always performed in a fixed order.
First, we need to import the LinkedHashMap class:
import java.util.LinkedHashMap;
Then, we can create an empty LinkedHashMap instance to store key-value pairs:
LinkedHashMap<String, Integer> map = new LinkedHashMap<String, Integer>();
Next, we can use put Method to add key-value pairs to the map:
map.put("apple", 10); // 插入键 "apple",值为 10 map.put("banana", 20); // 插入键 "banana",值为 20 map.put("orange", 30); // 插入键 "orange",值为 30
We can use the get method to query by key:
Integer value = map.get("apple"); // 查询键 "apple" 的值,返回10
By using the keys() and values() methods, we can get the values in the map respectively The collection of all keys and all values.
Set<String> keys = map.keySet(); // 获取所有键的集合 Collection<Integer> values = map.values(); // 获取所有值的集合
When traversing LinkedHashMap, we can use the entrySet() method. This method returns a Set containing all key-value pairs of the map, where the order of the key-value pairs is in the order of insertion.
for (Map.Entry<String, Integer> entry : map.entrySet()) { System.out.println(entry.getKey() + "=>" + entry.getValue()); }
Summary:
In Java programming, LinkedHashMap is a sortable key-value pair mapping structure, which can easily meet the needs of traversal in insertion order. When using LinkedHashMap, we can use put, get, remove and clear methods to manage key-value pairs, and at the same time traverse all key-value pairs through the entrySet() method. By mastering the basic usage of LinkedHashMap, we can perform more flexible programming.
The above is the detailed content of How to do ordered mapping using LinkedHashMap function in Java. For more information, please follow other related articles on the PHP Chinese website!
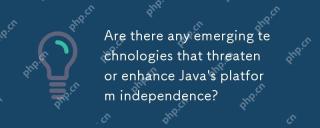
Emerging technologies pose both threats and enhancements to Java's platform independence. 1) Cloud computing and containerization technologies such as Docker enhance Java's platform independence, but need to be optimized to adapt to different cloud environments. 2) WebAssembly compiles Java code through GraalVM, extending its platform independence, but it needs to compete with other languages for performance.
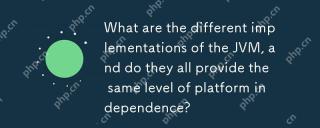
Different JVM implementations can provide platform independence, but their performance is slightly different. 1. OracleHotSpot and OpenJDKJVM perform similarly in platform independence, but OpenJDK may require additional configuration. 2. IBMJ9JVM performs optimization on specific operating systems. 3. GraalVM supports multiple languages and requires additional configuration. 4. AzulZingJVM requires specific platform adjustments.
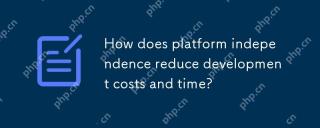
Platform independence reduces development costs and shortens development time by running the same set of code on multiple operating systems. Specifically, it is manifested as: 1. Reduce development time, only one set of code is required; 2. Reduce maintenance costs and unify the testing process; 3. Quick iteration and team collaboration to simplify the deployment process.
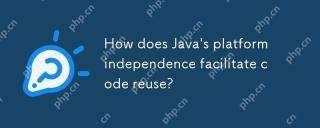
Java'splatformindependencefacilitatescodereusebyallowingbytecodetorunonanyplatformwithaJVM.1)Developerscanwritecodeonceforconsistentbehavioracrossplatforms.2)Maintenanceisreducedascodedoesn'tneedrewriting.3)Librariesandframeworkscanbesharedacrossproj
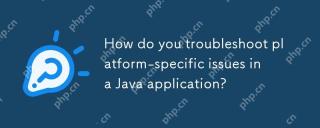
To solve platform-specific problems in Java applications, you can take the following steps: 1. Use Java's System class to view system properties to understand the running environment. 2. Use the File class or java.nio.file package to process file paths. 3. Load the local library according to operating system conditions. 4. Use VisualVM or JProfiler to optimize cross-platform performance. 5. Ensure that the test environment is consistent with the production environment through Docker containerization. 6. Use GitHubActions to perform automated testing on multiple platforms. These methods help to effectively solve platform-specific problems in Java applications.
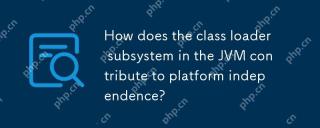
The class loader ensures the consistency and compatibility of Java programs on different platforms through unified class file format, dynamic loading, parent delegation model and platform-independent bytecode, and achieves platform independence.
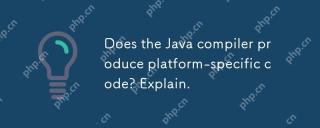
The code generated by the Java compiler is platform-independent, but the code that is ultimately executed is platform-specific. 1. Java source code is compiled into platform-independent bytecode. 2. The JVM converts bytecode into machine code for a specific platform, ensuring cross-platform operation but performance may be different.
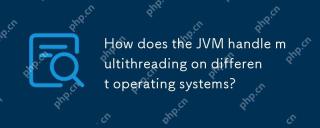
Multithreading is important in modern programming because it can improve program responsiveness and resource utilization and handle complex concurrent tasks. JVM ensures the consistency and efficiency of multithreads on different operating systems through thread mapping, scheduling mechanism and synchronization lock mechanism.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
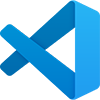
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Atom editor mac version download
The most popular open source editor

SublimeText3 Chinese version
Chinese version, very easy to use
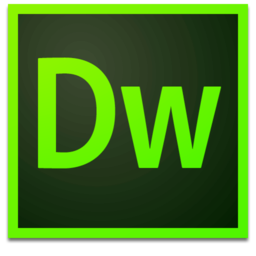
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
