This article will introduce how to use Python regular expressions for code debugging. Regular expressions are a powerful tool that can help us find and process text in our programs. When we are processing text, we may encounter some problems, such as unable to find matching content, or the matching results are incorrect. Using regular expressions can help us quickly locate problems, thereby improving the efficiency of code debugging.
1. Getting started with regular expressions
Before using regular expressions for code debugging, we need to understand the basic syntax of regular expressions. Regular expressions consist of a series of characters and special characters used to match specific text content. Here are some common regular expression metacharacters:
- . Matches any character.
- Matches the preceding character zero or more times.
- # Matches the preceding character one or more times.
- ? Matches the preceding character zero or one time.
- Matches escape characters.
- [] matches any character within square brackets.
- () is used for grouping and can be matched as a whole.
2. Use the re module for regular expression matching
Python provides the re module for regular expression matching. Here is a simple example for matching numbers in a string:
import re
s = 'abc123def456'
pattern = r'd '
result = re .findall(pattern, s)
print(result)
After running the code, the output result is ['123', '456'], indicating that the match is successful. In the above code, r'd' means matching one or more numbers, and the re.findall() function returns all matching results.
3. Use regular expressions for code debugging
Sometimes we will encounter some problems, such as being unable to find the required match when performing string operations, or the program has problems extracting data. mistake. At this time we can use regular expressions for code debugging.
1. Find and replace the content in the text
Use regular expressions to quickly find and replace the content in the text. For example, if we want to replace all numbers in the text with the letter x, we can use the following code:
import re
s = 'abc123def456'
pattern = r'd '
result = re.sub(pattern, 'x', s)
print(result)
After running the code, the output result is 'abcxdefx'. In the above code, the re.sub() function is used to replace all matching results.
2. Check whether the string format is correct
During the development process, we often need to check whether the string format is correct. For example, if we need to check whether an email address complies with the regulations, we can use the following code:
import re
email = 'test@123.com'
pattern = r'^w @ [a-zA-Z_] ?.[a-zA-Z]{2,3}$'
result = re.match(pattern, email)
if result:
print('Email address is valid')
else :
print('Email address is invalid')
After running the code, the output result is 'Email address is valid'. In the above code, r'^w @[a-zA-Z_] ?.[a-zA-Z]{2,3}$' means matching a legal email address, and the re.match() function is used to match the entire string.
3. Find and extract data from text
Sometimes we need to extract data from text and use it for other operations. For example, to extract all links in an HTML page, you can use the following code:
import re
html = '
BaiduTENcent'pattern = r'href="(1 )"'
result = re. findall(pattern, html)
for x in result:
print(x)
After running the code, the output results are 'https://www.php.cn/link/f228bda69952fa13fe74d09b34e4983b' and 'https://www .php.cn/link/154aa6866aefb6f8d0b722621fa71e83'. In the above code, r'href="(1)"' means matching the link address in the href attribute, and the re.findall() function is used to return all matching results .
Summary
When debugging Python code, using regular expressions can quickly locate problems and improve debugging efficiency. This article introduces some common regular expression syntax and usage methods, hoping to help readers solve code debugging problems.
- " ↩
The above is the detailed content of How to use Python regular expressions for code debugging. For more information, please follow other related articles on the PHP Chinese website!
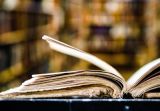
This tutorial demonstrates how to use Python to process the statistical concept of Zipf's law and demonstrates the efficiency of Python's reading and sorting large text files when processing the law. You may be wondering what the term Zipf distribution means. To understand this term, we first need to define Zipf's law. Don't worry, I'll try to simplify the instructions. Zipf's Law Zipf's law simply means: in a large natural language corpus, the most frequently occurring words appear about twice as frequently as the second frequent words, three times as the third frequent words, four times as the fourth frequent words, and so on. Let's look at an example. If you look at the Brown corpus in American English, you will notice that the most frequent word is "th
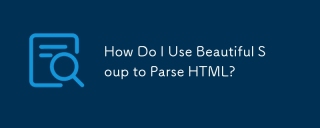
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
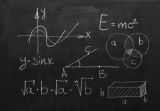
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
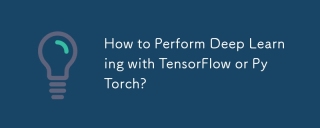
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
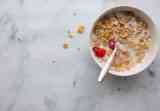
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
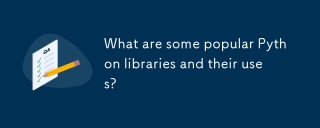
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
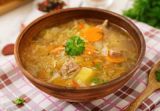
This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex
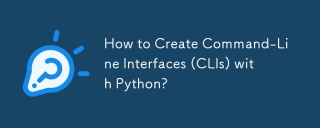
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
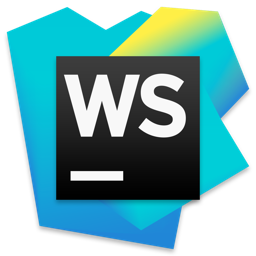
WebStorm Mac version
Useful JavaScript development tools
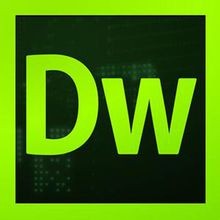
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use
