In the process of website development, it is very common to use a database to store and operate data. In order to connect to the database and perform operations, we usually use a combination of PHP and MySQL. However, for PHP, there are two different ways to use MySQL for database operations: MySQLi and PDO. In this article, we will explore how to use PDO for database operations.
PDO refers to PHP Data Object, which is an extension of PHP that helps PHP developers connect and operate databases in an object-oriented manner. PDO supports a variety of databases, and MySQL is just one of them. For developers, using PDO for database operations has the following benefits:
- PDO is object-oriented and easy to understand and use;
- PDO is more secure than MySQLi and can avoid SQL Injection attack;
- PDO is compatible with a variety of databases and can be operated using the same functions in different database systems;
- PDO provides more error handling and debugging options, which helps Developers debug and optimize code.
Let’s start with how to use PDO for database operations:
- Connecting to the database
Connecting to the database is the first step to perform database operations. We use PDO to connect to the MySQL database. To connect to the MySQL database, you need to pass the following parameters:
$host: database host address, usually localhost or 127.0.0.1
$dbname: database name
$username: username to connect to the database
$password: Password for connecting to the database
// Database connection parameters
$host = 'localhost';
$dbname = 'my_database';
$username = 'my_username';
$password = 'my_password';
// Use PDO to connect to the database
try {
$conn = new PDO("mysql:host=$host;dbname=$dbname", $username, $password);
} catch(PDOException $e) {
echo "数据库连接失败:" . $e->getMessage();
}
- Execute SQL query
PDO uses the query() method to execute SQL query statements and returns a PDOStatement object, which can use the fetch() method to extract data.
Example:
//Query all users
$sql = "SELECT * FROM users";
$stmt = $conn->query($sql);
// Output query results
while($row = $stmt->fetch()) {
echo $row['id'] . " " . $row['name'] . "
";
}
- Insert data
To insert data, you need to execute the INSERT statement. Here we use the prepare() and execute() methods to perform the insert operation.
Example:
// Insert new users
$sql = "INSERT INTO users (name, email) VALUES (:name, :email)";
$stmt = $conn->prepare($sql);
$stmt->bindParam(':name', $name);
$stmt->bindParam(':email', $email);
// Enter new user information
$name = 'amy';
$email = 'amy@example.com';
//Execute insertion
$stmt->execute();
/ / Output the insertion result
echo "The ID of the new user is: " . $conn->lastInsertId();
- Update data
To update the data, You need to execute the UPDATE statement, and also use the prepare() and execute() methods to perform the update operation.
Example:
//Update user email
$sql = "UPDATE users SET email = :email WHERE id = :id";
$stmt = $conn->prepare($sql);
$stmt->bindParam(':email', $email);
$ stmt->bindParam(':id', $id);
// Enter update information
$email = 'newemail@example.com';
$id = 1;
//Execute update
$stmt->execute();
- Delete data
To delete data, you need to execute the DELETE statement, the same Use the prepare() and execute() methods to perform deletion operations.
Example:
//Delete users
$sql = "DELETE FROM users WHERE id = :id";
$stmt = $conn->prepare($sql );
$stmt->bindParam(':id', $id);
// Enter deletion user information
$id = 1;
// Execute Delete
$stmt->execute();
Finally, we need to use the close() method to close the database connection after the database operation is completed.
Example:
//Close PDO connection
$conn = null;
Summary
In this article, we learned how to use PDO Perform database operations. PDO is a safe, convenient and highly compatible database operation method, which can improve our development efficiency and code quality in practical applications. Before performing database operations, we need to introduce PDO extensions and pass parameters for connecting to the database. After that, we can use query(), prepare(), execute() and other methods to perform SQL queries, inserts, updates and deletes. Finally, we need to close the database connection using the close() method.
The above is the detailed content of Using PDO for database operations. For more information, please follow other related articles on the PHP Chinese website!
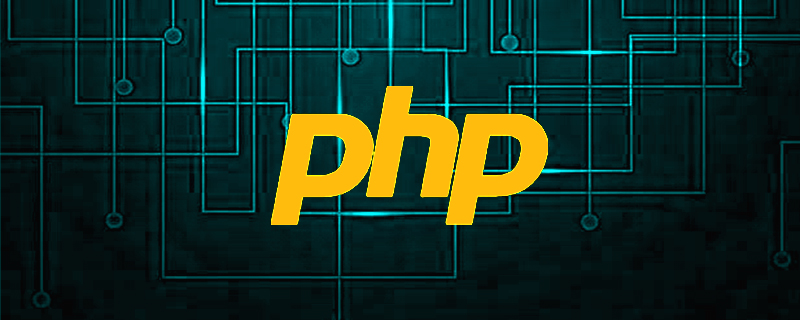
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
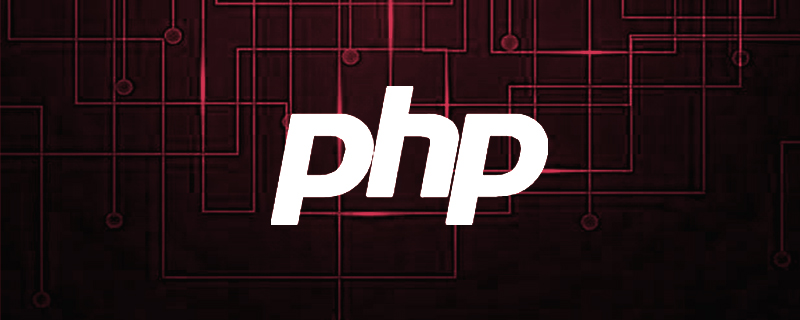
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
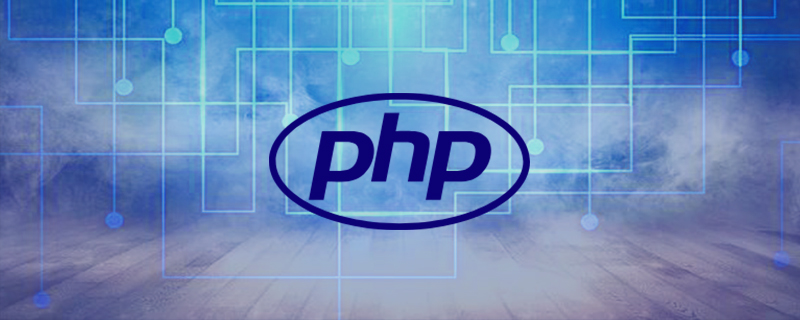
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
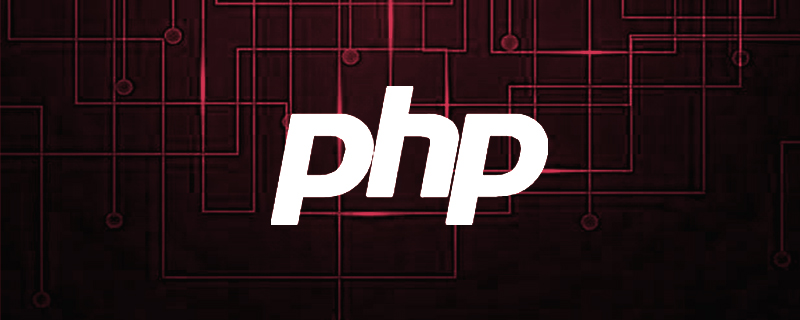
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
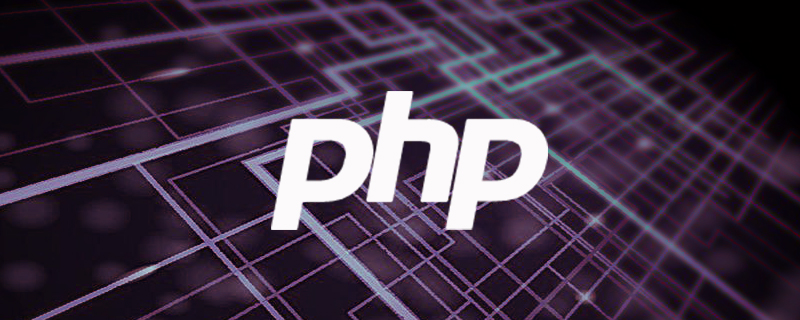
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
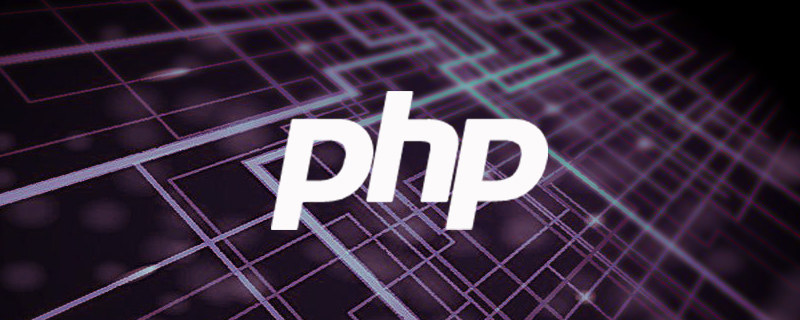
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
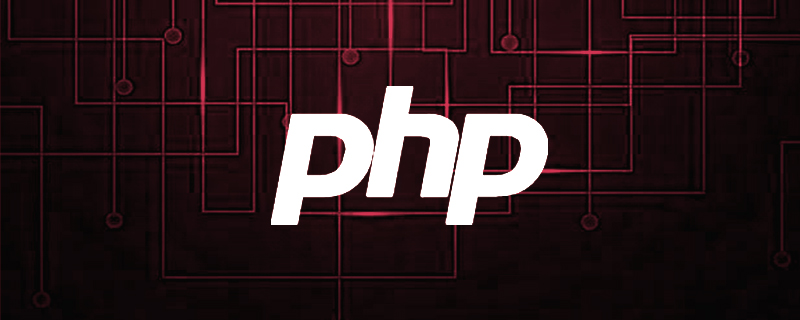
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
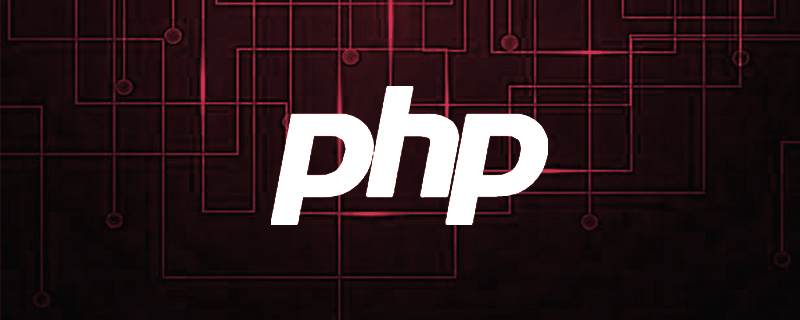
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version
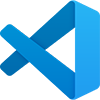
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
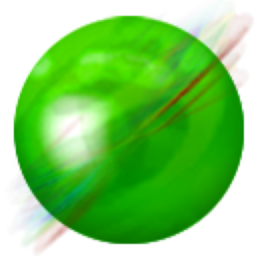
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
