Socket programming and its applications in PHP
Socket is a mechanism used for network communication and can be used to implement network-based software applications. As a widely used programming language, PHP also has important applications in network programming. Among them, Socket programming is a common way to implement network programming in PHP. Socket can be used to implement various network communication modes, such as TCP/IP and UDP.
Basic concepts of Socket programming
Socket is an abstract concept that can be understood as an interface that connects networks and applications. Socket programming is a programming method that implements the Socket interface. In fact, Socket programming can be regarded as an extension of IO operations, which can transfer data between applications. Socket programming can be performed on the same computer or between different computers.
Socket programming can realize two network communication modes: TCP/IP and UDP.
- TCP/IP mode: Also called streaming mode, data is transmitted through connections. The data transmission rate is generally slower than UDP mode, but it has better reliability. In TCP/IP mode, the establishment process of data transmission requires a "three-way handshake" process to ensure the reliability of the connection.
- UDP mode: Also called datagram mode, it uses a connectionless method for data transmission. The data transmission rate is fast, but the reliability is lower than TCP/IP mode.
Applications of Socket Programming
Through Socket programming, PHP can realize the development of many network applications, such as website interaction, email services, online games, etc. The specific applications are as follows:
1. Website interaction
Through Socket programming, data exchange between websites can be realized, as well as data interaction between the website and the client. Socket programming in PHP is usually used to implement protocols between browsers and servers, such as HTTP, HTTPS, etc. Realizing website interaction through Socket programming can enhance the scalability and controllability of the website, improve website operation efficiency and application security.
2. Mail service
Socket programming can also be used to implement mail services, including mail sending, receiving, and storage. The SMTP protocol and POP3 protocol commonly used in PHP can be implemented using Socket programming. Implementing email services through Socket programming can support more email clients, thus improving the stability and reliability of email services.
3. Online Games
Socket programming can realize the programming of online games. Through Socket programming, data interaction, resource loading and other functions between the client and the server can be realized. Because games involve real-time, interactivity, etc., and Socket programming can provide efficient and reliable network transmission, using Socket programming to implement game programming can achieve better results.
Implementation of Socket programming
There are two common ways to implement Socket programming: using the Socket library function provided by the system and using the Socket class library that comes with PHP. Here we take the use of PHP's own class library as an example to introduce the implementation of Socket programming.
1. Create a Socket connection
Use the socket_create() function in PHP to create a Socket connection, as shown below:
$socket = socket_create(AF_INET, SOCK_STREAM, SOL_TCP);
Among them, AF_INET indicates that the IPv4 network protocol is used, SOCK_STREAM indicates that the TCP/IP protocol is used, and SOL_TCP indicates that the TCP protocol type is used.
2. Bind the port and IP
After creating the Socket connection, you need to bind the port and IP so that other devices can access this service. The socket_bind() function in PHP can be used to bind the port and IP, as shown below:
socket_bind($socket, '127.0.0.1', 8080);
Among them, '127.0.0.1' represents the bound IP address, and 8080 represents the bound port number.
3. Listen for connections
After binding the port and IP, you need to listen for connection requests from other devices. You can use the socket_listen() function in PHP to implement the listening operation of Socket connections, as shown below:
socket_listen($socket, 3);
where 3 represents the set maximum number of connections .
4. Accept connection requests
When other devices request connections, you need to use the socket_accept() function in PHP to accept the connection request, as shown below:
$connection = socket_accept(socket);
Among them, $connection represents the created connection instance.
5. Sending and receiving data
After the connection is established, data can be transmitted between Socket connections. Use the socket_write() function in PHP to send data to another Socket, and use the socket_read() function to read data from another Socket.
Summary
Socket programming is a common way to implement network programming in PHP. Through Socket programming, various network communication modes can be realized, such as TCP/IP and UDP. Socket programming can realize website interaction, email service, online games and other programming. In PHP, you can use the Socket library functions provided by the system and the Socket class library that comes with PHP to implement Socket programming. Mastering Socket programming can improve the level of PHP network programming, expand the application scope of PHP, and improve development efficiency and application security.
The above is the detailed content of Socket programming and its application in PHP. For more information, please follow other related articles on the PHP Chinese website!
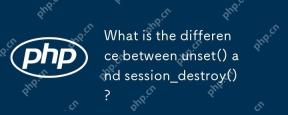
Thedifferencebetweenunset()andsession_destroy()isthatunset()clearsspecificsessionvariableswhilekeepingthesessionactive,whereassession_destroy()terminatestheentiresession.1)Useunset()toremovespecificsessionvariableswithoutaffectingthesession'soveralls
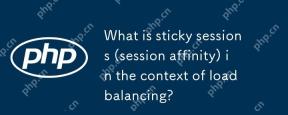
Stickysessionsensureuserrequestsareroutedtothesameserverforsessiondataconsistency.1)SessionIdentificationassignsuserstoserversusingcookiesorURLmodifications.2)ConsistentRoutingdirectssubsequentrequeststothesameserver.3)LoadBalancingdistributesnewuser
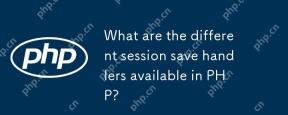
PHPoffersvarioussessionsavehandlers:1)Files:Default,simplebutmaybottleneckonhigh-trafficsites.2)Memcached:High-performance,idealforspeed-criticalapplications.3)Redis:SimilartoMemcached,withaddedpersistence.4)Databases:Offerscontrol,usefulforintegrati
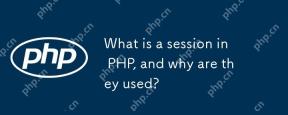
Session in PHP is a mechanism for saving user data on the server side to maintain state between multiple requests. Specifically, 1) the session is started by the session_start() function, and data is stored and read through the $_SESSION super global array; 2) the session data is stored in the server's temporary files by default, but can be optimized through database or memory storage; 3) the session can be used to realize user login status tracking and shopping cart management functions; 4) Pay attention to the secure transmission and performance optimization of the session to ensure the security and efficiency of the application.
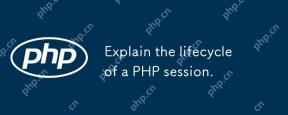
PHPsessionsstartwithsession_start(),whichgeneratesauniqueIDandcreatesaserverfile;theypersistacrossrequestsandcanbemanuallyendedwithsession_destroy().1)Sessionsbeginwhensession_start()iscalled,creatingauniqueIDandserverfile.2)Theycontinueasdataisloade
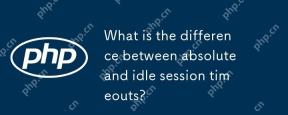
Absolute session timeout starts at the time of session creation, while an idle session timeout starts at the time of user's no operation. Absolute session timeout is suitable for scenarios where strict control of the session life cycle is required, such as financial applications; idle session timeout is suitable for applications that want users to keep their session active for a long time, such as social media.
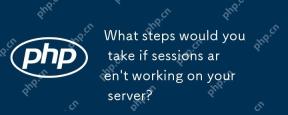
The server session failure can be solved through the following steps: 1. Check the server configuration to ensure that the session is set correctly. 2. Verify client cookies, confirm that the browser supports it and send it correctly. 3. Check session storage services, such as Redis, to ensure that they are running normally. 4. Review the application code to ensure the correct session logic. Through these steps, conversation problems can be effectively diagnosed and repaired and user experience can be improved.
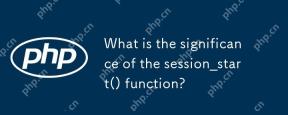
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
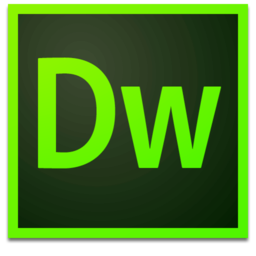
Dreamweaver Mac version
Visual web development tools
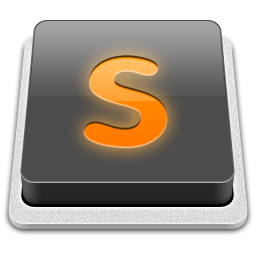
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
