


Detailed explanation of permission control and access control of Gin framework
The Gin framework is a lightweight Web framework that uses the coroutine mechanism of the Go language and an efficient routing matching algorithm to quickly process HTTP requests. At the same time, the Gin framework also provides a powerful middleware mechanism that can easily implement permission control and access control. This article will introduce the permission control and access control mechanism of the Gin framework in detail to help developers better master this function.
1. The middleware mechanism of the Gin framework
Before understanding the permission control and access control mechanisms of the Gin framework, we need to first understand the middleware mechanism of the Gin framework. Middleware refers to a mechanism that pre-processes or post-processes requests during request processing. Middleware can intercept, filter, modify and other operations on requests to achieve various functions. In the Gin framework, middleware adopts a processing method similar to the onion model. Multiple middlewares can be called in a chain to process requests multiple times. The Gin framework provides two ways of defining middleware: global middleware and local middleware.
Global middleware refers to the middleware defined before routing registration, which can process all requests. Global middleware can be defined through the Use() function, for example:
router := gin.Default() router.Use(AuthMiddleware())
This code defines a global middleware AuthMiddleware(), which will process all requests.
Partial middleware refers to middleware defined after route registration, which only processes a specific request. Local middleware can be defined through the Handlers() or Handle() function, for example:
router := gin.Default() router.GET("/users", AuthMiddleware(), ListUsersHandler())
This code defines a routing processing function for GET requests with the path "/users", and also adds a local Middleware AuthMiddleware(), this middleware only processes GET requests with the path "/users".
2. Permission control of the Gin framework
Permission control refers to authenticating the user's identity and determining whether the user has the right to perform a certain operation based on the user's identity. The Gin framework can implement permission control through the middleware mechanism. Generally speaking, permission control needs to be handled in global middleware to ensure that all requests are authenticated. The following is an example of implementing permission control:
func AuthMiddleware() gin.HandlerFunc { return func(c *gin.Context) { token := c.GetHeader("Authorization") if token == "" { c.AbortWithStatus(http.StatusUnauthorized) return } // TODO: 对Token进行验证,判断用户是否有权限 // ... c.Next() } }
This middleware first obtains the Authorization field from the request header. If the field is empty, it returns a 401 error and terminates the request processing. Then the Token is verified to determine whether the user has the authority to make the request. Finally, call the c.Next() function to continue processing the request.
3. Access control of Gin framework
Access control refers to restricting users and controlling their access to a certain resource. The Gin framework can implement access control through middleware mechanisms. Access control can take two forms: whitelist and blacklist.
Whitelist means that only certain users are allowed to access certain resources, and other users are not authorized to access. A whitelist can be defined in local middleware to process a specific request. For example:
func OnlyAdmin Middleware() gin.HandlerFunc { return func(c *gin.Context) { user := c.MustGet("user").(*model.User) if user.Role != "admin" { c.AbortWithStatus(http.StatusForbidden) return } c.Next() } } router.GET("/admin", OnlyAdmin(), AdminPageHandler())
This code defines a routing processing function for GET requests with the path "/admin", and also adds a local middleware OnlyAdmin(). This middleware only allows those with administrator roles. user access. If it is another user, return a 403 error and terminate the request processing.
Blacklist refers to prohibiting certain users from accessing certain resources, while other users can access them. The blacklist can be defined in the global middleware to process all requests. For example:
func BanlistMiddleware() gin.HandlerFunc { bannedUsers := []string{"user1", "user2", "user3"} return func(c *gin.Context) { user := c.MustGet("user").(*model.User) if contains(bannedUsers, user.Username) { c.AbortWithStatus(http.StatusForbidden) return } c.Next() } } router.Use(BanlistMiddleware())
This code defines a global middleware BanlistMiddleware(), which prohibits access by certain users. If the user is on the banned list, return a 403 error and terminate request processing. This middleware is defined before route registration and processes all requests.
4. Summary
The middleware mechanism of the Gin framework is very powerful and can easily implement different functions. In this article, we learned about the permission control and access control mechanisms of the Gin framework, which can help us better protect the security of web applications. Of course, this is only the basis of permission control and access control. In actual applications, more complex logic and security mechanisms are needed to protect the security of the system.
The above is the detailed content of Detailed explanation of permission control and access control of Gin framework. For more information, please follow other related articles on the PHP Chinese website!
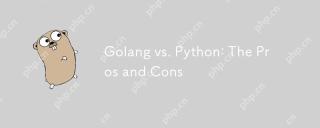
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
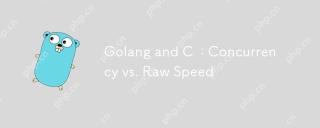
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
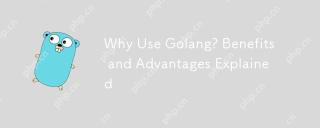
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
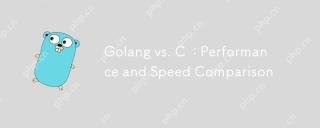
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
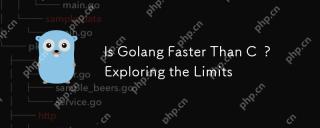
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
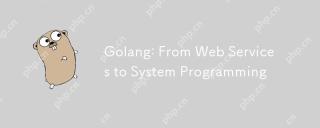
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
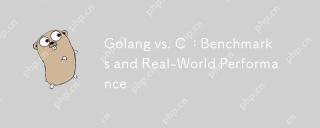
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
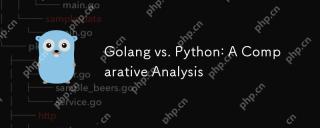
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.