When using PHP to develop web applications, you often encounter error messages such as "PHP Notice: Undefined index:". This error message is usually related to arrays.
In PHP, when we use undefined array index, we get this type of error message. This usually happens when:
- An attempt is made to access an array element that does not exist
- An attempt is made to access an array using the wrong key
In this article , we'll explore how to resolve this error and provide some common application development practices.
- Checking the Array
When you encounter the "PHP Notice: Undefined index:" error message, the first step is to check whether your array is defined. If you don't define an array, you need to add one in your code.
If you have defined an array, then you should check whether the array element you are accessing exists. You can do this like the following example:
$myarray = array("apple", "banana", "orange");
if(isset($myarray[3])) {
echo $myarray[3];
} else {
echo "Array element not found.";
}
In the above example, we first check if array element 3 is defined. If defined, prints the value of array element 3. Otherwise, print the "Array element not found" message.
- Using isset() to check array elements
In PHP, the isset() function is used to check whether a variable has been set. Returns true if the variable has been set; false otherwise.
You can use the isset() function to check whether the array element you are trying to access exists. Like the following:
$myarray = array("apple", "banana", "orange");
if(isset($myarray[3])){
echo $myarray[3];
} else {
echo "Array element not found.";
}
In the above example, we use isset() function to check whether array element 3 has been defined. If defined, prints the value of array element 3. Otherwise, print the "Array element not found" message.
- Using the array_key_exists() function
The array_key_exists() function accepts two parameters: key and array. It returns a Boolean value indicating whether the specified key exists.
You can use the array_key_exists() function to check whether the array element you are trying to access exists. Like the following:
$myarray = array("apple", "banana", "orange");
if(array_key_exists(3, $myarray)){
echo $myarray[3];
} else {
echo "Array element not found.";
}
In the above example, we use the array_key_exists() function to check whether array element 3 has been defined. If defined, prints the value of array element 3. Otherwise, print the "Array element not found" message.
To sum up, when you develop a web application in PHP, you may receive an error message like "PHP Notice: Undefined index:". If you encounter this error, first check if the array is defined. Next, you can use the isset() function or the array_key_exists() function to check if the array element you are trying to access exists. By adopting these best practices, you can avoid this error and write high-quality PHP code.
The above is the detailed content of Solution to PHP Notice: Undefined index:. For more information, please follow other related articles on the PHP Chinese website!
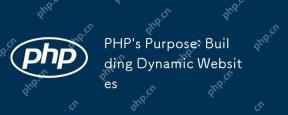
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
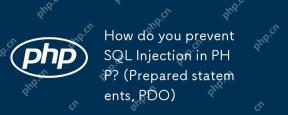
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.
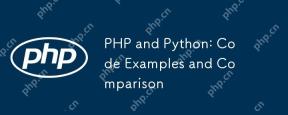
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
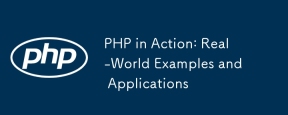
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
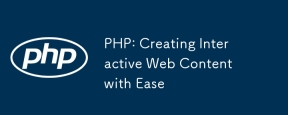
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
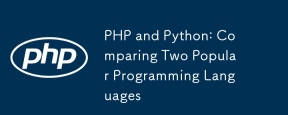
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
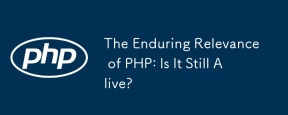
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
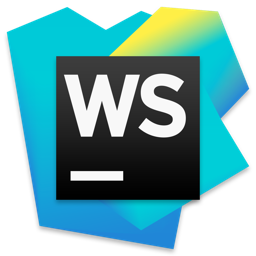
WebStorm Mac version
Useful JavaScript development tools
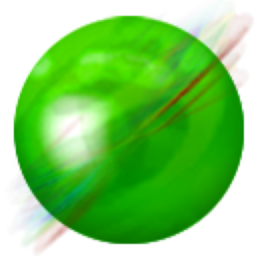
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment