Yii framework is a high-performance, open source web development framework with efficient routing and modularity mechanisms, which is very suitable for rapid development of complex web applications. Among them, sending emails is one of the inevitable functions of any application. In the Yii framework, it is very simple to implement email sending, and it also provides many customization options and rich business logic support.
1. Email configuration in Yii framework
In Yii framework, configuration files are very important resources, used to configure the basic information and various components of the application. Similarly, we can configure email through configuration files. The Yii framework supports multiple email sending methods, including SMTP server, PHP Mail, Sendmail and qmail. We can configure email by adding the following code snippet to the configuration file:
'components' => [ 'mailer' => [ 'class' => 'yiiswiftmailerMailer', 'transport' => [ 'class' => 'Swift_SmtpTransport', 'host' => 'smtp.gmail.com', 'username' => 'your-email@gmail.com', 'password' => 'your-password', 'port' => '587', 'encryption' => 'tls', ], ], ],
In the above code snippet, we are using an SMTP server for email sending, and using Gmail as the SMTP server. You can select other SMTP servers according to your needs and configure the corresponding username and password. Beyond that, there are many other options for you to choose and configure.
2. Email sending in Yii framework
Once we complete the email configuration, we can start sending emails. Sending mail is very simple, just use the shortcut Mail provided by the Yii framework, as shown below:
$mail = Yii::$app->mailer->compose(); $mail->setFrom('from@example.com') ->setTo('to@example.com') ->setSubject('Subject') ->setTextBody('Plain text content') ->setHtmlBody('<b>HTML content</b>') ->send();
In the above code, we create a Swift_Message instance and set various properties of the mail, such as sending Person, recipient, subject, email content, etc. Finally, we call the send() method to send the email.
3. Email templates in Yii framework
In actual applications, we usually do not use plain text as email content, but use customized email templates. In the Yii framework, we can use templates to generate HTML email content, for example:
$mail = Yii::$app->mailer->compose('contact-html', ['contactForm' => $form]) ->setFrom([$form->email => $form->name]) ->setTo(Yii::$app->params['adminEmail']) ->setSubject('Message from ' . $form->name) ->send();
In the above code, we call the compose() method and specify the email template file name and variables for dynamically generating HTML content of email. Template files are automatically parsed and rendered by the Yii framework, which is very convenient.
4. Mail Queue in Yii Framework
In large-scale web applications, email sending is a relatively slow and resource-consuming operation, which may reduce system performance. In order to optimize the process of sending emails, we can use the email queue function provided by the Yii framework. By adding the email sending task to the queue, we can allow the system to actually send emails when it is idle, thereby reducing the pressure on the system.
In the Yii framework, we can use the Queue plug-in of Swift Mailer to implement the mail queue. For example:
$queue = Yii::$app->queue; $queue->push(new SendEmailJob([ 'from' => 'from@example.com', 'to' => 'to@example.com', 'subject' => 'Test Subject', 'body' => 'Test text', ]));
In the above code, we call the push() method of Yii::$app->queue to add the email sending task to the queue. The queue is automatically managed by the Yii framework, which is very convenient.
Summary
Email sending is one of the inevitable functions of any web application, and the Yii framework provides very powerful and flexible email sending support. We can configure emails through configuration files, use Mail shortcuts to send emails, use email templates to generate HTML email content, and use email queues to optimize the email sending process. I believe that after mastering these skills, you will be able to better implement the email sending function and improve the performance and stability of the system.
The above is the detailed content of Email in Yii framework: Implementing email sending. For more information, please follow other related articles on the PHP Chinese website!
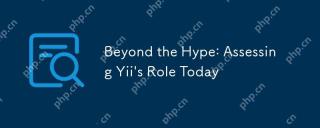
Yii remains a powerful choice for developers. 1) Yii is a high-performance PHP framework based on the MVC architecture and provides tools such as ActiveRecord, Gii and cache systems. 2) Its advantages include efficiency and flexibility, but the learning curve is steep and community support is relatively limited. 3) Suitable for projects that require high performance and flexibility, but consider the team technology stack and learning costs.
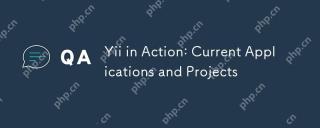
Yii framework is suitable for enterprise-level applications, small and medium-sized projects and individual projects. 1) In enterprise-level applications, Yii's high performance and scalability make it outstanding in large-scale projects such as e-commerce platforms. 2) In small and medium-sized projects, Yii's Gii tool helps quickly build prototypes and MVPs. 3) In personal and open source projects, Yii's lightweight features make it suitable for small websites and blogs.
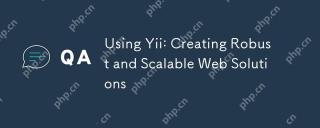
The Yii framework is suitable for building efficient, secure and scalable web applications. 1) Yii is based on the MVC architecture and provides component design and security features. 2) It supports basic CRUD operations and advanced RESTfulAPI development. 3) Provide debugging skills such as logging and debugging toolbar. 4) It is recommended to use cache and lazy loading for performance optimization.
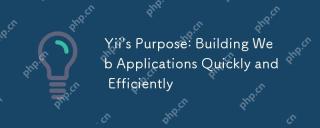
Yii's purpose is to enable developers to quickly and efficiently build web applications. Its implementation is implemented through the following methods: 1) Component-based design and MVC architecture to improve code maintainability and reusability; 2) Gii tools automatically generate code to improve development speed; 3) Lazy loading and caching mechanism optimization performance; 4) Flexible scalability to facilitate integration of third-party libraries; 5) Provide RBAC functions to handle complex business logic.
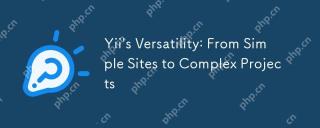
Yiiisversatileavssuitable Projectsofallsizes.1) Simple Sites, YiiOofferseassetupandrapiddevelopment.2) ForcomplexProjects, ITModularityandrbacSystemManagescalabilityandSecurity effective.
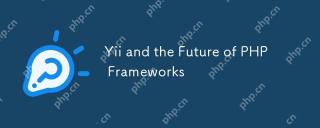
The Yii framework will continue to play an important role in the future development of PHP frameworks. 1) Yii provides efficient MVC architecture, powerful ORM system, built-in caching mechanism and rich extension libraries. 2) Its componentized design and flexibility make it suitable for complex business logic and RESTful API development. 3) Yii is constantly updated to adapt to modern PHP features and technical trends, such as microservices and containerization.
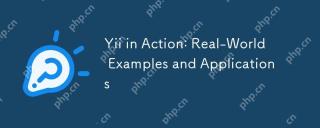
The Yii framework is suitable for developing web applications of all sizes, and its advantages lie in its high performance and rich feature set. 1) Yii adopts an MVC architecture, and its core components include ActiveRecord, Widget and Gii tools. 2) Through the request processing process, Yii efficiently handles HTTP requests. 3) Basic usage shows a simple example of creating controllers and views. 4) Advanced usage demonstrates the flexibility of database operations through ActiveRecord. 5) Debugging skills include using the debug toolbar and logging system. 6) Performance optimization It is recommended to use cache and database query optimization, follow coding specifications and dependency injection to improve code quality.
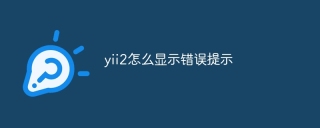
In Yii2, there are two main ways to display error prompts. One is to use Yii::$app->errorHandler->exception() to automatically catch and display errors when an exception occurs. The other is to use $this->addError(), which displays an error when model validation fails and can be accessed in the view through $model->getErrors(). In the view, you can use if ($errors = $model->getErrors())


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
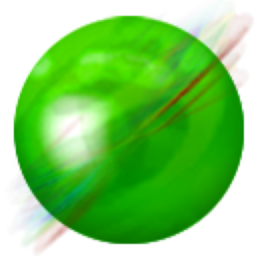
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
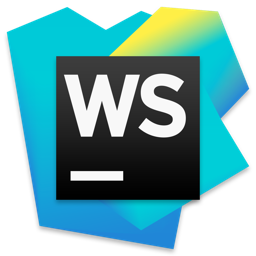
WebStorm Mac version
Useful JavaScript development tools
