


Object Relational Mapping (ORM) Basics: Understanding Doctrine ORM
Object Relational Mapping (ORM) Basics: Understanding Doctrine ORM
When we develop applications, we need to operate on the database to store and obtain data. However, it is inconvenient to use the original database query code directly. We need to establish a mapping relationship between objects and data. This is the role of ORM. ORM automatically maps and converts objects and database tables, allowing easy data manipulation, making our code easier to maintain.
Doctrine ORM is one of the most popular ORM frameworks in PHP. It uses a simple but effective method to map PHP objects and database tables, providing an easy-to-use API for CRUD operations.
This article will introduce some basic knowledge of Doctrine ORM, including configuration, entity (Entity), mapping (Mapping) and query (query), etc.
Configuration
Before we begin, we need to install Doctrine ORM. It can be installed through Composer, using the following command:
composer require doctrine/orm
Next, in our PHP file, we need to initialize Doctrine. You can pass the following code:
use DoctrineORMToolsSetup; use DoctrineORMEntityManager; require_once "vendor/autoload.php"; $paths = array("path/to/entity-files"); $isDevMode = false; // the connection configuration $dbParams = array( 'driver' => 'pdo_mysql', 'user' => 'your_database_user', 'password' => 'your_database_password', 'dbname' => 'your_database_name', ); $config = Setup::createAnnotationMetadataConfiguration($paths, $isDevMode); $entityManager = EntityManager::create($dbParams, $config);
In the above code, we first specify the path to the entity file. We then specified the database connection parameters such as driver, username, password, and database name. Finally, we use the Setup::createAnnotationMetadataConfiguration() function to configure the metadata, and then use the EntityManager::create() function to create the entity manager.
Entity
In fact, Model and Entity are the same thing. We need to create an entity class to map the database table. This class needs to inherit the DoctrineORMMappingClassMetadata class and use DoctrineORMMappingEntity and DoctrineORMMappingTable annotations.
use DoctrineORMMapping as ORM; /** * @ORMEntity * @ORMTable(name="users") */ class User { /** * @ORMId * @ORMGeneratedValue * @ORMColumn(type="integer") */ private $id; /** * @ORMColumn(type="string") */ private $name; /** * @ORMColumn(type="string", length=100, unique=true) */ private $email; // ... getters and setters }
In the above code, we have defined a User entity class that will map the database table named "users". It has three attributes: $id, $name and $email. Annotations tell Doctrine ORM how to map these properties, for example the $id property is the primary key and is auto-incremented, the $name property is mapped to a database column of type varchar, the $email property is mapped to type varchar and must be unique within the database table.
Mapping
After we define the entity, we need to tell Doctrine ORM how to map the entity to the database table. We can use XML, comments or YAML to define mapping relationships.
Here, we use annotation to define the mapping relationship. For example, in the code below, we define a mapping relationship to map the User entity to the users
database table:
/** * @ORMEntity * @ORMTable(name="users") */ class User { // properties ... // many-to-one association /** * @ORMManyToOne(targetEntity="Department") * @ORMJoinColumn(name="department_id", referencedColumnName="id") */ private $department; }
In the code above, we define a User entity with Department Many-to-one relationships between entities. All mapping relationship definitions need to be marked with annotations.
Query
Doctrine ORM provides a set of easy-to-use query APIs that allow us to easily perform CRUD operations. For example, the following code demonstrates how to query an entity using Doctrine:
$userRepository = $entityManager->getRepository('User'); $users = $userRepository->findAll(); foreach ($users as $user) { echo sprintf("-%s ", $user->getName()); }
In the above code, we use the $entityManager variable to obtain a User repository instance. We then retrieve all User instances using the findAll() method, printing the username of each instance.
Summary
This article introduces the basic knowledge of Doctrine ORM, including configuration, entities, mapping and queries. ORM is a very powerful tool that can greatly simplify the coding of database-related functions. I hope this article will help you understand ORM, and I hope you can learn more about Doctrine ORM and start using it.
The above is the detailed content of Object Relational Mapping (ORM) Basics: Understanding Doctrine ORM. For more information, please follow other related articles on the PHP Chinese website!
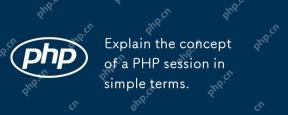
PHPsessionstrackuserdataacrossmultiplepagerequestsusingauniqueIDstoredinacookie.Here'showtomanagethemeffectively:1)Startasessionwithsession_start()andstoredatain$_SESSION.2)RegeneratethesessionIDafterloginwithsession_regenerate_id(true)topreventsessi
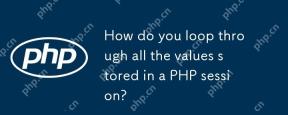
In PHP, iterating through session data can be achieved through the following steps: 1. Start the session using session_start(). 2. Iterate through foreach loop through all key-value pairs in the $_SESSION array. 3. When processing complex data structures, use is_array() or is_object() functions and use print_r() to output detailed information. 4. When optimizing traversal, paging can be used to avoid processing large amounts of data at one time. This will help you manage and use PHP session data more efficiently in your actual project.
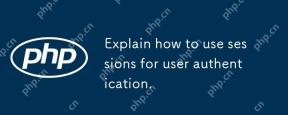
The session realizes user authentication through the server-side state management mechanism. 1) Session creation and generation of unique IDs, 2) IDs are passed through cookies, 3) Server stores and accesses session data through IDs, 4) User authentication and status management are realized, improving application security and user experience.
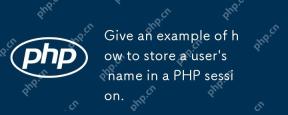
Tostoreauser'snameinaPHPsession,startthesessionwithsession_start(),thenassignthenameto$_SESSION['username'].1)Usesession_start()toinitializethesession.2)Assigntheuser'snameto$_SESSION['username'].Thisallowsyoutoaccessthenameacrossmultiplepages,enhanc
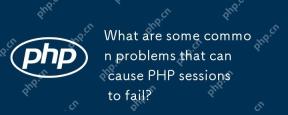
Reasons for PHPSession failure include configuration errors, cookie issues, and session expiration. 1. Configuration error: Check and set the correct session.save_path. 2.Cookie problem: Make sure the cookie is set correctly. 3.Session expires: Adjust session.gc_maxlifetime value to extend session time.
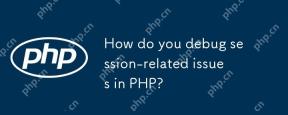
Methods to debug session problems in PHP include: 1. Check whether the session is started correctly; 2. Verify the delivery of the session ID; 3. Check the storage and reading of session data; 4. Check the server configuration. By outputting session ID and data, viewing session file content, etc., you can effectively diagnose and solve session-related problems.
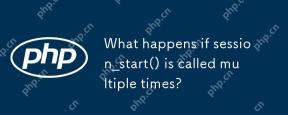
Multiple calls to session_start() will result in warning messages and possible data overwrites. 1) PHP will issue a warning, prompting that the session has been started. 2) It may cause unexpected overwriting of session data. 3) Use session_status() to check the session status to avoid repeated calls.
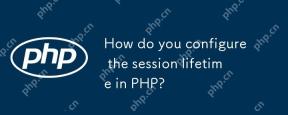
Configuring the session lifecycle in PHP can be achieved by setting session.gc_maxlifetime and session.cookie_lifetime. 1) session.gc_maxlifetime controls the survival time of server-side session data, 2) session.cookie_lifetime controls the life cycle of client cookies. When set to 0, the cookie expires when the browser is closed.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
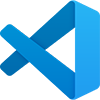
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
