


Python server programming: Optimize HTTP request response speed with Redis
With the increase in web applications and services, optimizing HTTP request response speed has become increasingly important. In most cases, using caching technology to speed up response times is an effective way. In this article, we will introduce how to use Python to write Redis server-side code to optimize HTTP request response speed.
What is Redis?
Redis is a high-performance key-value database. It supports a variety of data structures such as strings, hashes, lists, sets, sorted sets. Redis uses memory to store data and periodically persists data on disk. Because Redis uses memory to store data, it is faster than traditional relational databases.
What is WebSocket?
WebSocket is a TCP-based protocol that allows full-duplex communication over a single persistent connection. It is increasingly used in web applications, especially in real-time applications such as online games and chat applications. The WebSocket protocol differs from the HTTP protocol in that it establishes and maintains persistent connections, while the HTTP protocol is stateless.
Use Redis as a cache
In order to optimize the response speed of HTTP requests, we can use Redis as a cache. When making an HTTP request, the server first checks whether the data required for the request exists in the Redis cache. If present, the response is returned from the Redis cache without having to query the database or calculate the result again. If the required data does not exist in the Redis cache, the server performs the necessary calculations or queries and stores the data in Redis for future use.
The following is an example of Python server-side code using Redis as a cache:
import redis from flask import Flask, jsonify, request app = Flask(__name__) cache = redis.Redis(host='localhost', port=6379) def get_data_from_database(id): # Perform query to get data from database # ... # Return results return results @app.route('/api/data/<id>') def get_data(id): # Check if data exists in cache data = cache.get(id) if data: # Data found in cache, return cached data return jsonify({'data': data.decode('utf-8')}) # Data not found in cache, query database results = get_data_from_database(id) # Store results in cache cache.set(id, results) # Return results return jsonify({'data': results})
In this example, we define a get_data_from_database()
function that takes the Get data from the database and return the results. Then, we define a get_data()
function that accepts an id
parameter and looks up the data in the Redis cache. If the data is found in the Redis cache, the cached data is returned. Otherwise, we call the get_data_from_database()
function to retrieve the data, then store the data in the Redis cache and return the result to the client.
How to test Redis performance?
When using Redis as a cache, we should test the performance of Redis. Here are some ways to test Redis performance:
- Use the
redis-benchmark
tool.redis-benchmark
is a built-in Redis benchmark tool that can be used to test the performance of the Redis server. - Use a client testing tool such as
redis-cli
orredis-py
. - Simulate the workload and conduct actual testing. Testing server performance under real load is the most accurate way.
Reference code:
import time import redis # Connect to Redis redis_client = redis.Redis(host='localhost', port=6379) # Define test data test_data = {'id': '123', 'name': 'test'} # Test Redis write performance write_start_time = time.time() for i in range(1000): redis_client.set('test_data:{0}'.format(i), str(test_data)) write_end_time = time.time() print('Redis write performance (1000 iterations): {0}'.format(write_end_time-write_start_time)) # Test Redis read performance read_start_time = time.time() for i in range(1000): redis_client.get('test_data:{0}'.format(i)) read_end_time = time.time() print('Redis read performance (1000 iterations): {0}'.format(read_end_time-read_start_time))
Summary
In this article, we introduced how to use Python to write Redis server-side code to optimize HTTP request response speed. We discussed the advantages of Redis as a cache and how to test Redis performance. In actual projects, using Redis as a cache is an effective way to speed up the response speed of web applications and services.
The above is the detailed content of Python server programming: Optimize HTTP request response speed with Redis. For more information, please follow other related articles on the PHP Chinese website!
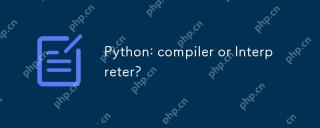
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
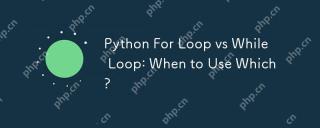
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
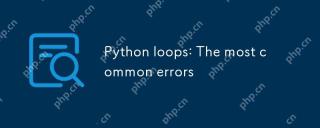
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i
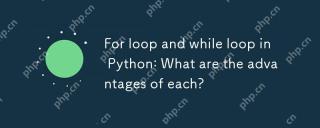
Forloopsareadvantageousforknowniterationsandsequences,offeringsimplicityandreadability;whileloopsareidealfordynamicconditionsandunknowniterations,providingcontrolovertermination.1)Forloopsareperfectforiteratingoverlists,tuples,orstrings,directlyacces
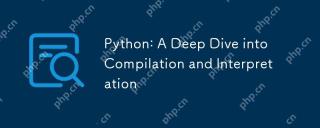
Pythonusesahybridmodelofcompilationandinterpretation:1)ThePythoninterpretercompilessourcecodeintoplatform-independentbytecode.2)ThePythonVirtualMachine(PVM)thenexecutesthisbytecode,balancingeaseofusewithperformance.
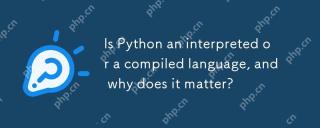
Pythonisbothinterpretedandcompiled.1)It'scompiledtobytecodeforportabilityacrossplatforms.2)Thebytecodeistheninterpreted,allowingfordynamictypingandrapiddevelopment,thoughitmaybeslowerthanfullycompiledlanguages.
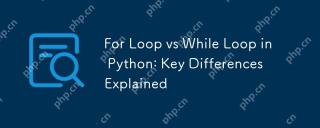
Forloopsareidealwhenyouknowthenumberofiterationsinadvance,whilewhileloopsarebetterforsituationswhereyouneedtoloopuntilaconditionismet.Forloopsaremoreefficientandreadable,suitableforiteratingoversequences,whereaswhileloopsoffermorecontrolandareusefulf
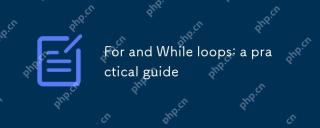
Forloopsareusedwhenthenumberofiterationsisknowninadvance,whilewhileloopsareusedwhentheiterationsdependonacondition.1)Forloopsareidealforiteratingoversequenceslikelistsorarrays.2)Whileloopsaresuitableforscenarioswheretheloopcontinuesuntilaspecificcond


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
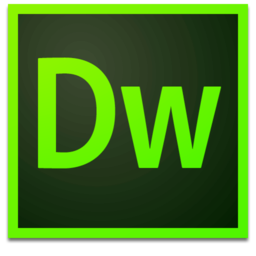
Dreamweaver Mac version
Visual web development tools
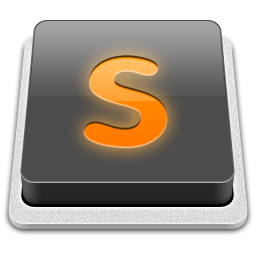
SublimeText3 Mac version
God-level code editing software (SublimeText3)
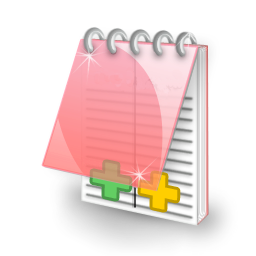
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
