


Python server programming: How to handle efficient file uploads
With the development of cloud computing and the popularization of applications, Web applications have attracted more and more attention, which has also promoted the development of server programming. File upload has always been a very important link in web application development. Especially in large websites or enterprise-level systems, file upload processing occupies a large part of the resources. Python is a very popular programming language. It has the advantages of being easy to learn, full-featured, and highly efficient in development. Especially in the field of web server programming, it has high distributed processing capabilities, so it has become the first choice of many web developers. This article will introduce how to use Python for efficient file upload processing, including how to choose a file upload method, how to handle large file uploads, how to improve file upload efficiency, etc.
1. Select the file upload method
There are generally two ways to upload files: single file upload and multiple file upload. Single file upload is suitable for users who only need to upload one file. It is generally implemented using the input tag in HTML and the type="file" attribute. Multiple file upload is suitable for situations where users need to upload multiple files. It is generally implemented using the multi-file selection box or JavaScript plug-in in HTML5.
For a single file upload, you can use Python's built-in cgi module to easily handle file upload requests. The specific code is as follows:
import cgi form = cgi.FieldStorage() fileitem = form['file'] if fileitem.filename: with open('uploaded_file.txt', 'wb') as f: f.write(fileitem.file.read()) f.close() print '上传文件 "%s" 成功!' % fileitem.filename else: print '没有选择文件!'
For multiple file uploads, you need to use a third-party library, such as flask, django, etc. Taking flask as an example, the specific code is as follows:
from flask import request @app.route('/upload', methods=['POST']) def upload(): uploaded_files = request.files.getlist("file[]") for file in uploaded_files: file.save(file.filename) return '上传文件成功!'
It should be noted that for file upload, we need to consider factors such as file type, file size, file name, etc., and need to perform certain verification and processing on the uploaded file. .
2. Processing large file uploads
For large file uploads, we need to consider some issues, such as memory limitations, server bandwidth, etc. In Python, you can use frameworks like Werkzeug or Tornado to handle large file uploads.
Take Werkzeug as an example. It provides a mechanism called "Live Upload" that can read uploaded files to the server piece by piece and save the file to the hard disk after the reading is completed. It is very simple to implement. The specific code is as follows:
from werkzeug.utils import secure_filename from werkzeug.wsgi import LimitedStream @app.route('/upload', methods=['POST']) def upload(): file = request.files['file'] filename = secure_filename(file.filename) size_limit = 1024 * 1024 * 1024 # 限制文件大小为1G stream = LimitedStream(file.stream, size_limit) with open(filename, 'wb') as f: while True: chunk = stream.read(1024 * 1024) # 每次读取1M if not chunk: break f.write(chunk) return '上传文件成功!'
This method is very useful for uploading large files, but it should be noted that only 1M of data can be read at a time, so if the uploaded file is too large, you need to Read multiple times, which may cause a decrease in transmission efficiency.
3. Improve the efficiency of file upload
To improve the efficiency of file upload, you need to consider the following aspects:
- Use the high concurrent processing capabilities of the web server, such as Use a reverse proxy server such as Nginx or Apache to send file upload requests to the backend Python server for processing.
- Set a larger buffer to use large blocks of memory to cache data in Python, reducing the number of I/Os and improving file upload efficiency.
- Compress uploaded files and reduce file size, which can significantly reduce network bandwidth consumption and improve file upload efficiency.
- Use multi-threads or multi-processes to handle file upload tasks. You can handle other tasks while uploading files, improving the system's concurrent processing capabilities.
Summary
As an efficient and easy-to-use programming language, Python can demonstrate its advantages in distributed processing capabilities in the field of Web server programming, and file upload is a Web application A very important part of the program. This article mainly introduces the two methods of file upload, the processing method of large file upload, and the method to improve the efficiency of file upload. It is hoped to help developers better handle file upload requests and improve the performance and user experience of web applications.
The above is the detailed content of Python server programming: How to handle efficient file uploads. For more information, please follow other related articles on the PHP Chinese website!
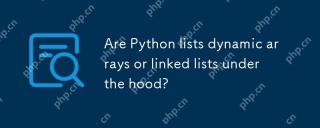
Pythonlistsareimplementedasdynamicarrays,notlinkedlists.1)Theyarestoredincontiguousmemoryblocks,whichmayrequirereallocationwhenappendingitems,impactingperformance.2)Linkedlistswouldofferefficientinsertions/deletionsbutslowerindexedaccess,leadingPytho
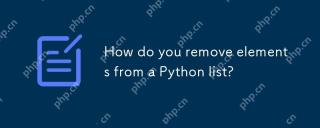
Pythonoffersfourmainmethodstoremoveelementsfromalist:1)remove(value)removesthefirstoccurrenceofavalue,2)pop(index)removesandreturnsanelementataspecifiedindex,3)delstatementremoveselementsbyindexorslice,and4)clear()removesallitemsfromthelist.Eachmetho
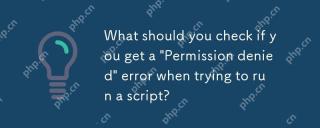
Toresolvea"Permissiondenied"errorwhenrunningascript,followthesesteps:1)Checkandadjustthescript'spermissionsusingchmod xmyscript.shtomakeitexecutable.2)Ensurethescriptislocatedinadirectorywhereyouhavewritepermissions,suchasyourhomedirectory.
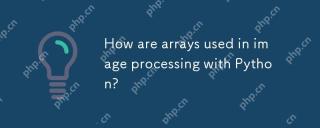
ArraysarecrucialinPythonimageprocessingastheyenableefficientmanipulationandanalysisofimagedata.1)ImagesareconvertedtoNumPyarrays,withgrayscaleimagesas2Darraysandcolorimagesas3Darrays.2)Arraysallowforvectorizedoperations,enablingfastadjustmentslikebri
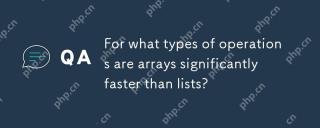
Arraysaresignificantlyfasterthanlistsforoperationsbenefitingfromdirectmemoryaccessandfixed-sizestructures.1)Accessingelements:Arraysprovideconstant-timeaccessduetocontiguousmemorystorage.2)Iteration:Arraysleveragecachelocalityforfasteriteration.3)Mem
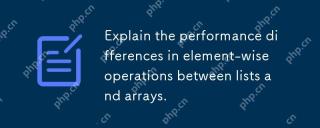
Arraysarebetterforelement-wiseoperationsduetofasteraccessandoptimizedimplementations.1)Arrayshavecontiguousmemoryfordirectaccess,enhancingperformance.2)Listsareflexiblebutslowerduetopotentialdynamicresizing.3)Forlargedatasets,arrays,especiallywithlib
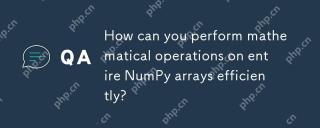
Mathematical operations of the entire array in NumPy can be efficiently implemented through vectorized operations. 1) Use simple operators such as addition (arr 2) to perform operations on arrays. 2) NumPy uses the underlying C language library, which improves the computing speed. 3) You can perform complex operations such as multiplication, division, and exponents. 4) Pay attention to broadcast operations to ensure that the array shape is compatible. 5) Using NumPy functions such as np.sum() can significantly improve performance.
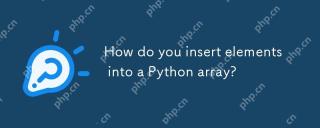
In Python, there are two main methods for inserting elements into a list: 1) Using the insert(index, value) method, you can insert elements at the specified index, but inserting at the beginning of a large list is inefficient; 2) Using the append(value) method, add elements at the end of the list, which is highly efficient. For large lists, it is recommended to use append() or consider using deque or NumPy arrays to optimize performance.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
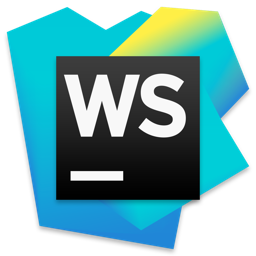
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor
