


Java backend development: Using Redisson to implement distributed API locks
With the continuous increase of Internet applications and the increasing number of users, the demand for distributed systems is getting higher and higher. In order to ensure the stability and data consistency of distributed systems, the use of locks is essential. However, in distributed systems, the implementation of locks is difficult and complex. Traditional lock implementation methods are difficult to meet the requirements of high concurrency and high availability. Therefore, this article will introduce how to use Redisson to implement distributed API locks to solve lock problems in distributed systems.
Redisson is a distributed Java object and service hosting library based on Redis implementation. It provides a simple and easy-to-use Java-based API designed to handle the special needs of high concurrency and distributed systems. Redisson supports various data structures, such as Java objects, Maps, Sets, Sorted Sets, Lists, Queues, etc. It also supports distributed locks, semaphores, counters and other functions.
The main steps to use Redisson to implement distributed locks are as follows:
1. Introduce Redisson dependencies
Before using Redisson, you need to add Redisson dependencies to the Maven or Gradle project :
<!-- Redisson --> <dependency> <groupId>org.redisson</groupId> <artifactId>redisson</artifactId> <version>3.11.1</version> </dependency>
2. Create Redisson client
Redisson client is the main object associated with the Redis server, which provides the basic method of communicating with Redis. Before creating a Redisson client, you need to configure the Redisson connection parameters:
Config config = new Config(); config.useSingleServer().setAddress("redis://127.0.0.1:6379").setDatabase(0); RedissonClient redisson = Redisson.create(config);
In the above code, we configure the connection address and database number between the client and the Redis server.
3. Obtain the distributed lock object
The distributed lock object in Redisson is the RLock interface. We can obtain the RLock object through the following code:
RLock lock = redisson.getLock("lockName");
Among them, "lockName" represents the name of the lock, and different names can be set according to different scenarios.
4. Obtain the lock and execute business logic
After obtaining the lock object, we can call the lock method to obtain the lock before executing the business logic:
lock.lock(); try { //业务逻辑代码 }finally { lock.unlock(); }
The lock method will Blocks the current thread until the lock is acquired. Generally, we also need to call the unlock method of the lock in the finally block to release the lock.
5. Other methods
Redisson provides some other methods to operate distributed lock objects, such as:
- tryLock(long waitTime, long leaseTime, TimeUnit unit): Try to acquire the lock within the specified waiting time, and set an expiration time for the lock after acquiring the lock.
- isHeldByCurrentThread(): Determine whether the current thread holds the lock.
- forceUnlock(): Forcefully release the lock.
The advantage of using Redisson to implement distributed API locks is that it can avoid single points of failure and provide high availability. At the same time, Redisson also provides a monitoring function, which can easily monitor the lock situation in the system, so as to detect and solve problems in time.
In short, the use of distributed locks is crucial to the stability and data consistency of distributed systems. Using Redisson to implement distributed API locks can greatly improve the availability and performance of the system, and is recommended.
The above is the detailed content of Java backend development: Using Redisson to implement distributed API locks. For more information, please follow other related articles on the PHP Chinese website!
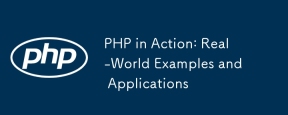
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
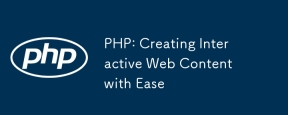
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
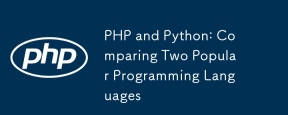
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
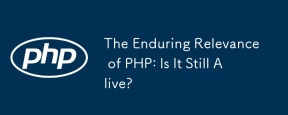
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
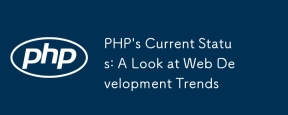
PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
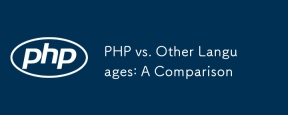
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
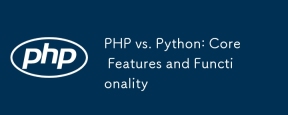
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
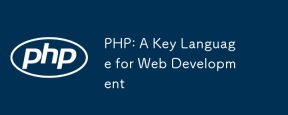
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
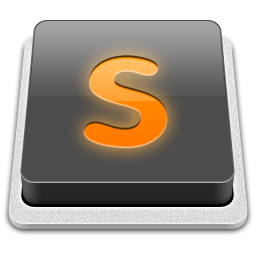
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
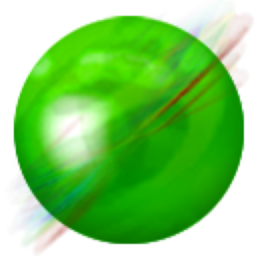
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment