


Really master the error handling skills in PHP language development
PHP is a very popular server-side programming language that is often used to develop web applications. In the development process, error handling is an essential skill. Being able to accurately identify, track, and resolve errors can greatly improve the stability and reliability of your code. This article will introduce some error handling techniques to truly master PHP language development and help developers better face errors that may occur in PHP code.
- Error handling function
PHP provides some built-in functions to detect errors, such as error_reporting(), ini_set() and set_error_handler(). Among them, set_error_handler() is a very useful function, which can customize the error handling function. Developers can use this function to capture fatal errors and non-fatal errors in PHP code and process them.
For example, the following custom error handling function records the error information to the log file:
function custom_error_handler($errno, $errstr, $errfile, $errline) { $log_file = 'error.log'; $message = date('Y-m-d H:i:s') . " - [$errno] $errstr in $errfile on line $errline "; file_put_contents($log_file, $message, FILE_APPEND); } set_error_handler('custom_error_handler');
In this function, $errno is the error level and $errstr is the error description information , $errfile is the file name where the error occurred, $errline is the number of lines where the error occurred. Through these variables, developers can record error information into log files for subsequent viewing and processing.
- Exception handling
In addition to error handling functions, PHP also provides an exception handling mechanism. Different from error handling functions, the exception handling mechanism can handle more complex error situations, such as unexpected situations when the code is running, such as array out-of-bounds, object non-existence, etc.
The exception handling mechanism in PHP mainly consists of three keywords: try, catch, and finally. Developers can use the try block to catch code blocks that may cause exceptions. If an exception is caught, the exception can be handled through the catch block; and the finally block is used to perform some necessary cleanup operations, such as releasing database connections, etc.
For example, the following sample code:
function divide($dividend, $divisor) { if ($divisor == 0) { throw new Exception('Divider cannot be zero.'); } return $dividend / $divisor; } try { $result = divide(2, 0); } catch (Exception $e) { echo 'Caught exception: ', $e->getMessage(), " "; } finally { echo 'End of program.'; }
In this code, the function divide() divides two numbers. If the divisor is 0, an exception will be thrown. In the main program, three blocks of try, catch and finally are used to handle this function that may cause exceptions. If an exception is caught, an error message will be output; if the exception is not caught, the code in the finally block will be executed normally.
- Logging
In the actual development process, the error stack may be very large, so some mechanism is needed to accurately locate the error. Logging is a very good solution to this problem.
PHP provides a very convenient built-in function error_log(), which can record error information to a specified file. Through this function, developers can arbitrarily insert error information recording code into the code to capture error information at runtime for subsequent processing.
For example, the following sample code uses the error_log() function to record error information:
function divide($dividend, $divisor) { if ($divisor == 0) { $error_message = 'Divider cannot be zero.'; error_log($error_message); return false; } return $dividend / $divisor; }
In this code, if the divisor is 0, the error information will be recorded through the error_log() function. and returns false.
- Error log monitoring
Although error logging is very useful, in actual applications, when a large number of errors occur in the system, it is obviously very difficult to manually view the logs. Therefore, error log monitoring has become an essential skill.
PHP developers can use some ready-made error monitoring tools, such as Sentry, Bugsnag, etc. These tools can automatically catch exceptions and errors in PHP code and aggregate them into a unified platform. Developers can view all error information through this platform, including error level, error description information, error location, etc. In addition, these tools also provide some convenient functions, such as reminding developers of errors through email, SMS, DingTalk, etc.
Summary
As a popular Web programming language, error handling is an important part of PHP's development. This article introduces some error handling techniques for truly mastering PHP language development, including error handling functions, exception handling, logging and error log monitoring. Through these techniques, developers can better face possible errors in PHP code and improve the reliability and stability of the code.
The above is the detailed content of Really master the error handling skills in PHP language development. For more information, please follow other related articles on the PHP Chinese website!
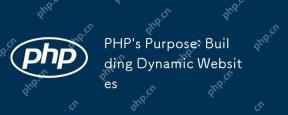
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
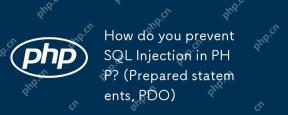
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.
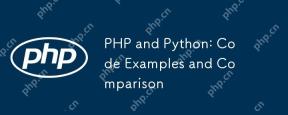
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
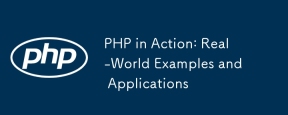
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
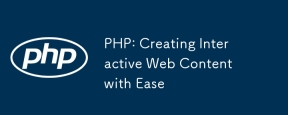
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
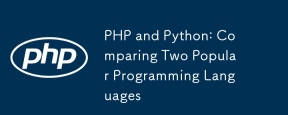
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
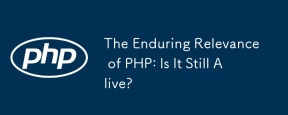
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor
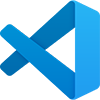
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software