Time Series Forecasting Tips in Python
With the advent of the data era, more and more data are collected and used for analysis and prediction. Time series data is a common data type that contains a series of data based on time. The methods used to forecast this type of data are called time series forecasting techniques. Python is a very popular programming language with strong data science and machine learning support, so it is also a very suitable tool for time series forecasting.
This article will introduce some commonly used time series forecasting techniques in Python and provide some examples of using them in real projects.
- Stationary time series and difference techniques
Stationary time series refers to a time series whose statistical characteristics fluctuate over time and do not change as time passes. In many cases, time series data are not stationary, meaning they have time trends and seasonal components. To convert this data into a stationary time series, we can use a differencing technique, which calculates the difference between two consecutive time points. The pandas library in Python provides functions that can be used to perform this operation.
The following is an example of using the differencing technique to convert a non-stationary time series into a stationary time series:
import pandas as pd # 读取时间序列数据 data = pd.read_csv("time_series_data.csv", header=None) # 对数据进行一阶差分 data_diff = data.diff().dropna()
- Moving average
The moving average is Refers to the method of replacing the values of the same time period in the original data with the mean value of the data in a given time period. It can be implemented using the pandas library implemented with the rolling() function. Moving averages are useful for removing noise, smoothing time series, and discovering trends and cyclical (such as seasonality) components.
Here is an example code of how to use a moving average to predict the next time series value:
import pandas as pd import numpy as np # 读取时间序列数据 data = pd.read_csv("time_series_data.csv", header=None) # 使用5个数据点进行移动平均 rolling_mean = data.rolling(window=5).mean()[5:] # 预测下一个时间步的值 last_value = data.values[-1][0] prediction = np.mean(rolling_mean) + last_value print(prediction)
- Autoregressive Moving Average (ARIMA)
Auto Regressive moving average (ARIMA) is a commonly used time series forecasting model. It is a linear model composed of an autoregressive process and a moving average process, which can be implemented using the ARIMA() function in the statamod library in Python, which allows us to specify the parameters of the stationarity and moving average of time series data.
Here is a sample code for time series forecasting using ARIMA model:
from statsmodels.tsa.arima_model import ARIMA # 读取时间序列数据 data = pd.read_csv("time_series_data.csv", header=None).values.flatten() # 训练ARIMA模型 model = ARIMA(data, order=(2, 1, 0)) model_fit = model.fit(disp=0) # 预测未来 n 个时间点的值 future_prediction = model_fit.predict(start=len(data), end=len(data)+n-1)
Summary
Python has powerful tools for time series analysis and forecasting. Among them, stationary time series and difference techniques can convert non-stationary time series into stationary time series. Moving average is a widely used smoothing technique to reduce noise and smooth time series. Autoregressive moving average (ARIMA) is a commonly used time series forecasting model that uses autoregressive and moving average.
By using these technologies, you can write independent and repeatable time series analysis and forecasting code in Python, with application scenarios including stock forecasting, weather forecasting, etc.
The above is the detailed content of Time Series Forecasting Tips in Python. For more information, please follow other related articles on the PHP Chinese website!
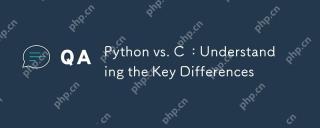
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
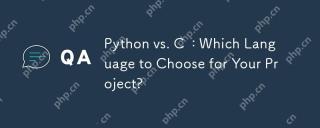
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
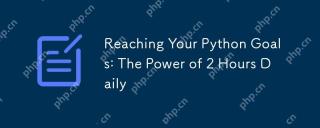
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.
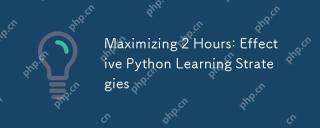
Methods to learn Python efficiently within two hours include: 1. Review the basic knowledge and ensure that you are familiar with Python installation and basic syntax; 2. Understand the core concepts of Python, such as variables, lists, functions, etc.; 3. Master basic and advanced usage by using examples; 4. Learn common errors and debugging techniques; 5. Apply performance optimization and best practices, such as using list comprehensions and following the PEP8 style guide.

Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
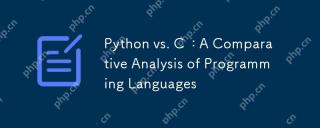
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
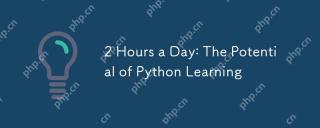
It is feasible to invest two hours a day to learn Python. 1. Learn new knowledge: Learn new concepts in one hour, such as lists and dictionaries. 2. Practice and exercises: Use one hour to perform programming exercises, such as writing small programs. Through reasonable planning and perseverance, you can master the core concepts of Python in a short time.
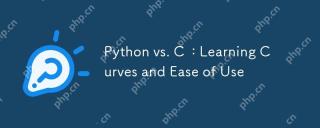
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
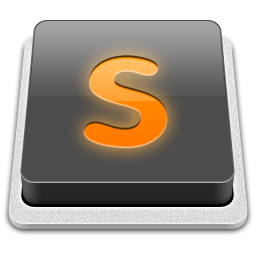
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Atom editor mac version download
The most popular open source editor
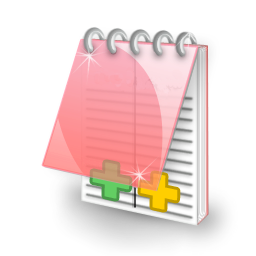
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.