How to use image sharpening techniques in Python?
Image sharpening is a commonly used image processing technique that can make pictures clearer and more detailed. In Python, we can use some common image processing libraries to implement image sharpening functions. This article will introduce how to use the Pillow library, OpenCV library and Scikit-Image library in Python for image sharpening.
- Use Pillow library for image sharpening
Pillow library is a commonly used image processing library in Python, which provides an enhanced version of PIL (Python Image Library). The Pillow library can be used to read and process various types of images, such as JPG, PNG, BMP, etc. The steps to use the Pillow library for image sharpening are as follows:
1) Install the Pillow library
Enter the following command in the command line to install the Pillow library:
pip install Pillow
2) Read Picture
Use the Image module of the Pillow library to read pictures. For example, we can read a picture named "test.jpg":
from PIL import Image image = Image.open('test.jpg')
3) Enhance the sharpness of the picture
Use the Filter module of the Pillow library to perform sharpening operations. You can use filters such as blur, edge enhancement, and sharpness enhancement. Here we use the UnsharpMask filter to enhance the sharpness of the image:
from PIL import ImageFilter sharpened_image = image.filter(ImageFilter.UnsharpMask(radius=2, percent=150, threshold=3))
In the above code, the radius parameter specifies the blur radius, the percent parameter specifies the sharpening percentage, and the threshold parameter specifies the sharpening threshold.
4) Save the result
Finally, use the save() method to save the result as a new picture:
sharpened_image.save('sharpened_test.jpg')
- Use the OpenCV library for image sharpening
The OpenCV library is an open source computer vision library that can be used for a variety of image processing tasks. The steps to use the OpenCV library for image sharpening are as follows:
1) Install the OpenCV library
Enter the following command in the command line to install the OpenCV library:
pip install opencv-python
2) Read Picture
Use the imread() function of the OpenCV library to read the picture. For example, we can read a picture named "test.jpg":
import cv2 image = cv2.imread('test.jpg')
3) Enhance the sharpness of the picture
Use the Laplacian function of the OpenCV library to enhance the sharpness of the picture. The code is as follows:
import cv2 kernel_size = 3 scale = 1 delta = 0 ddepth = cv2.CV_16S gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) gray = cv2.GaussianBlur(gray, (3, 3), 0) dst = cv2.Laplacian(gray, ddepth, ksize=kernel_size, scale=scale, delta=delta) absdst = cv2.convertScaleAbs(dst)
In the above code, the kernel_size parameter specifies the size of the operator, the scale parameter specifies the scaling factor, the delta parameter specifies the offset, and the ddepth parameter specifies the output depth.
4) Save the result
Finally, use the imwrite() function to save the result as a new picture:
cv2.imwrite('sharpened_test.jpg', absdst)
- Use the Scikit-Image library for images Sharpening
The Scikit-Image library is a Python image processing library that provides various image processing algorithms. The steps to use the Scikit-Image library for image sharpening are as follows:
1) Install the Scikit-Image library
Enter the following command on the command line to install the Scikit-Image library:
pip install scikit-image
2) Reading pictures
Use the io module of the Scikit-Image library to read pictures. For example, we can read a picture named "test.jpg":
from skimage import io image = io.imread('test.jpg')
3) Enhance the sharpness of the picture
Use the transformation module of the Scikit-Image library to perform sharpening operations . Here we use the unsharp_mask() function to enhance the sharpness of the image:
from skimage import filters sharpened_image = filters.unsharp_mask(image, radius=2, amount=1.5, multichannel=True)
In the above code, the radius parameter specifies the size of the convolution kernel, the amount parameter specifies the degree of sharpening, and the multichannel parameter specifies whether it is color. image.
4) Save the result
Finally, use the imsave() function of the io module to save the result as a new picture:
io.imsave('sharpened_test.jpg', sharpened_image)
Conclusion
This article introduces the method of image sharpening using the Pillow library, OpenCV library and Scikit-Image library in Python. These libraries provide various algorithms and functions to process images, which we can choose to use according to our needs. Image sharpening is an important part of image processing. It can improve the quality and clarity of images and has broad application prospects in practical applications.
The above is the detailed content of How to use image sharpening techniques in Python?. For more information, please follow other related articles on the PHP Chinese website!
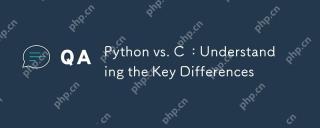
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
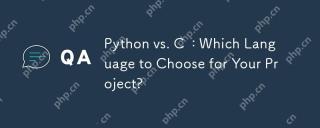
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
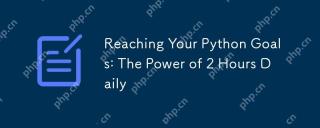
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.
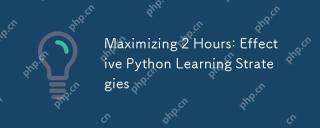
Methods to learn Python efficiently within two hours include: 1. Review the basic knowledge and ensure that you are familiar with Python installation and basic syntax; 2. Understand the core concepts of Python, such as variables, lists, functions, etc.; 3. Master basic and advanced usage by using examples; 4. Learn common errors and debugging techniques; 5. Apply performance optimization and best practices, such as using list comprehensions and following the PEP8 style guide.

Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
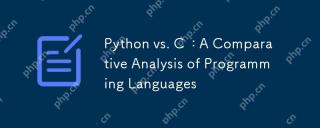
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
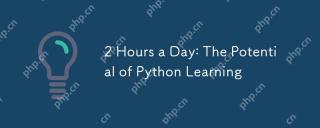
It is feasible to invest two hours a day to learn Python. 1. Learn new knowledge: Learn new concepts in one hour, such as lists and dictionaries. 2. Practice and exercises: Use one hour to perform programming exercises, such as writing small programs. Through reasonable planning and perseverance, you can master the core concepts of Python in a short time.
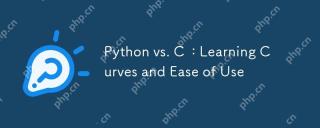
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
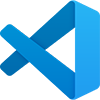
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Atom editor mac version download
The most popular open source editor

SublimeText3 English version
Recommended: Win version, supports code prompts!