Golang is a powerful programming language that is very feature-rich and suitable for various software development projects. In Golang, setting fonts is a very important task because it directly affects the display effect of the program in the user interface. This article will introduce you how to set fonts in Golang.
In Golang, there is a package called "golang.org/x/image/font", which provides a simple way to set the font. The specific steps are as follows:
一, Install font files
First, you need to download and install the font files you want to use. This can be done in various ways, such as downloading from free font libraries or purchasing from commercial font suppliers.
2. Introduction of font package
After installing the font file, you need to introduce the "golang.org/x/image/font" package into your Golang project. You can use the following command to download it in the command line:
go get golang.org/x/image/font
3. Use the font file
After introducing the font package , you need to load the font files into your Golang project. You can use the following code to load the font file into the program:
fontBytes, err := ioutil.ReadFile("path/to/your/font/file.ttf") if err != nil { log.Fatalf("read font file: %v", err) } font, err := truetype.Parse(fontBytes) if err != nil { log.Fatalf("parse font: %v", err) }
You can replace the path to the font file with the path to the font file you are actually using.
4. Set the font
After loading the font, you need to use the font to set the font and size for the text. Here is an example:
const dpi = 72 fontFace := truetype.NewFace(font, &truetype.Options{ Size: 12, DPI: dpi, Hinting: font.HintingFull, })
In the above code, we specify the font and font size by creating a truetype.NewFace object. "DPI" refers to "PPI" or "pixels per inch," which is the number of dots you see on your monitor. In this case, we set it to 72, which is the default PPI value.
In addition to the font size, you can also set the font type, such as bold, italic, etc. You can use the following code:
fontFaceBold := truetype.NewFace(font, &truetype.Options{ Size: 12, DPI: dpi, Hinting: font.HintingFull, Weight: truetype.Bold, })
In the above code, we specify that the font is bold by setting "Weight" to truetype.Bold.
5. Using Fonts
After setting and loading the font, you can use it to set the font for your output. Here is an example:
import ( "image" "image/color" "image/png" "os" "golang.org/x/image/font" "golang.org/x/image/font/basicfont" "golang.org/x/image/font/gofont/gomonobold" "golang.org/x/image/math/fixed" ) func main() { img := image.NewRGBA(image.Rect(0, 0, 400, 200)) col := color.RGBA{0xff, 0xff, 0xff, 0xff} drawString(img, "Hello, Golang!", 100, 100, fontFace, col) drawString(img, "Hello, Golang Bold!", 100, 150, fontFaceBold, col) f, err := os.Create("output.png") if err != nil { log.Fatalf("create output file: %v", err) } png.Encode(f, img) if err := f.Close(); err != nil { log.Fatalf("close file: %v", err) } } func drawString(img *image.RGBA, str string, x, y int, face font.Face, col color.Color) { d := &font.Drawer{ Dst: img, Src: image.NewUniform(col), Face: face, } d.Dot = fixed.Point26_6{ X: fixed.Int26_6(x * 64), Y: fixed.Int26_6(y * 64), } d.DrawString(str) }
In the above code snippet, we created a custom function called "drawString" to write a string to the image. In this function, we take as function parameters the image itself, the string to be written, the string position, and the font type and color to use. We then use "font.Drawer" to write the string to the image.
The final output image will contain the output of Hello, Golang! and Hello, Golang Bold! in the set font.
Summary
In this article, we introduced a simple way to set fonts in Golang. Using the "golang.org/x/image/font" package provided by Golang, you can easily set the font as well as the font size and type. Hope this article helps you better understand how to set fonts in Golang.
The above is the detailed content of golang font settings. For more information, please follow other related articles on the PHP Chinese website!
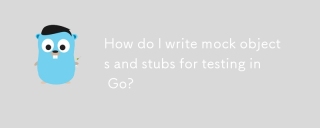
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
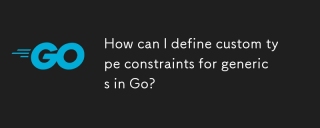
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
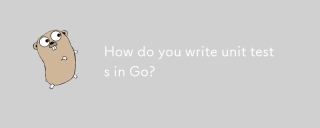
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
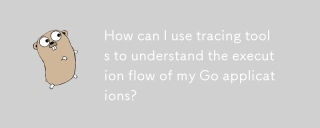
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
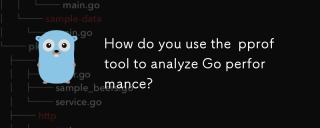
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
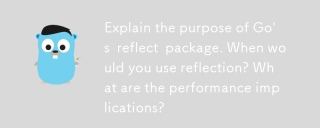
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
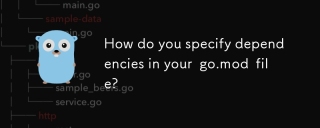
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
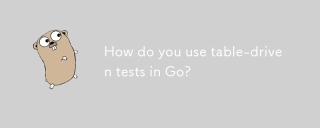
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
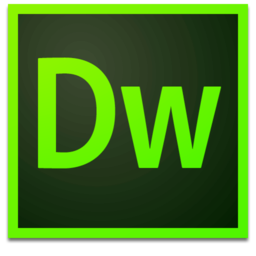
Dreamweaver Mac version
Visual web development tools
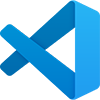
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
