PHP and Assembly are two completely different programming languages, but their respective characteristics and uses make them commonly used tools among developers. This article will introduce the basic knowledge and application scenarios of PHP and Assembly to help readers better understand these two programming languages.
1. PHP Getting Started Guide
PHP is an open source general-purpose scripting language, originally designed for dynamic web development. Now, PHP has been widely used in web development, command line scripting, desktop applications and other fields.
1. Basic syntax
PHP’s syntax is similar to C language, but there are also some special syntax and functions. The following are some basic syntax examples:
<?php // 输出Hello World echo "Hello World"; // 变量定义和赋值 $name = "PHP"; $version = 7; // 算术运算符 $result = $version * 2; // 条件语句 if ($version > 5) { echo "$name 版本大于5"; } else { echo "$name 版本小于或等于5"; } // 循环语句 for ($i = 0; $i < 5; $i++) { echo "$i "; } ?>
2. Commonly used functions
PHP has many built-in functions that can easily complete various tasks. The following are some examples of commonly used functions:
<?php // 字符串函数 echo strlen("Hello World"); // 输出11 echo strpos("Hello World", "World"); // 输出6 // 数组函数 $fruits = array("apple", "banana", "orange"); echo count($fruits); // 输出3 echo in_array("apple", $fruits); // 输出1(True) // 文件函数 $file = fopen("example.txt", "r"); echo fgets($file); // 输出文件的第一行 ?>
3. Application scenarios
PHP is one of the most popular web development languages, with a wide range of application scenarios, including:
- Dynamic web pages
- Content Management System (CMS)
- E-commerce Website
- Social Network
- Big Data Processing
- Game Server
2. Getting Started with Assembly
Assembly is a low-level programming language, mainly used for writing system software and device drivers. It uses a machine language level instruction set, so it is closer to the hardware than a high-level programming language.
1. Basic syntax
Assembly’s syntax is very simple, but it has high requirements for binary encoding. The following are some Assembly syntax examples:
section .data message db 'Hello, world!',0xa len equ $-message section .text global _start _start: ; 输出消息 mov eax,4 mov ebx,1 mov ecx,message mov edx,len int 0x80 ; 退出程序 mov eax,1 xor ebx,ebx int 0x80
2. Common instructions
Assembly uses a machine language level instruction set, which can directly operate the CPU. The following are some examples of commonly used instructions:
section .text _start: ; 算术运算指令 mov eax,5 mov ebx,7 add eax,ebx sub ebx,3 ; 分支指令 cmp eax,10 jg larger jl smaller jmp end larger: mov eax,100 jmp end smaller: mov eax,50 jmp end end: ; 退出程序 mov eax,1 xor ebx,ebx int 0x80
3. Application scenarios
Assembly is widely used in the writing of system software and device drivers due to its direct control of the underlying computer layer. The following are some Assembly application scenarios:
- Operating System Kernel
- Driver
- Embedded System
- Game Development
- Maintenance and management of large computer systems
3. Comparison and combined application of PHP and Assembly
PHP and Assembly are two very different programming languages. PHP is a high-level programming language focused on rapid development and collaboration, while Assembly is a low-level programming language focused on control and performance.
Although there are big differences in application fields, PHP and Assembly can also be used together in certain scenarios. For example, in PHP extension development, Assembly can be used to write extension modules in the underlying C language to improve the performance and efficiency of PHP applications.
In addition, in the field of high-performance computing, Assembly's instruction-level optimization can be combined with PHP's advanced features to jointly achieve a more efficient computing process.
In short, although PHP and Assembly are very different in terms of the language itself and its application scenarios, they each have their own advantages and can be used in application scenarios in different fields, or they can be combined with each other to play a greater role. role.
The above is the detailed content of Getting Started with PHP: PHP and Assembly. For more information, please follow other related articles on the PHP Chinese website!
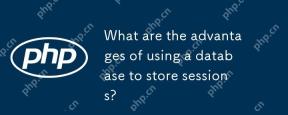
The main advantages of using database storage sessions include persistence, scalability, and security. 1. Persistence: Even if the server restarts, the session data can remain unchanged. 2. Scalability: Applicable to distributed systems, ensuring that session data is synchronized between multiple servers. 3. Security: The database provides encrypted storage to protect sensitive information.
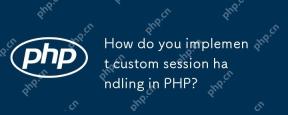
Implementing custom session processing in PHP can be done by implementing the SessionHandlerInterface interface. The specific steps include: 1) Creating a class that implements SessionHandlerInterface, such as CustomSessionHandler; 2) Rewriting methods in the interface (such as open, close, read, write, destroy, gc) to define the life cycle and storage method of session data; 3) Register a custom session processor in a PHP script and start the session. This allows data to be stored in media such as MySQL and Redis to improve performance, security and scalability.
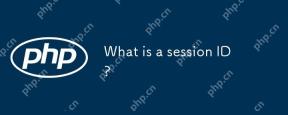
SessionID is a mechanism used in web applications to track user session status. 1. It is a randomly generated string used to maintain user's identity information during multiple interactions between the user and the server. 2. The server generates and sends it to the client through cookies or URL parameters to help identify and associate these requests in multiple requests of the user. 3. Generation usually uses random algorithms to ensure uniqueness and unpredictability. 4. In actual development, in-memory databases such as Redis can be used to store session data to improve performance and security.
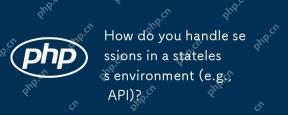
Managing sessions in stateless environments such as APIs can be achieved by using JWT or cookies. 1. JWT is suitable for statelessness and scalability, but it is large in size when it comes to big data. 2.Cookies are more traditional and easy to implement, but they need to be configured with caution to ensure security.
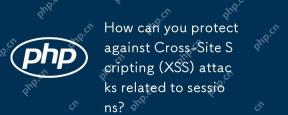
To protect the application from session-related XSS attacks, the following measures are required: 1. Set the HttpOnly and Secure flags to protect the session cookies. 2. Export codes for all user inputs. 3. Implement content security policy (CSP) to limit script sources. Through these policies, session-related XSS attacks can be effectively protected and user data can be ensured.
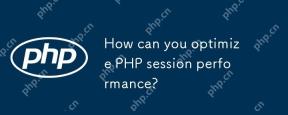
Methods to optimize PHP session performance include: 1. Delay session start, 2. Use database to store sessions, 3. Compress session data, 4. Manage session life cycle, and 5. Implement session sharing. These strategies can significantly improve the efficiency of applications in high concurrency environments.
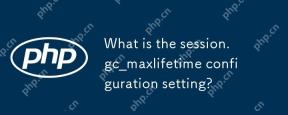
Thesession.gc_maxlifetimesettinginPHPdeterminesthelifespanofsessiondata,setinseconds.1)It'sconfiguredinphp.iniorviaini_set().2)Abalanceisneededtoavoidperformanceissuesandunexpectedlogouts.3)PHP'sgarbagecollectionisprobabilistic,influencedbygc_probabi

In PHP, you can use the session_name() function to configure the session name. The specific steps are as follows: 1. Use the session_name() function to set the session name, such as session_name("my_session"). 2. After setting the session name, call session_start() to start the session. Configuring session names can avoid session data conflicts between multiple applications and enhance security, but pay attention to the uniqueness, security, length and setting timing of session names.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
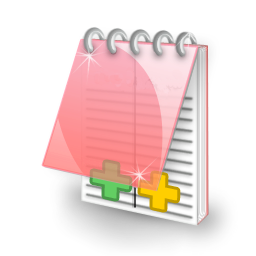
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use
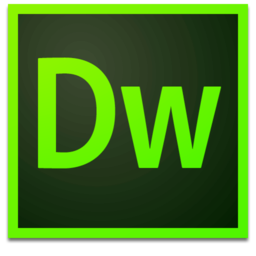
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
