In PHP programming, array is a very important data type, which can be used to store multiple variables of different types. In the process of operating an array, we may need to add/modify the key name of the array. This article will introduce how to add keys to arrays in PHP.
1. Basic syntax
In PHP, there are two ways to add elements to an array:
- Use square bracket syntax []
The sample code is as follows:
$array = []; // 初始化一个数组 $array['name'] = '张三'; $array['age'] = 20; print_r($array); // 输出数组内容
In the above code, we use square brackets [] to add key names, and any number of elements can be added to the array. Use the print_r() function to output the array content. The result is as follows:
Array ( [name] => 张三 [age] => 20 )
- Use the array_push() function
The sample code is as follows:
$array = []; array_push($array, '张三', 20); print_r($array);
In the above code , we use the array_push() function to add elements to the array. This function will add one or more elements to the end of the array. Also use the print_r() function to output, the results are as follows:
Array ( [0] => 张三 [1] => 20 )
2. Add associative array
Associative array is a special array type, and its elements use strings as key names. When adding an associative array, we need to add the key name and value to the array as a whole. The sample code is as follows:
$array = []; $array[] = array('name'=>'张三', 'age'=>20); // 添加关联数组 print_r($array); // 输出数组内容
In the above code, we use square brackets [] to add an associative array. The key name and value need to be filled in an array(), so as to ensure the corresponding relationship between the key name and value. The output result of using the print_r() function is as follows:
Array ( [0] => Array ( [name] => 张三 [age] => 20 ) )
3. Modify the key name of the array
If you want to modify the key name of the array, we can delete the original element through the unset() function, and then use Square brackets [] or the array_push() function add new elements to modify the key name. The sample code is as follows:
$array = ['name'=>'张三', 'age'=>20]; // 删除name元素 unset($array['name']); // 添加新元素 $array['username'] = 'zhangsan'; print_r($array);
In the above code, we use the unset() function to delete the original name element, and use square brackets [] to add new elements to achieve the effect of modifying the key name. The output result of using the print_r() function is as follows:
Array ( [age] => 20 [username] => zhangsan )
4. Use foreach() to loop through the array
In actual development, it is often necessary to traverse all elements of the array. PHP provides the foreach() loop to conveniently traverse arrays. The sample code is as follows:
$array = ['name'=>'张三', 'age'=>20]; foreach ($array as $key => $value) { echo $key . '=>' . $value . '<br>'; }
In the above code, we use foreach() to loop through the array and output the key names and values of the array in sequence. The output results are as follows:
name=>张三 age=>20
5. Summary
This article introduces several methods of adding key names to arrays in PHP, including using square brackets [], array_push() function, and adding associative arrays and modify the array key names. Additionally, we also covered how to use foreach() to loop through an array. By studying this article, I believe that readers have mastered common array operation skills and can become more proficient in using PHP for development.
The above is the detailed content of How to add key name to php array. For more information, please follow other related articles on the PHP Chinese website!
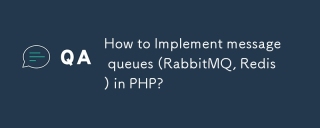
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
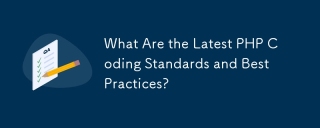
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
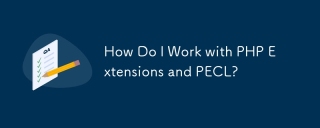
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
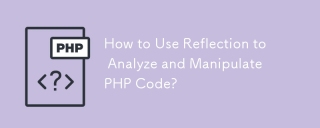
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
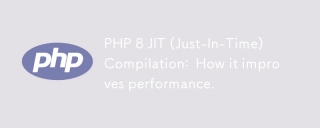
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
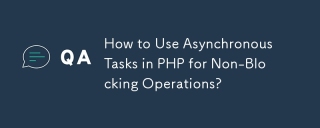
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
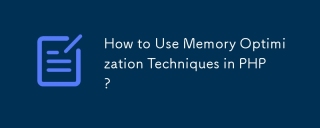
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v
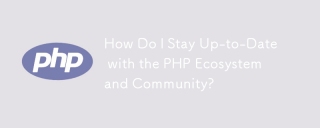
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
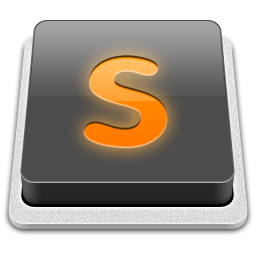
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.