In the Go language, in addition to the basic array and slice types, there is also a powerful slice type slice. Slices can be regarded as dynamic arrays, and their underlying implementation is also an array, which is flexible and efficient. When using slices, we often need to use the append method to append elements to the slice.
Memory management mechanism of Go language
Before understanding the append method, we need to first understand the memory management mechanism of Go language. In the Go language, memory is divided into two types: heap and stack. Allocating memory on the stack is generally faster than allocating memory on the heap, but stack space is limited in most cases, and allocating stack space at function call time results in additional overhead and latency.
Therefore, the memory allocator of the Go language adopts a special mechanism: when a new object needs to allocate memory, it will first try to allocate memory from the stack. If the allocation fails, the runtime library's memory allocator is called to dynamically allocate heap space. This mechanism can make the program perform better in terms of performance and be more efficient in implementation.
Slice data type in Go language
A slice is a dynamic array that can flexibly increase or decrease the number of elements. Unlike arrays, the length and capacity of slices can be modified at runtime. The following is the syntax for defining a slice:
// 声明一个slice变量a var a []int // 通过make函数创建slice a = make([]int, 5, 10) // 直接初始化slice b := []int{1, 2, 3}
In the above example, an integer slice with a capacity of 10 and a length of 5 is created. If no capacity parameter is passed in, the default capacity is equal to the length.
append method
The append method is a method built into the Go language. Its function is to append one or more elements to the end of the slice. The syntax is as follows:
append(slice []Type, elems ...Type) []Type
Among them, slice is the slice of elements to be appended, and elems is the list of elements to be appended. This method returns a new slice that contains all the elements in the original slice and the new elements.
The following is an example of using the append method:
a := []int{1, 2, 3} a = append(a, 4, 5, 6) fmt.Println(a) // [1 2 3 4 5 6]
In the above example, we define an integer slice a containing 3 elements, and append 3 elements at the end 4, 5 and 6. The final output result is [1 2 3 4 5 6].
It should be noted that when using the append method, if the capacity is insufficient, the Go language will reallocate an underlying array with a larger capacity and copy the original elements to the new array. If the capacity is sufficient, the append method will directly append elements to the end of the original underlying array.
In practice, we usually don't need to worry about the capacity of the underlying array, because the capacity of the slice has been automatically adjusted internally in the append method. However, if we need to perform some special optimizations, such as reducing memory allocation or improving program efficiency, then we need to manually adjust the capacity of the underlying array.
Pointers for operating slices
Slices are operated through pointers in the Go language. When we add elements to a slice, the underlying underlying array may be reallocated or copied, causing the underlying pointer to change. Therefore, when using slices, you must pay attention to changes in the underlying pointer.
The following is an example of a slice pointer:
a := []int{3, 4, 5} b := a[:2] // b是a的前两个元素 c := append(b, 6) fmt.Println(a) // [3 4 6] fmt.Println(b) // [3 4] fmt.Println(c) // [3 4 6]
In the above example, we define an integer slice a, and then assign the first two elements of a to another slice b. Next, we append element 6 to b and get a new slice c. Finally, we output the elements of slices a, b, and c respectively. It can be seen that the elements of slices a and b have been modified, and the new slice c contains the original slice a and the new array after appending the elements.
It should be noted that the underlying array of the slice is shared. Therefore, when we modify an element of a slice, it may affect other slices that use the same underlying array.
Summary
In the Go language, the append method is an indispensable tool when operating slices. Through the append method, we can append elements to the slice and automatically adjust the capacity of the underlying array. When using slices, pay attention to changes in the underlying pointer, and the underlying array of the slice is shared. Be careful when modifying elements.
The above is the detailed content of golang append method. For more information, please follow other related articles on the PHP Chinese website!
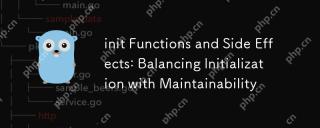
Toensureinitfunctionsareeffectiveandmaintainable:1)Minimizesideeffectsbyreturningvaluesinsteadofmodifyingglobalstate,2)Ensureidempotencytohandlemultiplecallssafely,and3)Breakdowncomplexinitializationintosmaller,focusedfunctionstoenhancemodularityandm
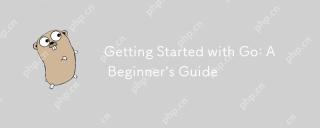
Goisidealforbeginnersandsuitableforcloudandnetworkservicesduetoitssimplicity,efficiency,andconcurrencyfeatures.1)InstallGofromtheofficialwebsiteandverifywith'goversion'.2)Createandrunyourfirstprogramwith'gorunhello.go'.3)Exploreconcurrencyusinggorout
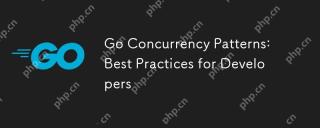
Developers should follow the following best practices: 1. Carefully manage goroutines to prevent resource leakage; 2. Use channels for synchronization, but avoid overuse; 3. Explicitly handle errors in concurrent programs; 4. Understand GOMAXPROCS to optimize performance. These practices are crucial for efficient and robust software development because they ensure effective management of resources, proper synchronization implementation, proper error handling, and performance optimization, thereby improving software efficiency and maintainability.
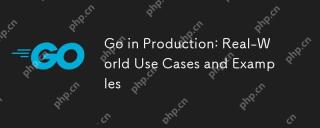
Goexcelsinproductionduetoitsperformanceandsimplicity,butrequirescarefulmanagementofscalability,errorhandling,andresources.1)DockerusesGoforefficientcontainermanagementthroughgoroutines.2)UberscalesmicroserviceswithGo,facingchallengesinservicemanageme
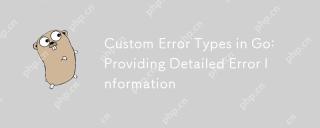
We need to customize the error type because the standard error interface provides limited information, and custom types can add more context and structured information. 1) Custom error types can contain error codes, locations, context data, etc., 2) Improve debugging efficiency and user experience, 3) But attention should be paid to its complexity and maintenance costs.
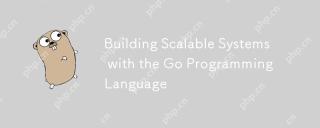
Goisidealforbuildingscalablesystemsduetoitssimplicity,efficiency,andbuilt-inconcurrencysupport.1)Go'scleansyntaxandminimalisticdesignenhanceproductivityandreduceerrors.2)Itsgoroutinesandchannelsenableefficientconcurrentprogramming,distributingworkloa
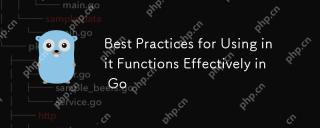
InitfunctionsinGorunautomaticallybeforemain()andareusefulforsettingupenvironmentsandinitializingvariables.Usethemforsimpletasks,avoidsideeffects,andbecautiouswithtestingandloggingtomaintaincodeclarityandtestability.
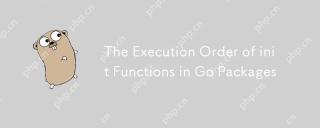
Goinitializespackagesintheordertheyareimported,thenexecutesinitfunctionswithinapackageintheirdefinitionorder,andfilenamesdeterminetheorderacrossmultiplefiles.Thisprocesscanbeinfluencedbydependenciesbetweenpackages,whichmayleadtocomplexinitializations


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
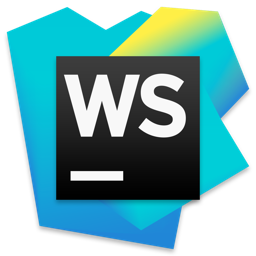
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
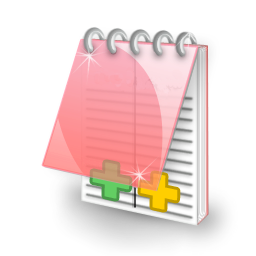
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
