


Data competition analysis of global variables and local variables of Golang functions
Golang is a strongly typed programming language with the characteristics of efficiency, simplicity, and concurrency, so it is gradually favored by more and more developers. In the development of Golang, the global variables and local variables of functions often involve data competition issues. This article will analyze the data competition problem of global variables and local variables in Golang functions from the perspective of actual coding.
1. Data competition of global variables
Golang global variables can be accessed in all functions, so if rigorous design and coding are not carried out, data competition problems are prone to occur.
For example, in the following code, we define a global variable num and increment it in two different functions:
var num int = 0 func addNum1() { for i := 0; i < 1000; i++ { num += 1 } } func addNum2() { for i := 0; i < 1000; i++ { num += 1 } }
In the above code, Both functions will increase the global variable num, which may cause data race problems. A data race occurs when two or more threads access the same shared resource simultaneously while at least one of the threads writes to the resource, resulting in undefined behavior.
The way to solve this problem is to use the Mutex type in the sync package provided by Golang. Mutex is a mutex lock. Only the thread holding the lock can access shared resources. The following is the modified code:
var num int = 0 var mutex sync.Mutex func addNum1() { for i := 0; i < 1000; i++ { mutex.Lock() num += 1 mutex.Unlock() } } func addNum2() { for i := 0; i < 1000; i++ { mutex.Lock() num += 1 mutex.Unlock() } }
In the above modified code, we implement mutually exclusive access to the global variable num through Mutex, thereby avoiding the problem of data competition.
2. Data race for local variables
Local variables are defined inside the function and can only be accessed within the function, so there are relatively few data race problems that may occur. However, there are still some issues that need to be paid attention to when using local variables.
For example, in the following code, the function getRandStr will return a random string with a length of 10:
import ( "math/rand" "time" ) func getRandStr() string { rand.Seed(time.Now().UnixNano()) baseStr := "abcdefghijklmnopqrstuvwxyz0123456789" var randBytes []byte for i := 0; i < 10; i++ { randBytes = append(randBytes, baseStr[rand.Intn(len(baseStr))]) } return string(randBytes) }
In the above code, we generated a 10-digit length through a random number a random string and use it as the return value. Such code seems to have no data competition problem, but in fact, considering that the parameters in rand.Seed(time.Now().UnixNano()) change with time, if it is called in multiple goroutines at the same time This function may cause the function to return the same result, resulting in a race problem.
In order to solve this problem, we can extract rand.Seed(time.Now().UnixNano()) outside the function and only need to call it once when the program is running. The following is the modified code:
import ( "math/rand" "time" ) func init() { rand.Seed(time.Now().UnixNano()) } func getRandStr() string { baseStr := "abcdefghijklmnopqrstuvwxyz0123456789" var randBytes []byte for i := 0; i < 10; i++ { randBytes = append(randBytes, baseStr[rand.Intn(len(baseStr))]) } return string(randBytes) }
In the above modified code, we only call rand.Seed(time.Now().UnixNano()) once through the init function to avoid multiple calls. The problem of data competition caused by calling this function at the same time in goroutine.
3. Conclusion
The above is the analysis of the data competition problem of global variables and local variables in Golang functions. To sum up, we need to follow the following principles:
- When reading and writing global variables, use a mutex lock to achieve mutually exclusive access to the global variable.
- When using local variables, please note that initialization is required when using a random number generator outside the function to avoid data competition problems caused by simultaneous access by multiple goroutines.
By following the above principles, we can avoid data race problems in Golang functions.
The above is the detailed content of Data competition analysis of global variables and local variables of Golang functions. For more information, please follow other related articles on the PHP Chinese website!
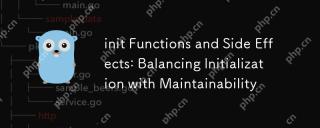
Toensureinitfunctionsareeffectiveandmaintainable:1)Minimizesideeffectsbyreturningvaluesinsteadofmodifyingglobalstate,2)Ensureidempotencytohandlemultiplecallssafely,and3)Breakdowncomplexinitializationintosmaller,focusedfunctionstoenhancemodularityandm
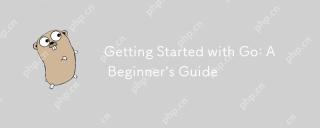
Goisidealforbeginnersandsuitableforcloudandnetworkservicesduetoitssimplicity,efficiency,andconcurrencyfeatures.1)InstallGofromtheofficialwebsiteandverifywith'goversion'.2)Createandrunyourfirstprogramwith'gorunhello.go'.3)Exploreconcurrencyusinggorout
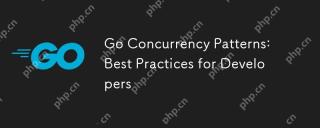
Developers should follow the following best practices: 1. Carefully manage goroutines to prevent resource leakage; 2. Use channels for synchronization, but avoid overuse; 3. Explicitly handle errors in concurrent programs; 4. Understand GOMAXPROCS to optimize performance. These practices are crucial for efficient and robust software development because they ensure effective management of resources, proper synchronization implementation, proper error handling, and performance optimization, thereby improving software efficiency and maintainability.
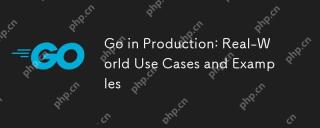
Goexcelsinproductionduetoitsperformanceandsimplicity,butrequirescarefulmanagementofscalability,errorhandling,andresources.1)DockerusesGoforefficientcontainermanagementthroughgoroutines.2)UberscalesmicroserviceswithGo,facingchallengesinservicemanageme
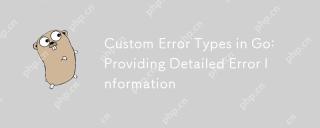
We need to customize the error type because the standard error interface provides limited information, and custom types can add more context and structured information. 1) Custom error types can contain error codes, locations, context data, etc., 2) Improve debugging efficiency and user experience, 3) But attention should be paid to its complexity and maintenance costs.
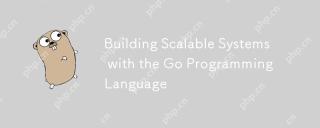
Goisidealforbuildingscalablesystemsduetoitssimplicity,efficiency,andbuilt-inconcurrencysupport.1)Go'scleansyntaxandminimalisticdesignenhanceproductivityandreduceerrors.2)Itsgoroutinesandchannelsenableefficientconcurrentprogramming,distributingworkloa
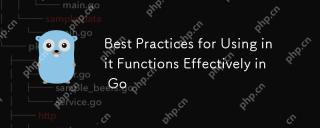
InitfunctionsinGorunautomaticallybeforemain()andareusefulforsettingupenvironmentsandinitializingvariables.Usethemforsimpletasks,avoidsideeffects,andbecautiouswithtestingandloggingtomaintaincodeclarityandtestability.
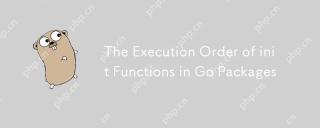
Goinitializespackagesintheordertheyareimported,thenexecutesinitfunctionswithinapackageintheirdefinitionorder,andfilenamesdeterminetheorderacrossmultiplefiles.Thisprocesscanbeinfluencedbydependenciesbetweenpackages,whichmayleadtocomplexinitializations


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
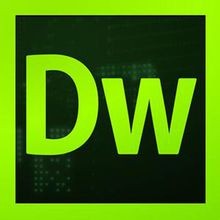
Dreamweaver CS6
Visual web development tools
