In PHP, arrays and sets are very common data structures. An array is an ordered collection that can contain different types of data, such as numbers, strings, and objects. A set is more abstract and represents a collection of elements, where each element is unique and has no specific order or index.
In actual development, we often need to convert an array into a set to facilitate more efficient searches and operations. In PHP, it is very simple to implement this conversion, and this article will introduce the implementation methods and techniques.
1. Use the array_unique function
In PHP, there is a built-in function array_unique, which can be used to remove duplicate elements in an array. The basic syntax of this function is as follows:
array array_unique ( array $array [, int $sort_flags = SORT_STRING ] )
Among them, $array represents the array that needs to be deduplicated, $sort_flags represents the optional sorting method, the common ones are SORT_STRING (sorted by string), SORT_NUMERIC (sorted by numbers) Sort by way) and SORT_REGULAR (sort in natural order), etc.
If you pass an array to the array_unique function, it will return a new array containing unique elements. For example:
$arr = array(1,2,2,3,3,3); $set = array_unique($arr); print_r($set); // 输出 Array ( [0] => 1 [1] => 2 [3] => 3 )
It can be seen that using the array_unique function can easily convert an array into a set.
2. Use the array_flip function
In addition to using the array_unique function, there is another simple way to convert an array into a collection, which is to use the array_flip function. This function is used to exchange the keys and values in the array, that is, the values in the array are used as the keys of the new array, and the original keys are ignored. The basic syntax of the function is as follows:
array array_flip ( array $array )
Among them, $array represents the array whose keys and values need to be exchanged. If you pass an array to the array_flip function, it will return a new array with the values in the original array as keys and all values in the new array. For example:
$arr = array('a', 'b', 'c'); $set = array_flip($arr); print_r($set); // 输出 Array ( [a] => 0 [b] => 1 [c] => 2 )
Since the elements in the collection must be unique, we can ignore the values in the new array and only keep its keys, that is:
$arr = array('a', 'b', 'c'); $set = array_flip($arr); $set = array_keys($set); print_r($set); // 输出 Array ( [0] => a [1] => b [2] => c )
In this way, we get a A collection containing all elements in the original array.
3. Use the array_reduce function
Another commonly used method is to use the array_reduce function. This function is used to iteratively calculate all elements in the array and return a final result. The basic syntax of the function is as follows:
mixed array_reduce ( array $array , callable $callback [, mixed $initial = NULL ] )
Among them, $array represents the array that needs to be iteratively calculated, $callback is a callback function used to calculate the elements in the array, $initial is an optional initial value, Used to specify the initial state during the iteration process. The basic syntax of the callback function is as follows:
mixed function callback ( mixed $carry , mixed $item )
Among them, $carry represents the result of the previous iteration, and $item represents the element of the current iteration. The callback function generates a new calculation result based on the last calculation result and the current element, and returns it to the array_reduce function for the next iteration.
In order to convert an array into a collection, we need to use the technique of deduplication in the callback function. Specifically, we can use the array reference technique in PHP to use the value as the key in the collection and set the value itself to 1 to ensure that the value corresponding to each key is 1, thereby ensuring that the elements in the collection are unique. The code is as follows:
$arr = array(1,2,2,3,3,3); $set = array_reduce($arr, function(&$res, $cur){ $res[$cur] = 1; return $res; }, array()); print_r(array_keys($set)); // 输出 Array ( [0] => 1 [1] => 2 [2] => 3 )
Through the processing of the above callback function, we successfully converted the original array into a collection. Of course, we can also use other set-specific operations in the callback function, such as intersection, union, difference, etc.
Summary
Converting an array to a collection is very common in actual development. PHP provides several ways to accomplish this conversion, including using the built-in functions array_unique, array_flip, and array_reduce, each with their own pros and cons. In actual scenarios, developers can choose different methods according to specific situations to ensure code efficiency and readability.
The above is the detailed content of php array to collection. For more information, please follow other related articles on the PHP Chinese website!
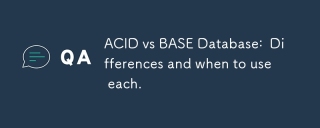
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
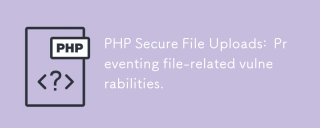
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
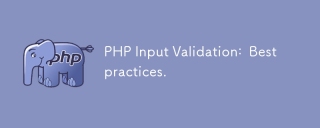
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
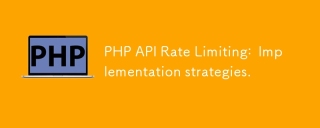
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
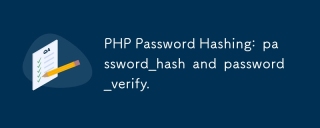
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
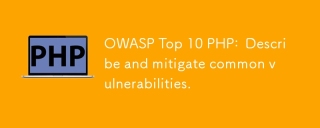
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
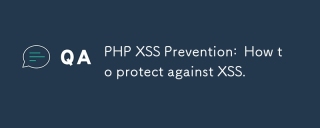
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
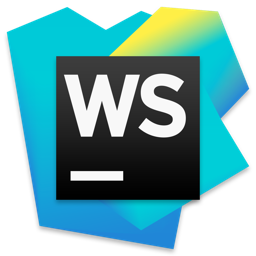
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor