JSON (JavaScript Object Notation) is a lightweight data exchange format. It is easy for humans to read and write, and also easy for computers to parse and generate. However, one limitation of JSON is that keys must be of type string. This means that working with JSON can get a little tricky when we need to pass or parse complex data structures.
In the Go language, we have a tool to solve this problem, which is to use untyped variables of type interface{}
. Using this feature we can create any structure without knowing the key names. In this article, we will explore examples of writing JSON using untyped variables in golang.
Indefinite type variables
To understand indefinite type variables, we need to first understand some basic knowledge of type conversion. In Go language, a value can be converted from one type to another through explicit and implicit type conversions. For example, we can convert a string
to int
, or a int
to float
.
An indefinite type variable is an unassigned type variable that can contain any type of value. This type of variable is very useful when you need to handle various types of data. We can use untyped variables to write JSON with undefined key names, even containing nested key-value pairs.
Creating an indefinite type variable
The easiest way to create an indefinite type variable is to use undeclared syntax. For example, the following statement will create an untyped variable named x
:
var x interface{}
At this time, the x
variable has not been assigned any type, so it can be assigned any type Value:
x = 42 // int x = "hello" // string x = true // bool
Operation of indefinite type variables
Since indefinite type variables can contain values of any type, we need to use type assertions to access their values. For example, if we know that the x
variable contains an int value, we can assign it to a new i
variable using the following type assertion:
i := x.(int)
If we don't To determine what type of value the x
variable contains, you can use type switches and the switch
statement to test its type:
switch v := x.(type) { case int: fmt.Printf("x is an int with value %v ", v) case string: fmt.Printf("x is a string with value %v ", v) default: fmt.Printf("x is of type %T ", v) }
The above code will output x
The type and value of the variable.
Limitations of indefinitely typed variables
Although indefinitely typed variables are very useful, they also have some limitations. Because untyped variables lose their stored type information, their values cannot be directly subjected to arithmetic or comparison operations. For example, the following code is incorrect:
x := 42 y := 50 z := x + y // Error: invalid operation: x + y (mismatched types interface {} and interface {})
In the above example, the compiler does not recognize the type of the x
and y
variables and therefore cannot perform the arithmetic operation. To avoid this, we can use type assertions to convert x
and y
to the corresponding types:
x := 42 y := 50 z := x.(int) + y.(int)
Writing JSON for indefinite type variables
With indefinite type variables, we can easily write JSON with indefinite key names. Let’s look at an example of using untyped variables to create a JSON with nested key-value pairs:
package main import ( "encoding/json" "fmt" ) func main() { data := make(map[string]interface{}) data["name"] = "Alice" data["age"] = 29 data["email"] = "alice@example.com" address := make(map[string]interface{}) address["city"] = "New York" address["state"] = "NY" data["address"] = address jsonString, _ := json.Marshal(data) fmt.Println(string(jsonString)) }
In the above example, we are using untyped variables to create a JSON with nested key-value pairs . First, we create an empty map and dynamically add key-value pairs using keys and values of type interface{}
. Then, we create another empty map to store nested key-value pairs. Finally, we add the nested map to the main map as a key-value pair and then encode it into a JSON string using the json.Marshal()
function.
Running the above code will output the following JSON string:
{ "address": { "city": "New York", "state": "NY" }, "age": 29, "email": "alice@example.com", "name": "Alice" }
We can see that the generated JSON string contains a nested key-value pair, which are represented in the same way is the key-value pair in the map. This example demonstrates how to use untyped variables to write complex JSON data structures without knowing the key names.
Conclusion
In this article, we learned how to write complex JSON data structures using untyped variables in Go language. To achieve this goal, we use untyped variables and maps, which allow us to easily create nested key-value pairs with arbitrary key names. Although untyped variables have some limitations, they are very convenient and useful in controlling different types of data. If you need to write JSON in Go language, using untyped variables can meet your needs.
The above is the detailed content of golang variable json. For more information, please follow other related articles on the PHP Chinese website!
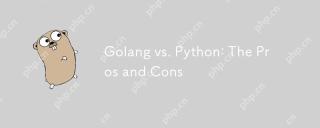
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
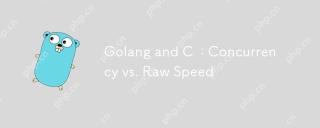
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
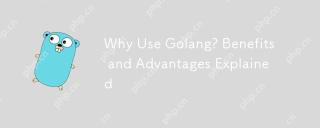
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
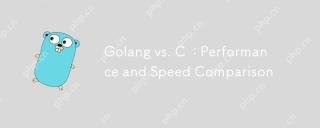
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
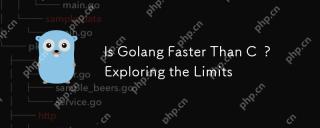
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
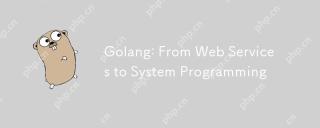
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
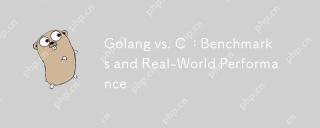
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
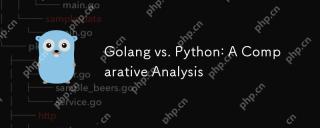
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
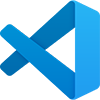
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft