More and more software systems require periodic operations, such as data backup, log cleaning, scheduled tasks, etc. When implementing these periodic operations, we usually need to use timers. Golang provides a built-in timer, but when performing timing operations, how can we stop the timer at the required time?
Generally speaking, we can use the time package provided by golang to generate a timer. For example:
timer1 := time.NewTimer(time.Second * 5) <-timer1.C fmt.Println("Timer 1 expired")
The above code will output "Timer 1 expired" after 5 seconds. The program here will receive a time signal from the timer channel (timer1.C) after waiting for 5 seconds, thereby realizing the timer's task. It should be noted that the timer created using the time.NewTimer() function will automatically repeat by default. You need to use timer.Stop() to end the timer, otherwise the timer will keep running.
But what if we need to stop the timer within a specified time? For example, when we are backing up data, if the specified backup time is exceeded, we need to forcefully stop the timer and end the backup task. At this time, we need to bind the stop signal when the timer is generated, and send abort information to the signal when it needs to be stopped.
// 定时任务函数 func doDataBackup(stopSignal chan bool){ // 模拟数据备份,并每10秒执行一遍 for { select { case <-time.After(time.Second * 10): backupData() case stop := <-stopSignal: if stop { fmt.Println("Data backup stopped.") return } } } } func main() { stopSignal := make(chan bool) // 每10秒备份一次数据,规定备份时间为50秒 go doDataBackup(stopSignal) time.Sleep(time.Second * 50) // 操纵停止信号,结束任务 stopSignal <- true }
In the above code, we added a stop signal stopSignal in the doDataBackup() function to accept forced stop information. When we need to stop the backup task, we only need to send true to the stopSignal channel, and the backup task will be forcibly stopped.
It should be noted that before sending a stop signal to the stopSignal channel, some necessary operations such as data saving and resource release may be required. These operations need to be performed before sending a stop signal to the channel, otherwise problems such as data loss may occur.
It should be emphasized that the above backup tasks are performed within a single coroutine. If we need to execute tasks regularly in multiple coroutines and need to stop them regularly, we can use golang's sync.WaitGroup and context packages to achieve this. For example:
func doTask(ctx context.Context, wg *sync.WaitGroup, id int){ defer wg.Done() fmt.Printf("goroutine %d started. ", id) for { select { case <-time.After(time.Second * 1): fmt.Printf("goroutine %d is working. ", id) case <-ctx.Done(): fmt.Printf("goroutine %d is stopped. ", id) return } } } func main() { wg := sync.WaitGroup{} ctx, cancel := context.WithTimeout(context.Background(), time.Second * 5) defer cancel() for i := 0; i < 3; i++ { wg.Add(1) go doTask(ctx, &wg, i) } wg.Wait() fmt.Println("Task completed.") }
In the above code, we created three coroutines. Each coroutine will perform a task regularly and control the closing of the coroutine through the incoming context. Use sync.WaitGroup to ensure that all coroutine tasks are completed before ending the program. Use the WithTimeout() function in the context, which stipulates that the maximum time for the task to run is 5 seconds. If the task is not completed within 5 seconds, the task will be forcibly stopped and the coroutine will exit.
The above is how to use timers in golang and how to implement scheduled stops. Using these methods, we can easily implement various periodic tasks and accurately control the running time and stop time of the tasks when needed.
The above is the detailed content of golang scheduled stop. For more information, please follow other related articles on the PHP Chinese website!
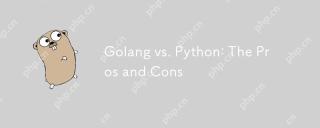
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
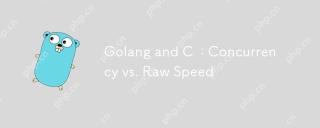
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
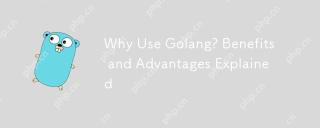
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
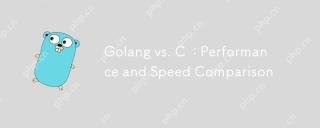
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
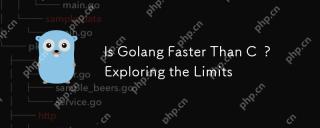
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
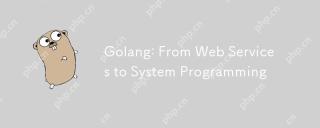
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
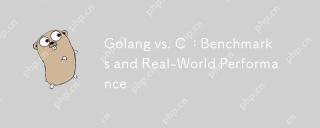
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
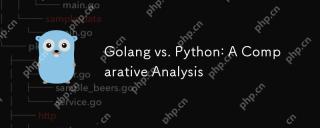
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
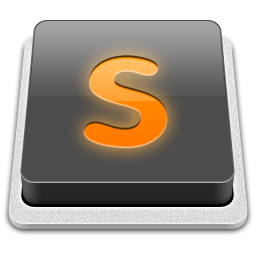
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor