In recent years, people have paid more and more attention to network security issues, and worms have also become a topic of great concern. Worms spread very quickly and can quickly infect a large number of computers, posing serious security threats to the network. To address this problem, we can use the golang language to write a simple worm to verify its propagation speed and destructive power.
First of all, we need to understand the principle of worm viruses. A worm is a self-replicating and self-propagating computer virus that usually spreads in a computer network. Each time it infects a computer, it spreads its own copies to other computers, thus achieving the propagation effect. Therefore, the core problem of worms is how to infect and spread quickly. Next, we will use golang language to complete this task.
First, we need to write a main function to start the spread of the worm virus. In this example, we will use the TCP protocol for infection. Here, we will use the local host as a starting point and send infection requests to the hosts it is connected to. The initial infection request can be passed to the program as a command line parameter, for example:
go run worm.go 192.168.1.100
In the main function, we need to obtain the parameters entered from the command line, confirm the starting point IP address, and send a Specific request to detect whether it is susceptible, the code is as follows:
func main() { if len(os.Args) < 2 { fmt.Printf("Usage: %s ip ", os.Args[0]) return } ip := os.Args[1] fmt.Printf("Starting worm from: %s ", ip) // check if target host is vulnerable if !isVulnerable(ip) { fmt.Printf("%s is not vulnerable ", ip) return } fmt.Printf("%s is vulnerable ", ip) // start worm worm(ip) }
On this basis, we can write two functions isVulnerable and worm for infection detection and propagation respectively.
The isVulnerable function is used to detect whether the IP address passed in is susceptible to infection. In this example, we assume that the target host listens to a specific TCP port and can respond to user-defined requests, so it can be determined to be susceptible to infection. Therefore, in the isVulnerable function, we need to send a predefined request to the TCP port of the target host to detect whether it is susceptible to infection. If the target host responds to the request correctly, it is judged to be susceptible to infection, otherwise it is judged to be less susceptible to infection. The specific implementation code is as follows:
func isVulnerable(ip string) bool { conn, err := net.Dial("tcp", ip+":1234") if err != nil { return false } defer conn.Close() request := "HELLO " _, err = conn.Write([]byte(request)) if err != nil { return false } response := make([]byte, 1024) n, err := conn.Read(response) if err != nil { return false } return strings.HasPrefix(string(response[:n]), "WELCOME") }
In the isVulnerable function, we use the net.Dial function to establish a TCP connection and send the custom request to the target host. If the connection is successfully established and the correct response is obtained, true is returned, otherwise false is returned.
Next, we need to write the worm function to realize the spread of the worm virus. In this example, we use a simple breadth-first search algorithm to implement propagation. The specific implementation process is as follows:
func worm(ip string) { queue := []string{ip} visited := make(map[string]bool) visited[ip] = true for len(queue) > 0 { current := queue[0] queue = queue[1:] fmt.Printf("Infecting %s ", current) // infect target host infect(current) // find new hosts to infect hosts := getHosts(current) for _, host := range hosts { if _, ok := visited[host]; !ok { queue = append(queue, host) visited[host] = true } } } }
In this function, we use a queue to save the hosts to be processed. As the program runs, the queue continues to grow, and each time the host at the front of the queue is taken out for processing infection, and at the same time add the host's uninfected neighbors to the queue to continue the infection process.
Among them, the infect function is used to infect the target host:
func infect(ip string) { conn, err := net.Dial("tcp", ip+":1234") if err != nil { return } defer conn.Close() request := "INFECTED " conn.Write([]byte(request)) }
and the getHosts function is used to obtain the uninfected neighbor list of the target host:
func getHosts(ip string) []string { hosts := []string{} for i := 1; i <= 254; i++ { current := fmt.Sprintf("%s.%d", strings.TrimSuffix(ip, ".1"), i) if isVulnerable(current) { hosts = append(hosts, current) } } return hosts }
In the getHosts function, we By traversing all IP addresses in the subnet where the current host is located, infection detection is performed one by one. If the IP address is susceptible to infection, it is added to the uninfected neighbor list and returned.
Through the combination of the above codes, we have completed the implementation of the worm virus in the golang language. Through testing and verification, we can easily find that the program spreads very quickly and is very effective. Therefore, in practical applications, we must pay attention to preventing such computer virus attacks, especially the propagation security in the network environment, to ensure the security and stability of the computer system.
The above is the detailed content of Golang implements worm virus. For more information, please follow other related articles on the PHP Chinese website!
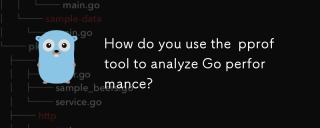
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
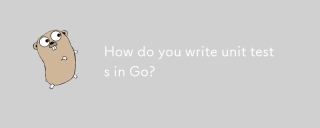
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
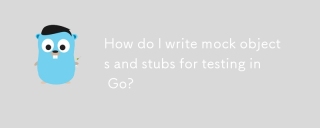
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
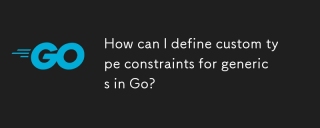
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
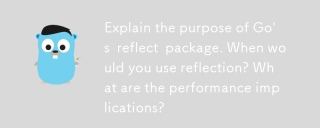
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
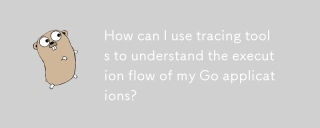
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
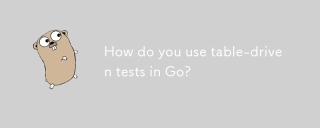
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
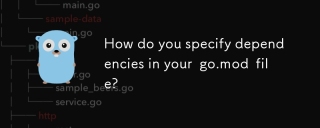
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
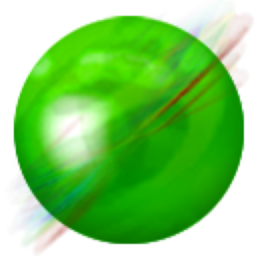
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
