Recently, while learning golang, I encountered a requirement: During the running of the program, other programs specified in the system need to be closed to ensure the correctness of the program running. In order to achieve this requirement, I conducted some research and gave the following methods.
Method 1: exec.Command
There is the exec package in golang, through which an external command can be executed, which means that other programs can be executed through the golang program. Based on this feature, we can use the exec.Command method to close other specified programs.
Code example:
cmd := exec.Command("taskkill", "/F", "/IM", "notepad.exe") err := cmd.Run() if err != nil { fmt.Println("execute command failed: ", err) }
The above code executes the taskkill command under the Windows system through the exec.Command method to close the notepad.exe program. Among them, /F means forcefully ending the process, and /IM is followed by the name of the process.
Next, we analyze the execution method of exec.Command based on the code:
- First create the exec.Cmd structure object cmd.
- Set the command and parameters to be executed (PID or process image name in Task Manager).
- Call the cmd.Run() method to execute the command.
- Handle the error err returned by cmd.Run(). If err is not nil, it means the command execution failed.
Method 2: syscall.Kill
In addition to using the exec package to execute external commands to shut down the process, golang also provides a native method - syscall.Kill. This function can directly kill the PID of the specified process.
Code example:
pid := 123 // 进程的PID err := syscall.Kill(pid, syscall.SIGKILL) // 执行kill命令 if err != nil { fmt.Println("kill process failed: ", err) }
The above code uses the syscall.Kill function to shut down the specified PID process. SIGKILL is the kill command in the Linux system, which can directly kill the process, which is equivalent to forcibly ending the process.
Next, we analyze the execution method of syscall.Kill based on the code:
- It is required to run the program with administrator privileges, otherwise the specified process will not be killed because there is no permission.
- Create the variable pid to store the PID of the process.
- Call syscall.Kill(pid, SIGKILL) to kill the process.
- Handle the error err returned by syscall.Kill. If err is not nil, it means the command execution failed.
Method 3: Use third-party libraries
In addition to the above two methods, we can also use third-party libraries to realize the function of closing other programs. The most commonly used one is the github.com/mitchellh/go-ps library, which can return information about all processes currently running in the system.
We can find the process with the specified name and kill it based on the process information.
Code example:
processes, err := ps.Processes() if err != nil { fmt.Println("get processes failed: ", err) return } for _, p := range processes { if strings.Contains(p.Executable(), "notepad") { err := syscall.Kill(p.Pid(), syscall.SIGTERM) if err != nil { fmt.Println("kill process failed: ", err) } } }
The above code obtains all process information in the system through the third-party library github.com/mitchellh/go-ps, and then traverses these processes to find names containing notepad process and kill it.
Next, let’s analyze how to use the go-ps library based on the code:
- Introduce the github.com/mitchellh/go-ps library.
- Call the ps.Processes() method to obtain all process information in the system.
- Traverse the process information and find the process with the specified name.
- Call the syscall.Kill(p.Pid(), SIGTERM) method to kill the process.
- Handle the error err returned by syscall.Kill. If err is not nil, it means the command execution failed.
Among the above three methods, exec.Command and syscall.Kill are native golang methods and are relatively simple to use. The method of using third-party libraries requires the introduction of external libraries, but you can obtain more rich information, such as process startup time, etc.
Summary
Through the above three methods, we can realize the function of golang program to close other programs. When we need to ensure the correctness of program operation, we can kill unnecessary processes in the system in this way to ensure the stability of the program. It should be noted that when using these methods, pay special attention to the PID and name of the process to avoid accidentally deleting other processes.
The above is the detailed content of golang close other programs. For more information, please follow other related articles on the PHP Chinese website!
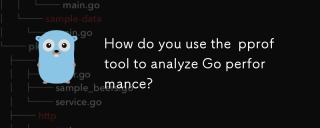
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
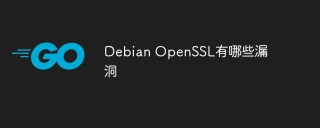
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
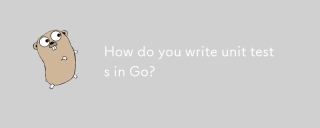
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
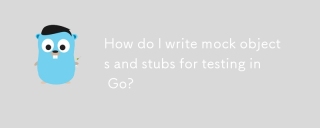
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
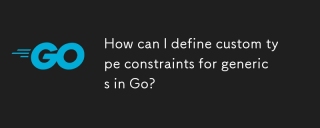
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
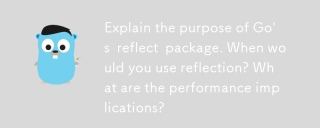
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
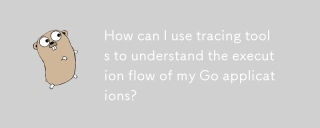
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
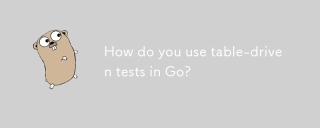
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
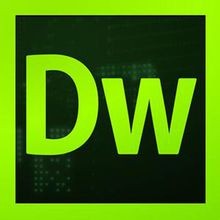
Dreamweaver CS6
Visual web development tools
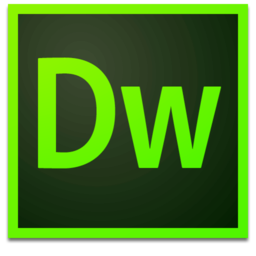
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.