How to use CGI in Go?
Using CGI in Go is a common web development technology. This article will introduce how to use CGI in Go to implement web applications.
What is CGI?
CGI stands for Common Gateway Interface, which is a standard protocol for interaction between web servers and other applications. With CGI, a web server can send requests to other applications, then receive their responses and send them back to the client. CGI is a very flexible and scalable technology that can be used to create various types of web applications.
Using CGI in Go
Using CGI in Go is similar to other programming languages. First, you need to create a CGI script to handle web requests. Then, configure the path of the CGI execution script in the web server. Finally, the web request is sent into the CGI script.
Create CGI scripts
In Go, you can use the "net/http/cgi" package in the standard library to write CGI scripts. The package contains a function called "ServeCGI" which accepts two parameters: a "cmd" string representing the command of the CGI script to be executed, and a variable of type http.ResponseWriter representing the response written to the client end. Here is a simple CGI script example:
package main import ( "fmt" "net/http" "net/http/cgi" ) func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { cgi.Handler{ Path: "/path/to/cgi/script.cgi", Dir: "/path/to/cgi/directory", Env: []string{}, }.ServeHTTP(w, r) }) fmt.Println("Listening on :8080...") http.ListenAndServe(":8080", nil) }
In the above example, we created a "/" route that sends web requests to the specified CGI script. Note that in the Handler structure you need to specify the path and directory of the CGI script, as well as environment variables (if required).
Configuring the Web Server
To configure the CGI script in the web server, you need to edit the configuration file of the web server and add the following line:
ScriptAlias /cgi-bin/ /path/to/cgi/directory/
In the above example, We map the "/cgi-bin/" path to the directory where the CGI script is located. Then, send the web request to the "/cgi-bin/script.cgi" path, and the web server will automatically execute the CGI script.
Send Web Request
Now, we are ready to use CGI in Go. To send a web request, visit "http://localhost:8080/" in your browser and the web server will automatically send the request to the CGI script.
Summary
CGI is a common web development technology that can be used to create various types of web applications. In Go, you can use the "net/http/cgi" package from the standard library to write CGI scripts. You can easily use CGI to write web applications in Go by creating a CGI script and configuring the path for the CGI execution script in the web server.
The above is the detailed content of How to use CGI in Go?. For more information, please follow other related articles on the PHP Chinese website!
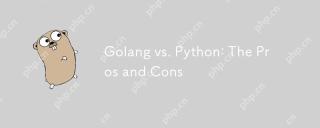
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
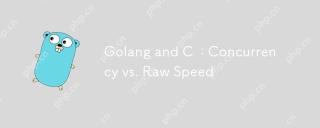
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
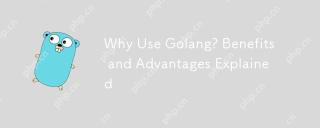
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
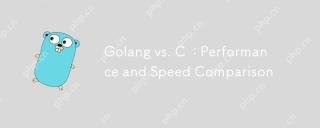
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
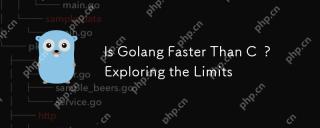
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
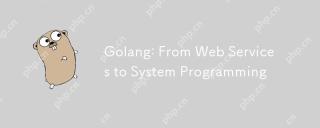
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
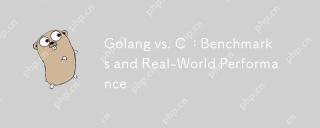
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
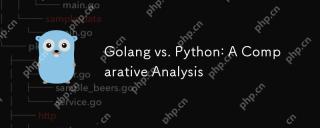
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
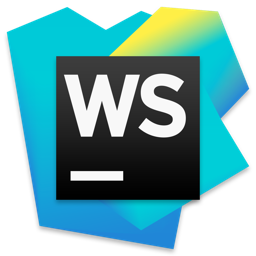
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
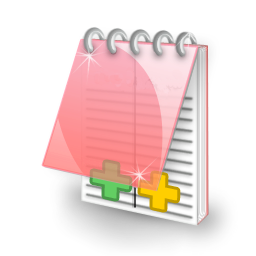
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software