With the development of Internet technology, there are increasingly higher requirements for efficient multi-tasking. In the Go language, coroutines are a very important feature that can solve this problem very well. This article will introduce how to use coroutines to implement concurrent programming in Go.
1. What is a coroutine?
A coroutine is a lightweight thread, also known as a user-mode thread. Compared with traditional multi-threaded programming, the advantages of coroutines are that they are more lightweight, occupy less system resources, switch contexts faster, and do not need to deal with thread safety issues such as locks like multi-threaded programming. In the Go language, coroutine is implemented using Goroutine.
2. Create and start a coroutine
In the Go language, you can use the go statement to start a coroutine. The go statement is followed by a function call, which starts a new coroutine to execute the function.
For example:
func main() { go printHello() // 启动一个goroutine去执行printHello函数 fmt.Println("main function") } func printHello() { fmt.Println("hello goroutine") }
In the above code, we use the go statement to start a new coroutine to execute the printHello function. The printHello function will be executed in the new coroutine without blocking the main thread. After the main function is executed, the program will not end immediately because the printHello function is still running.
3. Communication of coroutines
In coroutines, since memory is shared between different coroutines, communication issues between multiple coroutines will be involved. The Go language provides channels to implement communication between coroutines. The channel-based communication method is a very efficient and safe communication method.
1. Definition and initialization of channel
In Go language, you can use the make function to create a channel. The syntax is:
channel_name := make(chan data_type)
Among them, data_type is transmitted in the channel The type of data. For example, in the following code, we create a channel that transmits int type data:
ch := make(chan int)
2. Reading and writing of channel
The channel can perform both sending and receiving operations. . Both send and receive operations are blocking.
- Sending operation: Use channel's
channel_name <- value
where value is the value to be sent. For example, in the following code, we send the value 1 to the channel named ch:
ch <- 1 // 向ch中发送数值1
- Receiving operation: Use the channel's
value := <- channel_name
Among them, value is the received value. For example, in the following code, we receive a value from the channel named ch and assign it to the variable x:
x := <- ch // 从ch中接收一个数值,并将其赋值给变量x
It should be noted that if there is no data in the channel to receive, then Receive operations automatically block until data is available to receive. Likewise, if the channel is full, the send operation will block until there is enough space to send.
4. Use multiple coroutines to communicate
The following is a simple example in which two coroutines are created, one sends data to the channel and the other receives data from the channel . Data communication in these two coroutines is completed through channels:
func main() { ch := make(chan int) go producer(ch) go consumer(ch) time.Sleep(1 * time.Second) } func producer(ch chan int) { for i := 0; i < 5; i++ { ch <- i } } func consumer(ch chan int) { for i := range ch { fmt.Println("received:", i) } }
In the above code, the producer coroutine will generate values and send data to the channel, while the consumer coroutine will receive data from the channel and Printout. In the main function, we start the producer and consumer coroutines respectively through the go statement. Due to the blocking nature of the channel, the producer and consumer coroutines can communicate safely without worrying about data inconsistency.
4. Synchronization of coroutines
In multi-coroutine programming, sometimes you need to wait for other coroutines to complete before performing certain operations. In this case, you need to use coroutine synchronization technology.
The Go language provides a Sync package, which contains some basic tools for coroutine synchronization:
- WaitGroup: Wait for a group of coroutines to complete before performing an operation.
- Mutex: Mutex lock to prevent multiple coroutines from operating on the same data at the same time.
- Cond: Condition variable, allowing a coroutine to wait for a certain condition to be satisfied before performing the next operation.
Here we take WaitGroup as an example to introduce the implementation of coroutine synchronization.
1. Definition and initialization of WaitGroup
Before using WaitGroup, you need to use the Add method to add the number of coroutines to wait for in WaitGroup. For example:
var wg sync.WaitGroup wg.Add(2)
In the above code, we added two coroutines to WaitGroup.
2. Call the Done method after the execution of the coroutine is completed
After the execution of the coroutine is completed, the Done method of WaitGroup needs to be called to indicate that the execution of a coroutine has been completed, for example:
go func() { defer wg.Done() // 协程执行完成后调用Done方法 ... }()
In the above code, we add a coroutine to the WaitGroup and call the Done method after the coroutine execution is completed.
3. Wait for all coroutines to be executed.
After adding all coroutines that need to be waited for in WaitGroup, use the Wait method to wait for all coroutines to be executed. For example:
wg.Wait() // 等待所有协程执行完成
In the above code, we use the Wait method to wait for all coroutines to complete execution. The Wait method blocks the main goroutine until all coroutines are executed.
5. Summary
This article introduces the use of coroutines in Go language, including creating and starting coroutines, coroutine communication, coroutine synchronization, etc. Coroutines are a very important feature in the Go language and play a very important role in multi-tasking and high-concurrency programming. Using coroutines can make programs run more efficiently and stably, and also facilitate developers to perform concurrent programming.
The above is the detailed content of How to use coroutines in Go?. For more information, please follow other related articles on the PHP Chinese website!
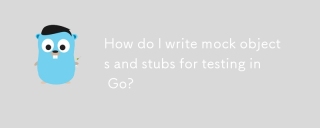
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
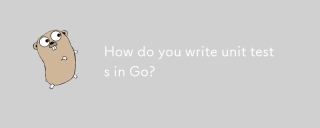
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
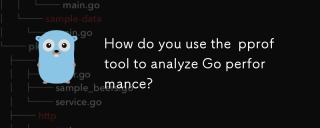
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
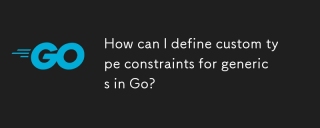
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
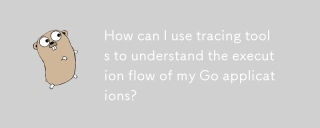
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
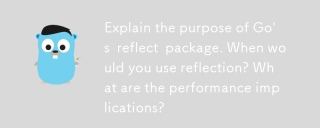
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
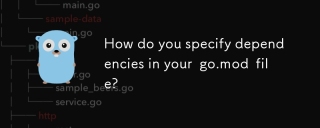
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
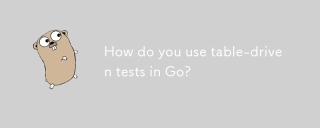
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
