How to use PHP's PDO class to optimize MySQL performance
With the rapid development of the Internet, MySQL database has become the core data storage technology for many websites, applications and even enterprises. However, with the continuous growth of data volume and the sharp increase in concurrent access, MySQL's performance problems have become increasingly prominent. PHP's PDO class is also widely used in the development and operation of MySQL because of its efficient and stable performance. In this article, we will introduce how to use the PDO class to optimize MySQL performance and improve the database's response speed and concurrent access capabilities.
1. Introduction to PDO class
PDO (PHP Data Object) class is a commonly used database operation class library in PHP. It is simple and easy to use, efficient and stable. PDO supports a variety of databases, including MySQL, Oracle, SQLite, etc. When using MySQL, PDO supports multiple connection methods, including TCP/IP, Unix domain socket and memory. The bottom layer of the PDO class uses prepared statements, which makes SQL statement execution more efficient and can also effectively prevent SQL injection attacks.
2. How to optimize MySQL performance using PDO class
- Use PDO class batch operation
In the use of MySQL, use PDO class batch operation Can significantly improve database processing performance. PDO batch operations mainly include: batch inserting data, batch updating data, and batch deleting data. Using PDO class batch operations can reduce the number of SQL statement submissions, thereby reducing the number of interactions between the database and the web server. At the same time, it can also reduce network latency, reduce the impact of locking comparison tables, and improve the concurrent processing capabilities of the database.
The specific implementation method is as follows:
a. Insert data in batches
<?php $values = array( array('id' => 1, 'username' => '张三', 'password' => 'password1'), array('id' => 2, 'username' => '李四', 'password' => 'password2'), array('id' => 3, 'username' => '王五', 'password' => 'password3'), ); $stmt = $pdo->prepare('INSERT INTO users (id, username, password) VALUES (?, ?, ?)'); foreach($values as $row){ $stmt->execute(array($row['id'], $row['username'], $row['password'])); }
b. Update data in batches
<?php $values = array( array('id' => 1, 'username' => '张三', 'password' => 'password11'), array('id' => 2, 'username' => '李四', 'password' => 'password22'), array('id' => 3, 'username' => '王五', 'password' => 'password33'), ); $sql = 'UPDATE users SET username = :username, password = :password WHERE id = :id'; $stmt = $pdo->prepare($sql); foreach($values as $row){ $stmt->execute($row); }
c. Delete data in batches
<?php $ids = array(1, 2, 3); $sql = 'DELETE FROM users WHERE id = ?'; $stmt = $pdo->prepare($sql); foreach($ids as $id){ $stmt->execute(array($id)); }
- Reasonable use of the caching mechanism of the PDO class
In the use of MySQL database, the PDO class can use the caching mechanism to improve performance and reduce the number of repeated queries. The caching mechanism of the PDO class mainly includes two aspects: query caching of the PDO class and MySQL result caching.
a. Query caching of the PDO class
The query caching of the PDO class mainly refers to using the PDOStatement object to implement query caching. PDOStatement objects are mainly used to encapsulate and execute SQL statements. By using PDOStatement objects to cache query results, you can reduce the number of interactions between PHP and the MySQL server and improve the query performance of the database.
The specific implementation method is as follows:
<?php $sql = 'SELECT * FROM users WHERE id = ?'; $stmt = $pdo->prepare($sql); $stmt->setFetchMode(PDO::FETCH_ASSOC); $stmt->bindParam(1, $id, PDO::PARAM_INT); $stmt->execute(); $result = $stmt->fetchAll(PDO::FETCH_ASSOC);
When querying, the PDOStatement object is automatically cached. When the same query is executed, the query results can be obtained directly from the cache of the PDOStatement object to avoid repeated queries. .
b. MySQL result caching
MySQL result caching refers to caching query results on the MySQL server for direct use next time, thereby reducing the number of database queries and improving performance. Usually MySQL's result caching mechanism is automatically implemented by the MySQL server, but you can also use the PDO class to manually control MySQL's result caching.
The specific implementation method is as follows:
<?php $pdo->setAttribute(PDO::MYSQL_ATTR_USE_BUFFERED_QUERY, true); $sql = 'SELECT * FROM users WHERE id = ?'; $stmt = $pdo->prepare($sql); $stmt->setFetchMode(PDO::FETCH_ASSOC); $stmt->bindParam(1, $id, PDO::PARAM_INT); $stmt->execute(); $result = $stmt->fetchAll(PDO::FETCH_ASSOC);
When querying, the PDO class uses the MYSQL_ATTR_USE_BUFFERED_QUERY attribute to enable MySQL's result cache, thereby reducing the number of queries and improving database processing performance.
3. Summary
By using the PDO class to optimize MySQL performance, the response speed and concurrent access capabilities of the MySQL database can be improved, thereby improving the processing performance of Web applications. In particular, the application of batch operations and caching mechanisms can greatly shorten query times and reduce repeated queries, reduce the interaction between the web server and the database, improve the performance of the entire application, and reduce the waste of system resources. I believe that by understanding the optimization methods introduced in this article, readers can more easily achieve efficient and stable performance optimization in MySQL applications.
The above is the detailed content of How to use PHP's PDO class to optimize MySQL performance. For more information, please follow other related articles on the PHP Chinese website!
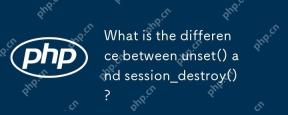
Thedifferencebetweenunset()andsession_destroy()isthatunset()clearsspecificsessionvariableswhilekeepingthesessionactive,whereassession_destroy()terminatestheentiresession.1)Useunset()toremovespecificsessionvariableswithoutaffectingthesession'soveralls
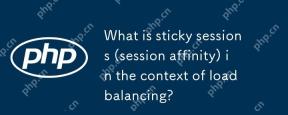
Stickysessionsensureuserrequestsareroutedtothesameserverforsessiondataconsistency.1)SessionIdentificationassignsuserstoserversusingcookiesorURLmodifications.2)ConsistentRoutingdirectssubsequentrequeststothesameserver.3)LoadBalancingdistributesnewuser
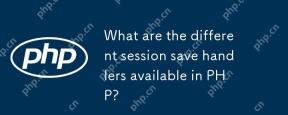
PHPoffersvarioussessionsavehandlers:1)Files:Default,simplebutmaybottleneckonhigh-trafficsites.2)Memcached:High-performance,idealforspeed-criticalapplications.3)Redis:SimilartoMemcached,withaddedpersistence.4)Databases:Offerscontrol,usefulforintegrati
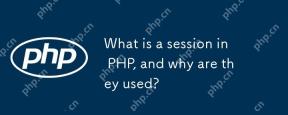
Session in PHP is a mechanism for saving user data on the server side to maintain state between multiple requests. Specifically, 1) the session is started by the session_start() function, and data is stored and read through the $_SESSION super global array; 2) the session data is stored in the server's temporary files by default, but can be optimized through database or memory storage; 3) the session can be used to realize user login status tracking and shopping cart management functions; 4) Pay attention to the secure transmission and performance optimization of the session to ensure the security and efficiency of the application.
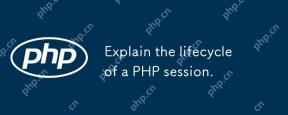
PHPsessionsstartwithsession_start(),whichgeneratesauniqueIDandcreatesaserverfile;theypersistacrossrequestsandcanbemanuallyendedwithsession_destroy().1)Sessionsbeginwhensession_start()iscalled,creatingauniqueIDandserverfile.2)Theycontinueasdataisloade
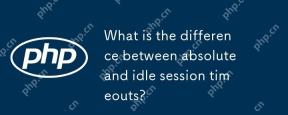
Absolute session timeout starts at the time of session creation, while an idle session timeout starts at the time of user's no operation. Absolute session timeout is suitable for scenarios where strict control of the session life cycle is required, such as financial applications; idle session timeout is suitable for applications that want users to keep their session active for a long time, such as social media.
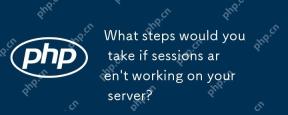
The server session failure can be solved through the following steps: 1. Check the server configuration to ensure that the session is set correctly. 2. Verify client cookies, confirm that the browser supports it and send it correctly. 3. Check session storage services, such as Redis, to ensure that they are running normally. 4. Review the application code to ensure the correct session logic. Through these steps, conversation problems can be effectively diagnosed and repaired and user experience can be improved.
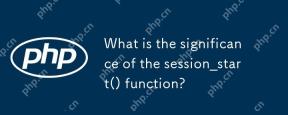
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
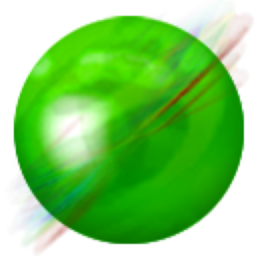
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
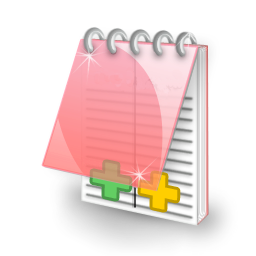
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
