Golang is a statically typed programming language launched by Google that supports concurrency and garbage collection mechanisms. It is widely favored in software development for its simplicity, efficiency, and reliability. The power of Golang lies in its interface mechanism. Through interfaces, programming techniques such as abstraction, encapsulation, and polymorphism can be realized, thereby making the code more concise and flexible. In Golang, interfaces can be freely combined and embedded to form various complex types, which greatly improves code reusability and efficiency. However, in actual development, we sometimes need to convert the interface into a byte array to facilitate transmission and storage. So, how to implement the operation of converting interfaces to bytes in Golang? This article will introduce this knowledge in detail.
- Definition of interface
In Golang, an interface is a collection of methods and does not contain fields. An interface type defines a functionality, not an implementation. Specifically, an interface type defines one or more methods, which are the only members of the interface. The zero value for an interface type is nil. Interfaces can be embedded in other interfaces to form composite interfaces.
Golang's interface is weakly typed, which means that the interface variable can store any type that conforms to the interface definition. This is Golang's polymorphism. Unlike other programming languages, polymorphism in Golang does not rely on inheritance relationships, but is implemented through interfaces. Therefore, interfaces are one of the most important mechanisms in Golang.
The interface is defined as follows:
type InterfaceName interface { Method1Name(param1 T1, param2 T2) ReturnType Method2Name(param1 T3, param2 T4) ReturnType // ... }
Among them, InterfaceName is the name of the interface, Method1Name, Method2Name, etc. are the method lists of the interface, T1, T2, T3, T4 are the types of parameters, and ReturnType Is the return value type of the method.
- Convert interface to bytes
In Golang, we can use some tools to convert interfaces to bytes. Here are a few common methods.
2.1 Using the encoding/gob package
The encoding/gob package in the Golang standard library implements the function of converting Golang data types into byte arrays. This package supports encoding various Golang data types into byte arrays and can decode them into the corresponding data types when needed. We can use the GobEncoder and GobDecoder interfaces in this package to implement custom type encoding and decoding.
The specific usage is as follows:
Define a Person structure to store personal information.
type Person struct { Name string Age int }
Create a Person variable and convert it to a byte array.
p := Person{Name: "Eric", Age: 20} // 创建一个 bytes.Buffer 用于存储编码后的字节流 buf := new(bytes.Buffer) // 创建一个 gob.Encoder,将 Person 类型编码成字节流 err := gob.NewEncoder(buf).Encode(p) if err != nil { fmt.Println(err) return }
Decode the byte array into the corresponding type.
var newP Person // 创建一个 gob.Decoder,从字节流中解码出 Person 类型 err = gob.NewDecoder(buf).Decode(&newP) if err != nil { fmt.Println(err) return } fmt.Println(newP.Name, newP.Age)
Use the encoding/gob package to convert all custom types that implement the GobEncoder and GobDecoder interfaces into byte arrays. You can also convert most of Golang's built-in types into byte arrays. However, this package does not support converting interface types to byte arrays, so it cannot be used directly to convert interfaces to byte arrays.
2.2 Using the encoding/json package
The encoding/json package in the Golang standard library is a support package for JSON encoding and decoding in Golang. It can also be used as a tool to convert interfaces into bytes. , which is very practical in some RESTful APIs.
Define an interface type MyInterface and create a structure MyStruct containing the interface.
type MyInterface interface { SayHello() string } type MyStruct struct { // MyInterface 隐式实现 MyInterface } func (s *MyStruct) SayHello() string { return "Hello" }
Encode the MyStruct type into a JSON byte array.
s := MyStruct{} // 创建一个 JSON 编码器,将 MyStruct 类型编码成 JSON 字节数组 b, err := json.Marshal(s) if err != nil { fmt.Println(err) return } fmt.Println(string(b))
Decode the corresponding type from the JSON byte array.
var newS MyStruct // 创建一个 JSON 解码器,从字节数组中解码出 MyStruct 类型 err = json.Unmarshal(b, &newS) if err != nil { fmt.Println(err) return } fmt.Println(newS.SayHello())
The encoding/json package can convert all custom types that implement the Marshaler and Unmarshaler interfaces into byte arrays, and most of Golang's built-in types can also be converted into byte arrays. Also, this package supports converting interface types to byte arrays, so it can be used to convert interfaces to byte arrays.
2.3 Using the Golang serialization framework
There are many excellent serialization frameworks in Golang, such as protobuf, msgpack, etc. These frameworks can convert Golang data types into byte arrays. These frameworks provide complete encoding and decoding interfaces, support flexible configuration and efficient serialization algorithms, which makes it very convenient to convert interfaces into bytes in Golang.
Taking protobuf as an example, first define a protobuf message type.
syntax = "proto3"; package main; message Person { string name = 1; int32 age = 2; }
Use protoc tool to generate Go code.
protoc -I=$SRC_DIR --go_out=$DST_DIR $SRC_DIR/person.proto
Using protobuf in Golang:
p := Person{Name: "Eric", Age: 20} // 将 Person 类型编码成字节流 pbBytes, err := proto.Marshal(&p) if err != nil { fmt.Println(err) return } // 将字节流解码成 Person 类型 var newP Person err = proto.Unmarshal(pbBytes, &newP) if err != nil { fmt.Println(err) return } fmt.Println(newP.Name, newP.Age)
By using the serialization framework, it is very convenient to convert various data types into byte arrays, and due to its high efficiency, it is practical Very popular in development.
Summary
This article introduces in detail the method of converting interfaces into byte arrays in Golang, including encoding/gob, encoding/json, Golang serialization framework, etc., and explains these in detail. The use and precautions of this method. Different methods are suitable for different scenarios and needs, and developers can choose the appropriate method according to the specific situation. As a statically typed programming language, Golang pays more attention to the readability and maintainability of the code while implementing interface conversion, which makes Golang one of the programming languages favored by many enterprises and projects.
The above is the detailed content of Golang interface to byte conversion. For more information, please follow other related articles on the PHP Chinese website!
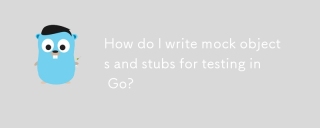
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
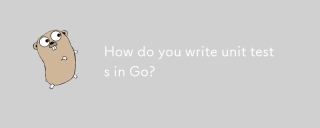
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
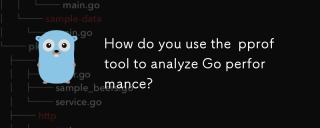
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
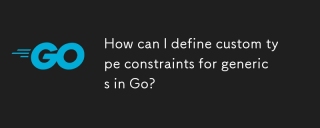
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
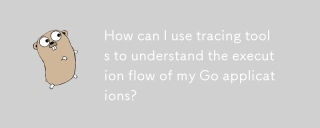
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
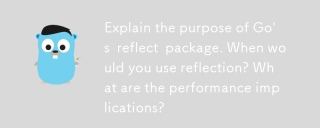
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
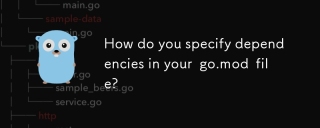
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
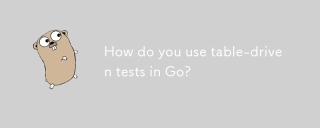
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
