With the continuous development of the Internet, grabbing red envelopes has become a very popular social activity, especially in the era of mobile Internet. Now, let’s introduce how to use golang to implement a simple red envelope grabbing system.
- Requirement Analysis
We need to implement the following functions:
- The background management terminal can create a specified number of red envelopes and set the number of red envelopes for each red envelope. Amount, total amount of red envelope and distribution time and other parameters.
- Users can grab red envelopes within the specified time. Each red envelope can only be received once. When all the red envelopes are collected, the red envelope grabbing activity ends.
- Technology Selection
In order to achieve the above requirements, we need to choose the appropriate technology, as follows:
- Web Framework : Since golang itself does not have its own web framework, we can choose third-party frameworks such as martini and gin.
- Database: We can choose MySQL, PostgreSQL, MongoDB and other databases.
- Cache: Since the operation of grabbing red envelopes requires high concurrency support, we need to use caches such as Redis to improve the concurrency capability of the system.
- Database design
We need to create the following two tables:
- Red envelope table (hb_info): used to store red envelopes Basic information, including red envelope ID, total red envelope amount, distribution time, etc.
- Red envelope grabbing record table (hb_detail): used to record the information of each user grabbing red envelopes, including user ID, amount grabbed and other information.
- System architecture design
We can divide the entire system into the following modules:
- Backend management module: main Responsible for creating red envelopes, setting parameters and other operations.
- Red envelope grabbing module: Mainly responsible for processing users’ requests for red envelope grabbing and completing the logical processing of red envelope grabbing.
- Database module: Mainly responsible for interacting with the database and storing red envelopes and red envelope grabbing records in the database.
- Cache module: Mainly responsible for storing red envelopes and red envelope grabbing records in the cache to improve the concurrency capability of the system.
- Technical Implementation
The following are the detailed steps to implement the red envelope grabbing system in golang:
5.1 Create red envelope
Implementation Process:
- Users create red envelopes through the background management page and set parameters such as the total amount of red envelopes, the number of red envelopes, and the type of red envelopes.
- The system generates a batch of red envelope codes, stores the red envelope codes and amounts in the Redis cache, and stores the red envelope information in the MySQL database.
- The red envelope code generation method can use UUID, timestamp, etc. to prevent code duplication. The code length can be customized according to business needs.
Code implementation:
func generateRedPackage(totalAmount float64, num int32, redPackageType int32) ([]*RedPackage, error) { // 验证红包金额和个数是否合法 if totalAmount <= 0 || num <= 0 { return nil, errors.New("红包金额或个数不能小于等于0") } // 计算平均值 avgAmount := totalAmount / float64(num) // 生成红包码 redPackageCodes := make([]string, num) for i := 0; i < len(redPackageCodes); i++ { code := generateRedPackageCode() redPackageCodes[i] = code } // 分配红包金额 redPackages := make([]*RedPackage, num) for i := 0; i < len(redPackages); i++ { redPackages[i] = &RedPackage{ Code: redPackageCodes[i], Amount: avgAmount, RedPackageType: redPackageType, } totalAmount -= avgAmount if i == len(redPackages) - 1 { redPackages[i].Amount += totalAmount break } redPackages[i].Amount += avgAmount } // 存入数据库和 Redis 缓存中 return redPackages, nil }
5.2 Grabbing red envelopes
Implementation process:
- The user initiates a request to grab red envelopes within the specified time, The system obtains a red envelope code from the Redis cache.
- The system verifies whether the current user has grabbed the red envelope. If not, it will proceed to grab the red envelope.
- The red envelope grabbing operation includes taking out the red envelope amount from the Redis cache, generating a red envelope grabbing record, and depositing the amount into the user account.
Code implementation:
func getRedPackage(code string, userId int64) (*RedPackage, error) { // 先从缓存中获取该红包的金额 rc := redisMgr.RedisClient() redPackageAmount, err := rc.RPop(code).Result() if err != nil { return nil, errors.New("红包已经被抢完了") } // 判断用户是否已经抢到过该红包 key := fmt.Sprintf("%s:%d", code, userId) result, err := rc.Exists(key).Result() if err != nil || result == 1 { return nil, errors.New("您已经抢过该红包了") } // 生成抢红包记录 record := &RedPackageRecord{ RedPackageCode: code, UserId: userId, Amount: redPackageAmount, CreateTime: time.Now(), } // 将抢红包记录和金额存入 MySQL 数据库中 err = dbMgr.SaveRedPackageRecord(record) if err != nil { return nil, err } // 将金额存入用户账户中 err = dbMgr.UpdateUserAmount(userId, redPackageAmount) if err != nil { return nil, err } // 返回抢到的红包金额 redPackage := &RedPackage{ Code: code, Amount: redPackageAmount, } return redPackage, nil }
- Summary
Through the above steps, we have completed the implementation of a simple red envelope grabbing system. In actual development, issues such as system security, stability, and performance also need to be considered, and more detailed testing and performance optimization are required to ensure that the system can meet user needs during operation.
The above is the detailed content of Golang realizes grabbing red envelopes. For more information, please follow other related articles on the PHP Chinese website!
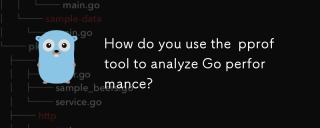
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
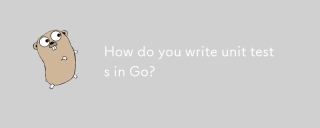
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
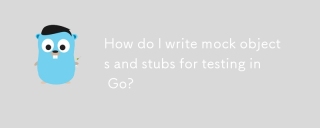
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
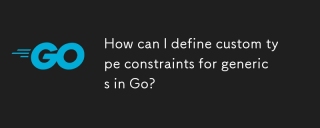
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
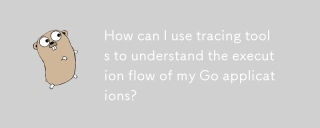
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
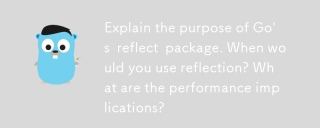
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
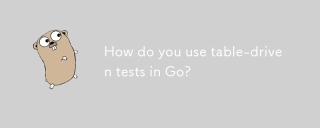
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
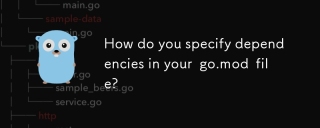
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
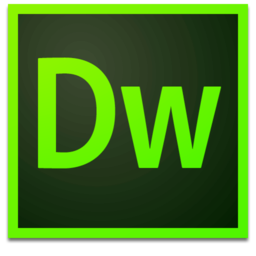
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
