Golang is a popular programming language that often involves file operations in development, including file reading, writing, deletion, etc. When performing file operations, the correctness of the file path is crucial, but what should you do when you encounter an incorrect file path?
First of all, we need to understand how file paths are represented in Golang. Different operating systems may represent file paths differently, so we need to make corresponding adjustments to the operating system. In the Windows operating system, the file path is represented by the backslash "", while in the Linux operating system, the file path is represented by the slash "/". Therefore, when writing Golang code, we need to write the code according to different operating systems to ensure the correctness of the code.
In Golang, we can use the Join
function in the os
package to splice file paths. For example, if we want to splice file paths in Windows, we can use the following code:
import "path/filepath" import "os" func main() { dir := "C:\Users\abc\Desktop" filename := "example.txt" filepath := filepath.Join(dir, filename) f, err := os.Open(filepath) if err != nil { panic(err) } }
In the above code, we first define a folder path dir
and a file name filename
, and then use the filepath.Join
function to splice them together to get the final file path filepath
. Next, we open the file through the os.Open
function and perform operations.
In addition to using the Join
function, we can also manually splice file paths. For example, in Linux, we can use the following code to manually splice file paths:
dir := "/home/abc" filename := "example.txt" filepath := dir + "/" + filename
However, it should be noted that when manually splicing file paths, we need to determine which delimiter to use according to the operating system.
When encountering an incorrect file path in Golang, we can use the following methods to troubleshoot and solve:
- Print the file path: add a print statement to the code , print out the file path for easy troubleshooting.
- Check whether the file path exists: Use the
os.Stat
function to check whether the file path exists. - Check file permissions: When operating a file, you need to ensure that you have sufficient permissions on the file.
- Use relative paths: When performing file operations, using relative paths can avoid path errors.
In summary, the correctness of the file path is very important for the normal operation of the Golang program. When writing Golang code, we need to adjust the path representation method according to the operating system, and make timely troubleshooting and adjustments when path errors occur.
The above is the detailed content of The golang file path is incorrect. For more information, please follow other related articles on the PHP Chinese website!
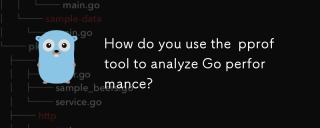
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
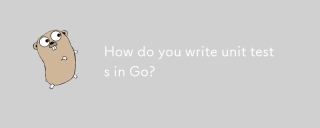
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
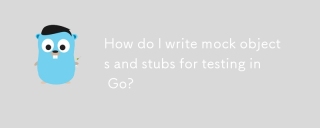
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
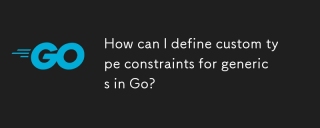
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
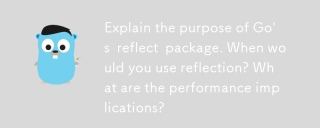
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
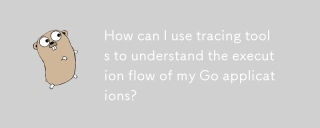
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
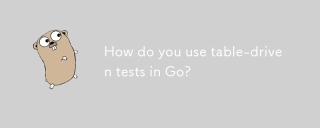
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
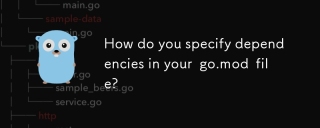
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
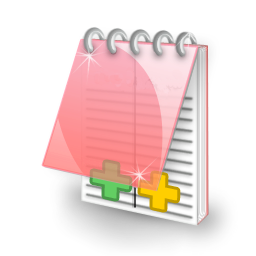
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
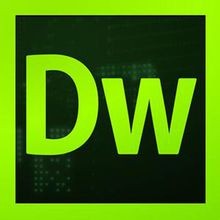
Dreamweaver CS6
Visual web development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
