Go is a statically typed programming language that supports various types including commonly used basic types, structures, arrays and slices, mappings, interfaces, and functions. The following are some commonly used Go language types:
- Basic types
Go language supports standard basic types, such as Boolean, integer, floating point, and string and character type.
- Boolean type: bool type, only Boolean values true and false.
- Integer types: int, int8, int16, int32, int64, uint, uint8, uint16, uint32 and uint64, representing signed integers and unsigned integers respectively, with sizes of 8, 16, 32 and 64 bits respectively. .
- Floating point types: float32 and float64, representing 32-bit and 64-bit floating point numbers respectively.
- Character type: rune type, which is an encoding of Unicode characters.
- Structure
The structure in Go language is a user-defined composite type that can contain multiple fields of different types. Structures can be used to represent some complex data types. Fields in a structure can be of any type, including other structure types.
For example:
type Person struct { Name string Age int Height float32 }
- Arrays and slices
Arrays are also a basic type in the Go language. Arrays have a fixed size, and the length of the array needs to be specified when defining. A slice is a reference to an array, dynamically allocated space, and can be increased or decreased at any time. You can use the built-in function len() to get the length of a slice.
For example:
var array [3]int // 定义一个长度为3的数组 slice := []int{1, 2, 3, 4, 5} // 定义一个切片
- Map
A map is a data structure of key-value pairs, similar to a dictionary or hash table in other languages . In Go language, mapping is represented using the built-in map type. You can use the make() function to create a map, and then use the [] operator to access elements in the map.
For example:
scores := make(map[string]int) // 创建一个名为scores的映射 scores["Alice"] = 85 // 添加一个键值对
- Interface
Interface is an abstract data type that can define a set of methods without implementing specific code. In a type that implements an interface, all methods defined in the interface need to be implemented. Interfaces can make code more flexible, extensible and maintainable.
For example:
type Shape interface { Area() float64 Perimeter() float64 } type Rectangle struct { Width float64 Height float64 } func (r Rectangle) Area() float64 { return r.Width * r.Height } func (r Rectangle) Perimeter() float64 { return 2 * (r.Width + r.Height) }
- Function type
Functions are first-class citizens in the Go language. They can be passed, assigned, and return. In the Go language, a function is also a type, and function types can be defined as parameters or return values.
For example:
type Operation func(int, int) int func Add(a, b int) int { return a + b } func Sub(a, b int) int { return a - b } func calc(op Operation, a, b int) int { return op(a, b) } sum := calc(Add, 10, 20) // 调用calc函数,传入Add函数作为参数 diff := calc(Sub, 30, 15) // 调用calc函数,传入Sub函数作为参数
The above are some commonly used Go language types. Go language also supports other types, such as channels, pointers, etc. Mastering these types is very important for writing high-quality, readable Go code.
The above is the detailed content of What are the golang types?. For more information, please follow other related articles on the PHP Chinese website!
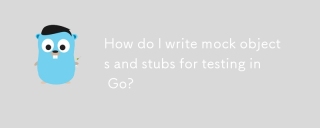
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
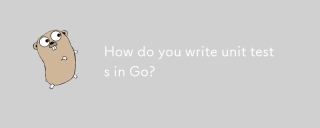
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
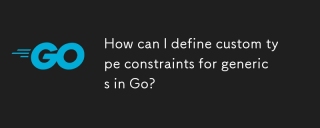
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
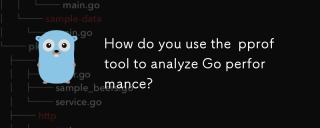
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
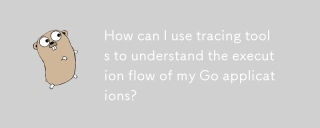
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
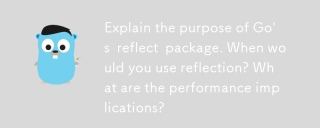
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
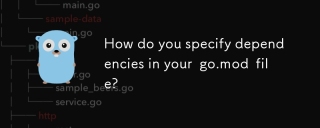
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
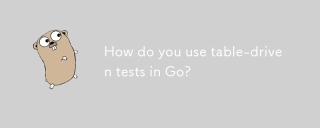
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version
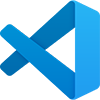
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
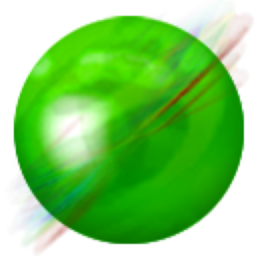
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
